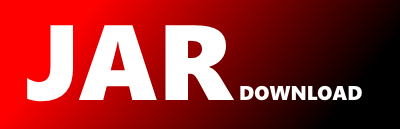
com.mark59.core.utils.Mark59Constants Maven / Gradle / Ivy
Show all versions of mark59-core Show documentation
/*
* Copyright 2019 Mark59.com
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.mark59.core.utils;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* @author Michael Cohen
* @author Philip Webb
* Written: Australian Winter 2019
*/
public class Mark59Constants {
/**
* current Mark59 version
*/
public static final String MARK59_VERSION = "6.2";
/**
* TRUE
*/
public static final String TRUE = "TRUE";
/**
* FALSE
*/
public static final String FALSE = "FALSE";
/**
* the default driver (used if DRIVER not specified as a script argument) - CHROME
*/
public static final String DEFAULT_DRIVER = "CHROME";
/**
* CHROME
*/
public static final String CHROME = "CHROME";
/**
* FIREFOX
*/
public static final String FIREFOX= "FIREFOX";
/**
* Browser default width
*/
public static final int DEFAULT_BROWSER_WIDTH = 1920;
/**
* Browser default height
*/
public static final int DEFAULT_BROWSER_HEIGHT = 1080;
/**
* Browser default dimensions (width by height)
*/
public static final String DEFAULT_BROWSER_DIMENSIONS ="1920,1080";
/**
* Value for {@link PropertiesKeys#MARK59_PROP_LOG_DIRECTORY_SUFFIX }
* date will suffix directory with current date
*/
public static final String DATE ="date";
/**
* Value for {@link PropertiesKeys#MARK59_PROP_LOG_DIRECTORY_SUFFIX }
* suffix directory with date and time
*/
public static final String DATE_TIME ="datetime";
/**
* REFERENCE
*/
public static final String REFERENCE = "__Mark59.com____";
/**
* H2 (file based) database
*/
public static final String H2 = "h2";
/**
* MySQL database
*/
public static final String MYSQL = "mysql";
/**
* Postgres database
*/
public static final String PG = "pg";
/**
* H2 'in memory' database
*/
public static final String H2MEM = "h2mem";
/**
* a H2 database acting as a 'client' server (useful in Docker for communication a H2 database over Docker instances)
*/
public static final String H2TCPCLIENT = "h2tcpclient";
// log name format options:
/**
* THREADNAME log name format option
*/
public static final String THREAD_NAME = "THREADNAME";
/**
* THREADGROUP log name format option
*/
public static final String THREAD_GROUP = "THREADGROUP";
/**
* SAMPLER log name format option
*/
public static final String SAMPLER = "SAMPLER";
/**
* LABEL log name format option (equates to a Mark59 Transaction)
*/
public static final String LABEL = "LABEL";
/**
* Count used in log names (ensures uniqueness, and indicates order the log occurred in the run)
*/
public static final String LOG_COUNTER = "LOG_COUNTER";
/**
* The (ordered) allowable value(s) used to define the format of log names in a Mark59 screenshot directory.
* Done via setting the via setting the values in a comma delimited list in property
* {@link PropertiesKeys#MARK59_PROP_LOGNAME_FORMAT}. Allowed value options are:
* ThreadName, ThreadGroup, Sampler, Label
* (case insensitive).
*
A log counter is always included in log names (ie, the LOG_COUNTER is not an option)
*/
public static final List LOGNAME_FORMAT_OPTIONS = Arrays.asList(THREAD_NAME, THREAD_GROUP, SAMPLER, LABEL);
/**
* Defines an enumeration of values used in the Mark59 framework that are used in to populate the JMeter
* results file data type (the 'dt' column in a csv formatted file)
*
* The current list of types, and their corresponding text values (as would appear in a JMeter csv Results file) are:
*
* __________________________________________________________________________________________________
* Mark59 JMeterFileDatatypes
enumeration
--> JMeter file 'dt' value
* CPU_UTIL --> CPU_UTIL
* MEMORY --> MEMORY
* DATAPOINT --> DATAPOINT
* CDP --> CDP to tag a DevTools (CDP) transaction
* TRANSACTION --> '' (blank) a standard transaction
* PARENT --> PARENT parent transaction (to sub-transactions)
*
*
* Note that 'PARENT' is intended for internal use only.
*/
public enum JMeterFileDatatypes {
/** DATAPOINT */
DATAPOINT("DATAPOINT", true),
/** CPU_UTIL */
CPU_UTIL("CPU_UTIL", true),
/** MEMORY */
MEMORY("MEMORY", true),
/** TRANSACTION */
TRANSACTION("", false),
/** CDP */
CDP("CDP", false),
/** PARENT */
PARENT("PARENT", false);
private final String datatypeText;
private final boolean metricDataType;
JMeterFileDatatypes(String datatypeText, boolean metricDataType) {
this.datatypeText = datatypeText;
this.metricDataType = metricDataType;
}
/**
* @return value of text as used in the JMeter file
*/
public String getDatatypeText() {
return datatypeText;
}
/**
* @return true if it is a 'metric' type
*/
public boolean isMetricDataType() {
return this.metricDataType;
}
/**
* @return a string list of all transaction data types as used in the JMeter file
*/
public static List listOfJMeterFileDatatypes() {
List listOfJMeterFileDatatypes = new ArrayList<>();
for (JMeterFileDatatypes jMeterFileDatatype : JMeterFileDatatypes.values()) {
listOfJMeterFileDatatypes.add(jMeterFileDatatype.name());
}
return listOfJMeterFileDatatypes;
}
/**
* @return a string list of metrics data types as used in the JMeter file
*/
public static List listOfMetricJMeterFileDatatypes() {
List listOfMetricJMeterFileDatatypes = new ArrayList<>();
for (Mark59Constants.JMeterFileDatatypes jMeterFileDatatype : JMeterFileDatatypes.values()) {
if (jMeterFileDatatype.isMetricDataType()) {
listOfMetricJMeterFileDatatypes.add(jMeterFileDatatype.name());
}
}
return listOfMetricJMeterFileDatatypes;
}
}
/**
* Defines an enumeration for transaction data-type values used in the Mark59 framework that are stored
* in the database (TXN_TYPE on the transaction table )
*
* Enum values are DATAPOINT, CPU_UTIL, MEMORY, TRANSACTION
*/
public enum DatabaseTxnTypes {
/** DATAPOINT */
DATAPOINT(true),
/** CPU_UTIL */
CPU_UTIL(true),
/** MEMORY */
MEMORY(true),
/** TRANSACTION */
TRANSACTION(false);
private final boolean metricTxnType;
DatabaseTxnTypes(boolean metricTxnType) {
this.metricTxnType = metricTxnType;
}
/**
* @return true if it is a 'metric' transaction type
*/
public boolean isMetricTxnType() {
return this.metricTxnType;
}
/**
* @return string list of all transaction types as used in the database
*/
public static List listOfDatabaseTxnTypes(){
List listOfDatabaseTxnTypes = new ArrayList<>();
for (DatabaseTxnTypes databaseTxnType : DatabaseTxnTypes.values()) {
listOfDatabaseTxnTypes.add(databaseTxnType.name());
}
return listOfDatabaseTxnTypes;
}
/**
* @return a string list of metrics types as used in the database
*/
public static List listOfMetricDatabaseTxnTypes() {
List listOfMetricDatabaseTxnTypes = new ArrayList<>();
for (DatabaseTxnTypes databaseTxntypes : DatabaseTxnTypes.values()) {
if (databaseTxntypes.isMetricTxnType()) {
listOfMetricDatabaseTxnTypes.add(databaseTxntypes.name());
}
}
return listOfMetricDatabaseTxnTypes;
}
}
/**
* Defines enumerated values for the operating system.
* Enum values are WINDOWS, LINUX, MAC
*/
public enum OS {
/** WINDOWS */
WINDOWS("WINDOWS"),
/** LINUX */
LINUX("LINUX"),
/** MAC */
MAC("MAC");
private final String osName;
/** @param osName operating system name */
OS(String osName) { this.osName = osName;}
/** @return a string holding the operating system name */
public String getOsName() {return osName;}
}
private Mark59Constants() {
}
}