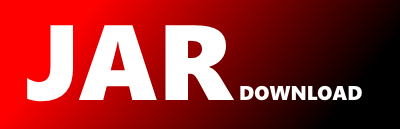
com.marklogic.hub.dataservices.ConceptService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marklogic-data-hub Show documentation
Show all versions of marklogic-data-hub Show documentation
Library for Creating an Operational Data Hub on MarkLogic
package com.marklogic.hub.dataservices;
// IMPORTANT: Do not edit. This file is generated.
import com.marklogic.client.DatabaseClient;
import com.marklogic.client.impl.BaseProxy;
import com.marklogic.client.io.Format;
import com.marklogic.client.io.marker.JSONWriteHandle;
/**
* Provides a set of operations on the database server
*/
public interface ConceptService {
/**
* Creates a ConceptService object for executing operations on the database server.
*
* The DatabaseClientFactory class can create the DatabaseClient parameter. A single
* client object can be used for any number of requests and in multiple threads.
*
* @param db provides a client for communicating with the database server
* @return an object for executing database operations
*/
static ConceptService on(DatabaseClient db) {
return on(db, null);
}
/**
* Creates a ConceptService object for executing operations on the database server.
*
* The DatabaseClientFactory class can create the DatabaseClient parameter. A single
* client object can be used for any number of requests and in multiple threads.
*
* The service declaration uses a custom implementation of the same service instead
* of the default implementation of the service by specifying an endpoint directory
* in the modules database with the implementation. A service.json file with the
* declaration can be read with FileHandle or a string serialization of the JSON
* declaration with StringHandle.
*
* @param db provides a client for communicating with the database server
* @param serviceDeclaration substitutes a custom implementation of the service
* @return an object for executing database operations
*/
static ConceptService on(DatabaseClient db, JSONWriteHandle serviceDeclaration) {
final class ConceptServiceImpl implements ConceptService {
private final DatabaseClient dbClient;
private final BaseProxy baseProxy;
private final BaseProxy.DBFunctionRequest req_updateDraftModelInfo;
private final BaseProxy.DBFunctionRequest req_deleteDraftModel;
private final BaseProxy.DBFunctionRequest req_saveConceptModels;
private final BaseProxy.DBFunctionRequest req_createDraftModel;
private final BaseProxy.DBFunctionRequest req_getConceptReferences;
ConceptServiceImpl(DatabaseClient dbClient, JSONWriteHandle servDecl) {
this.dbClient = dbClient;
this.baseProxy = new BaseProxy("/data-hub/data-services/concept/", servDecl);
this.req_updateDraftModelInfo = this.baseProxy.request(
"updateDraftConceptModelInfo.mjs", BaseProxy.ParameterValuesKind.MULTIPLE_MIXED);
this.req_deleteDraftModel = this.baseProxy.request(
"deleteDraftConceptModel.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_saveConceptModels = this.baseProxy.request(
"saveConceptModels.mjs", BaseProxy.ParameterValuesKind.SINGLE_NODE);
this.req_createDraftModel = this.baseProxy.request(
"createDraftConceptModel.mjs", BaseProxy.ParameterValuesKind.SINGLE_NODE);
this.req_getConceptReferences = this.baseProxy.request(
"getConceptReferences.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
}
@Override
public com.fasterxml.jackson.databind.JsonNode updateDraftModelInfo(String name, com.fasterxml.jackson.databind.JsonNode input) {
return updateDraftModelInfo(
this.req_updateDraftModelInfo.on(this.dbClient), name, input
);
}
private com.fasterxml.jackson.databind.JsonNode updateDraftModelInfo(BaseProxy.DBFunctionRequest request, String name, com.fasterxml.jackson.databind.JsonNode input) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("name", false, BaseProxy.StringType.fromString(name)),
BaseProxy.documentParam("input", false, BaseProxy.JsonDocumentType.fromJsonNode(input))
).responseSingle(false, Format.JSON)
);
}
@Override
public void deleteDraftModel(String conceptName) {
deleteDraftModel(
this.req_deleteDraftModel.on(this.dbClient), conceptName
);
}
private void deleteDraftModel(BaseProxy.DBFunctionRequest request, String conceptName) {
request
.withParams(
BaseProxy.atomicParam("conceptName", false, BaseProxy.StringType.fromString(conceptName))
).responseNone();
}
@Override
public void saveConceptModels(com.fasterxml.jackson.databind.JsonNode models) {
saveConceptModels(
this.req_saveConceptModels.on(this.dbClient), models
);
}
private void saveConceptModels(BaseProxy.DBFunctionRequest request, com.fasterxml.jackson.databind.JsonNode models) {
request
.withParams(
BaseProxy.documentParam("models", false, BaseProxy.JsonDocumentType.fromJsonNode(models))
).responseNone();
}
@Override
public com.fasterxml.jackson.databind.JsonNode createDraftModel(com.fasterxml.jackson.databind.JsonNode input) {
return createDraftModel(
this.req_createDraftModel.on(this.dbClient), input
);
}
private com.fasterxml.jackson.databind.JsonNode createDraftModel(BaseProxy.DBFunctionRequest request, com.fasterxml.jackson.databind.JsonNode input) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.documentParam("input", false, BaseProxy.JsonDocumentType.fromJsonNode(input))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getConceptReferences(String conceptName) {
return getConceptReferences(
this.req_getConceptReferences.on(this.dbClient), conceptName
);
}
private com.fasterxml.jackson.databind.JsonNode getConceptReferences(BaseProxy.DBFunctionRequest request, String conceptName) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("conceptName", false, BaseProxy.StringType.fromString(conceptName))
).responseSingle(false, Format.JSON)
);
}
}
return new ConceptServiceImpl(db, serviceDeclaration);
}
/**
* Update the description of an existing concept class. Concept name cannot yet be edited because doing so would break existing mapping and mastering configurations. Changes are saved to the concept class draft collection.
*
* @param name The name of the model
* @param input provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode updateDraftModelInfo(String name, com.fasterxml.jackson.databind.JsonNode input);
/**
* Mark a draft concept class to be deleted
*
* @param conceptName The name of the concept in the model
*
*/
void deleteDraftModel(String conceptName);
/**
* Save an array of concept models
*
* @param models The array of concept models
*
*/
void saveConceptModels(com.fasterxml.jackson.databind.JsonNode models);
/**
* Create a new draft model, resulting in a new Concept descriptor.
*
* @param input provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode createDraftModel(com.fasterxml.jackson.databind.JsonNode input);
/**
* Returns a json containing the names of the entities that reference the given concept class.
*
* @param conceptName provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode getConceptReferences(String conceptName);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy