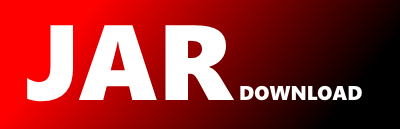
com.marklogic.hub.dataservices.EntitySearchService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marklogic-data-hub Show documentation
Show all versions of marklogic-data-hub Show documentation
Library for Creating an Operational Data Hub on MarkLogic
package com.marklogic.hub.dataservices;
// IMPORTANT: Do not edit. This file is generated.
import com.marklogic.client.DatabaseClient;
import com.marklogic.client.impl.BaseProxy;
import com.marklogic.client.io.Format;
import com.marklogic.client.io.marker.JSONWriteHandle;
import java.util.stream.Stream;
/**
* Provides a set of operations on the database server
*/
public interface EntitySearchService {
/**
* Creates a EntitySearchService object for executing operations on the database server.
*
* The DatabaseClientFactory class can create the DatabaseClient parameter. A single
* client object can be used for any number of requests and in multiple threads.
*
* @param db provides a client for communicating with the database server
* @return an object for executing database operations
*/
static EntitySearchService on(DatabaseClient db) {
return on(db, null);
}
/**
* Creates a EntitySearchService object for executing operations on the database server.
*
* The DatabaseClientFactory class can create the DatabaseClient parameter. A single
* client object can be used for any number of requests and in multiple threads.
*
* The service declaration uses a custom implementation of the same service instead
* of the default implementation of the service by specifying an endpoint directory
* in the modules database with the implementation. A service.json file with the
* declaration can be read with FileHandle or a string serialization of the JSON
* declaration with StringHandle.
*
* @param db provides a client for communicating with the database server
* @param serviceDeclaration substitutes a custom implementation of the service
* @return an object for executing database operations
*/
static EntitySearchService on(DatabaseClient db, JSONWriteHandle serviceDeclaration) {
final class EntitySearchServiceImpl implements EntitySearchService {
private final DatabaseClient dbClient;
private final BaseProxy baseProxy;
private final BaseProxy.DBFunctionRequest req_getMinAndMaxPropertyValues;
private final BaseProxy.DBFunctionRequest req_getSavedQuery;
private final BaseProxy.DBFunctionRequest req_deleteSavedQuery;
private final BaseProxy.DBFunctionRequest req_saveSavedQuery;
private final BaseProxy.DBFunctionRequest req_getSavedQueries;
private final BaseProxy.DBFunctionRequest req_exportSearchAsCSV;
private final BaseProxy.DBFunctionRequest req_getSemanticConceptInfo;
private final BaseProxy.DBFunctionRequest req_getModelRelationships;
private final BaseProxy.DBFunctionRequest req_getRecord;
private final BaseProxy.DBFunctionRequest req_getMatchingPropertyValues;
EntitySearchServiceImpl(DatabaseClient dbClient, JSONWriteHandle servDecl) {
this.dbClient = dbClient;
this.baseProxy = new BaseProxy("/data-hub/data-services/entitySearch/", servDecl);
this.req_getMinAndMaxPropertyValues = this.baseProxy.request(
"getMinAndMaxPropertyValues.mjs", BaseProxy.ParameterValuesKind.SINGLE_NODE);
this.req_getSavedQuery = this.baseProxy.request(
"getSavedQuery.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_deleteSavedQuery = this.baseProxy.request(
"deleteSavedQuery.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_saveSavedQuery = this.baseProxy.request(
"saveSavedQuery.mjs", BaseProxy.ParameterValuesKind.SINGLE_NODE);
this.req_getSavedQueries = this.baseProxy.request(
"getSavedQueries.mjs", BaseProxy.ParameterValuesKind.NONE);
this.req_exportSearchAsCSV = this.baseProxy.request(
"exportSearchAsCSV.mjs", BaseProxy.ParameterValuesKind.MULTIPLE_MIXED);
this.req_getSemanticConceptInfo = this.baseProxy.request(
"getSemanticConceptInfo.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_getModelRelationships = this.baseProxy.request(
"getModelRelationships.mjs", BaseProxy.ParameterValuesKind.NONE);
this.req_getRecord = this.baseProxy.request(
"getRecord.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_getMatchingPropertyValues = this.baseProxy.request(
"getMatchingPropertyValues.mjs", BaseProxy.ParameterValuesKind.SINGLE_NODE);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getMinAndMaxPropertyValues(com.fasterxml.jackson.databind.JsonNode facetRangeSearchQuery) {
return getMinAndMaxPropertyValues(
this.req_getMinAndMaxPropertyValues.on(this.dbClient), facetRangeSearchQuery
);
}
private com.fasterxml.jackson.databind.JsonNode getMinAndMaxPropertyValues(BaseProxy.DBFunctionRequest request, com.fasterxml.jackson.databind.JsonNode facetRangeSearchQuery) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.documentParam("facetRangeSearchQuery", false, BaseProxy.JsonDocumentType.fromJsonNode(facetRangeSearchQuery))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getSavedQuery(String id) {
return getSavedQuery(
this.req_getSavedQuery.on(this.dbClient), id
);
}
private com.fasterxml.jackson.databind.JsonNode getSavedQuery(BaseProxy.DBFunctionRequest request, String id) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("id", false, BaseProxy.StringType.fromString(id))
).responseSingle(false, Format.JSON)
);
}
@Override
public void deleteSavedQuery(String id) {
deleteSavedQuery(
this.req_deleteSavedQuery.on(this.dbClient), id
);
}
private void deleteSavedQuery(BaseProxy.DBFunctionRequest request, String id) {
request
.withParams(
BaseProxy.atomicParam("id", false, BaseProxy.StringType.fromString(id))
).responseNone();
}
@Override
public com.fasterxml.jackson.databind.JsonNode saveSavedQuery(com.fasterxml.jackson.databind.JsonNode saveQuery) {
return saveSavedQuery(
this.req_saveSavedQuery.on(this.dbClient), saveQuery
);
}
private com.fasterxml.jackson.databind.JsonNode saveSavedQuery(BaseProxy.DBFunctionRequest request, com.fasterxml.jackson.databind.JsonNode saveQuery) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.documentParam("saveQuery", false, BaseProxy.JsonDocumentType.fromJsonNode(saveQuery))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getSavedQueries() {
return getSavedQueries(
this.req_getSavedQueries.on(this.dbClient)
);
}
private com.fasterxml.jackson.databind.JsonNode getSavedQueries(BaseProxy.DBFunctionRequest request) {
return BaseProxy.JsonDocumentType.toJsonNode(
request.responseSingle(false, Format.JSON)
);
}
@Override
public java.io.Reader exportSearchAsCSV(String structuredQuery, String searchText, String queryOptions, String schemaName, String viewName, Long limit, com.fasterxml.jackson.databind.node.ArrayNode sortOrder, Stream columns) {
return exportSearchAsCSV(
this.req_exportSearchAsCSV.on(this.dbClient), structuredQuery, searchText, queryOptions, schemaName, viewName, limit, sortOrder, columns
);
}
private java.io.Reader exportSearchAsCSV(BaseProxy.DBFunctionRequest request, String structuredQuery, String searchText, String queryOptions, String schemaName, String viewName, Long limit, com.fasterxml.jackson.databind.node.ArrayNode sortOrder, Stream columns) {
return BaseProxy.TextDocumentType.toReader(
request
.withParams(
BaseProxy.atomicParam("structuredQuery", false, BaseProxy.StringType.fromString(structuredQuery)),
BaseProxy.atomicParam("searchText", true, BaseProxy.StringType.fromString(searchText)),
BaseProxy.atomicParam("queryOptions", false, BaseProxy.StringType.fromString(queryOptions)),
BaseProxy.atomicParam("schemaName", false, BaseProxy.StringType.fromString(schemaName)),
BaseProxy.atomicParam("viewName", false, BaseProxy.StringType.fromString(viewName)),
BaseProxy.atomicParam("limit", true, BaseProxy.LongType.fromLong(limit)),
BaseProxy.documentParam("sortOrder", false, BaseProxy.ArrayType.fromArrayNode(sortOrder)),
BaseProxy.atomicParam("columns", false, BaseProxy.StringType.fromString(columns))
).responseSingle(false, Format.TEXT)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getSemanticConceptInfo(String semanticConceptIRI) {
return getSemanticConceptInfo(
this.req_getSemanticConceptInfo.on(this.dbClient), semanticConceptIRI
);
}
private com.fasterxml.jackson.databind.JsonNode getSemanticConceptInfo(BaseProxy.DBFunctionRequest request, String semanticConceptIRI) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("semanticConceptIRI", false, BaseProxy.StringType.fromString(semanticConceptIRI))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getModelRelationships() {
return getModelRelationships(
this.req_getModelRelationships.on(this.dbClient)
);
}
private com.fasterxml.jackson.databind.JsonNode getModelRelationships(BaseProxy.DBFunctionRequest request) {
return BaseProxy.JsonDocumentType.toJsonNode(
request.responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getRecord(String docUri) {
return getRecord(
this.req_getRecord.on(this.dbClient), docUri
);
}
private com.fasterxml.jackson.databind.JsonNode getRecord(BaseProxy.DBFunctionRequest request, String docUri) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("docUri", false, BaseProxy.StringType.fromString(docUri))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getMatchingPropertyValues(com.fasterxml.jackson.databind.JsonNode facetValuesSearchQuery) {
return getMatchingPropertyValues(
this.req_getMatchingPropertyValues.on(this.dbClient), facetValuesSearchQuery
);
}
private com.fasterxml.jackson.databind.JsonNode getMatchingPropertyValues(BaseProxy.DBFunctionRequest request, com.fasterxml.jackson.databind.JsonNode facetValuesSearchQuery) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.documentParam("facetValuesSearchQuery", false, BaseProxy.JsonDocumentType.fromJsonNode(facetValuesSearchQuery))
).responseSingle(false, Format.JSON)
);
}
}
return new EntitySearchServiceImpl(db, serviceDeclaration);
}
/**
* Invokes the getMinAndMaxPropertyValues operation on the database server
*
* @param facetRangeSearchQuery provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode getMinAndMaxPropertyValues(com.fasterxml.jackson.databind.JsonNode facetRangeSearchQuery);
/**
* Invokes the getSavedQuery operation on the database server
*
* @param id provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode getSavedQuery(String id);
/**
* Invokes the deleteSavedQuery operation on the database server
*
* @param id provides input
*
*/
void deleteSavedQuery(String id);
/**
* Invokes the saveSavedQuery operation on the database server
*
* @param saveQuery provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode saveSavedQuery(com.fasterxml.jackson.databind.JsonNode saveQuery);
/**
* Invokes the getSavedQueries operation on the database server
*
*
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode getSavedQueries();
/**
* Invokes the exportSearchAsCSV operation on the database server
*
* @param structuredQuery provides input
* @param searchText provides input
* @param queryOptions provides input
* @param schemaName provides input
* @param viewName provides input
* @param limit provides input
* @param sortOrder provides input
* @param columns provides input
* @return as output
*/
java.io.Reader exportSearchAsCSV(String structuredQuery, String searchText, String queryOptions, String schemaName, String viewName, Long limit, com.fasterxml.jackson.databind.node.ArrayNode sortOrder, Stream columns);
/**
* Invokes the getSemanticConceptInfo operation on the database server
*
* @param semanticConceptIRI The IRI of the concept instance for which related entity instances info is to be returned
* @return The document with the IRI provided
*/
com.fasterxml.jackson.databind.JsonNode getSemanticConceptInfo(String semanticConceptIRI);
/**
* Invokes the getModelRelationships operation on the database server
*
*
* @return The relationships between entity models
*/
com.fasterxml.jackson.databind.JsonNode getModelRelationships();
/**
* Invokes the getRecord operation on the database server
*
* @param docUri The URI of the document to be returned
* @return The document with the URI provided
*/
com.fasterxml.jackson.databind.JsonNode getRecord(String docUri);
/**
* Invokes the getMatchingPropertyValues operation on the database server
*
* @param facetValuesSearchQuery provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode getMatchingPropertyValues(com.fasterxml.jackson.databind.JsonNode facetValuesSearchQuery);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy