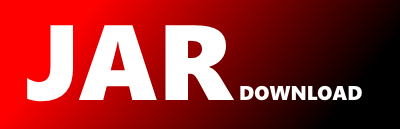
com.marklogic.hub.dataservices.FlowService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marklogic-data-hub Show documentation
Show all versions of marklogic-data-hub Show documentation
Library for Creating an Operational Data Hub on MarkLogic
package com.marklogic.hub.dataservices;
// IMPORTANT: Do not edit. This file is generated.
import com.marklogic.client.DatabaseClient;
import com.marklogic.client.impl.BaseProxy;
import com.marklogic.client.io.Format;
import com.marklogic.client.io.marker.JSONWriteHandle;
/**
* Provides a set of operations on the database server
*/
public interface FlowService {
/**
* Creates a FlowService object for executing operations on the database server.
*
* The DatabaseClientFactory class can create the DatabaseClient parameter. A single
* client object can be used for any number of requests and in multiple threads.
*
* @param db provides a client for communicating with the database server
* @return an object for executing database operations
*/
static FlowService on(DatabaseClient db) {
return on(db, null);
}
/**
* Creates a FlowService object for executing operations on the database server.
*
* The DatabaseClientFactory class can create the DatabaseClient parameter. A single
* client object can be used for any number of requests and in multiple threads.
*
* The service declaration uses a custom implementation of the same service instead
* of the default implementation of the service by specifying an endpoint directory
* in the modules database with the implementation. A service.json file with the
* declaration can be read with FileHandle or a string serialization of the JSON
* declaration with StringHandle.
*
* @param db provides a client for communicating with the database server
* @param serviceDeclaration substitutes a custom implementation of the service
* @return an object for executing database operations
*/
static FlowService on(DatabaseClient db, JSONWriteHandle serviceDeclaration) {
final class FlowServiceImpl implements FlowService {
private final DatabaseClient dbClient;
private final BaseProxy baseProxy;
private final BaseProxy.DBFunctionRequest req_updateFlow;
private final BaseProxy.DBFunctionRequest req_getFlow;
private final BaseProxy.DBFunctionRequest req_deleteFlow;
private final BaseProxy.DBFunctionRequest req_addStepToFlow;
private final BaseProxy.DBFunctionRequest req_getFlowsWithStepDetails;
private final BaseProxy.DBFunctionRequest req_getFlowWithLatestJobInfo;
private final BaseProxy.DBFunctionRequest req_getFlowsWithLatestJobInfo;
private final BaseProxy.DBFunctionRequest req_removeStepFromFlow;
private final BaseProxy.DBFunctionRequest req_createFlow;
private final BaseProxy.DBFunctionRequest req_getFullFlow;
FlowServiceImpl(DatabaseClient dbClient, JSONWriteHandle servDecl) {
this.dbClient = dbClient;
this.baseProxy = new BaseProxy("/data-hub/data-services/flow/", servDecl);
this.req_updateFlow = this.baseProxy.request(
"updateFlow.mjs", BaseProxy.ParameterValuesKind.MULTIPLE_MIXED);
this.req_getFlow = this.baseProxy.request(
"getFlow.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_deleteFlow = this.baseProxy.request(
"deleteFlow.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_addStepToFlow = this.baseProxy.request(
"addStepToFlow.mjs", BaseProxy.ParameterValuesKind.MULTIPLE_ATOMICS);
this.req_getFlowsWithStepDetails = this.baseProxy.request(
"getFlowsWithStepDetails.mjs", BaseProxy.ParameterValuesKind.NONE);
this.req_getFlowWithLatestJobInfo = this.baseProxy.request(
"getFlowWithLatestJobInfo.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_getFlowsWithLatestJobInfo = this.baseProxy.request(
"getFlowsWithLatestJobInfo.mjs", BaseProxy.ParameterValuesKind.NONE);
this.req_removeStepFromFlow = this.baseProxy.request(
"removeStepFromFlow.mjs", BaseProxy.ParameterValuesKind.MULTIPLE_ATOMICS);
this.req_createFlow = this.baseProxy.request(
"createFlow.mjs", BaseProxy.ParameterValuesKind.MULTIPLE_ATOMICS);
this.req_getFullFlow = this.baseProxy.request(
"getFullFlow.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
}
@Override
public com.fasterxml.jackson.databind.JsonNode updateFlow(String name, String description, com.fasterxml.jackson.databind.node.ArrayNode stepIds) {
return updateFlow(
this.req_updateFlow.on(this.dbClient), name, description, stepIds
);
}
private com.fasterxml.jackson.databind.JsonNode updateFlow(BaseProxy.DBFunctionRequest request, String name, String description, com.fasterxml.jackson.databind.node.ArrayNode stepIds) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("name", false, BaseProxy.StringType.fromString(name)),
BaseProxy.atomicParam("description", true, BaseProxy.StringType.fromString(description)),
BaseProxy.documentParam("stepIds", true, BaseProxy.ArrayType.fromArrayNode(stepIds))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getFlow(String name) {
return getFlow(
this.req_getFlow.on(this.dbClient), name
);
}
private com.fasterxml.jackson.databind.JsonNode getFlow(BaseProxy.DBFunctionRequest request, String name) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("name", false, BaseProxy.StringType.fromString(name))
).responseSingle(false, Format.JSON)
);
}
@Override
public void deleteFlow(String name) {
deleteFlow(
this.req_deleteFlow.on(this.dbClient), name
);
}
private void deleteFlow(BaseProxy.DBFunctionRequest request, String name) {
request
.withParams(
BaseProxy.atomicParam("name", false, BaseProxy.StringType.fromString(name))
).responseNone();
}
@Override
public com.fasterxml.jackson.databind.JsonNode addStepToFlow(String flowName, String stepName, String stepDefinitionType) {
return addStepToFlow(
this.req_addStepToFlow.on(this.dbClient), flowName, stepName, stepDefinitionType
);
}
private com.fasterxml.jackson.databind.JsonNode addStepToFlow(BaseProxy.DBFunctionRequest request, String flowName, String stepName, String stepDefinitionType) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("flowName", false, BaseProxy.StringType.fromString(flowName)),
BaseProxy.atomicParam("stepName", false, BaseProxy.StringType.fromString(stepName)),
BaseProxy.atomicParam("stepDefinitionType", false, BaseProxy.StringType.fromString(stepDefinitionType))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getFlowsWithStepDetails() {
return getFlowsWithStepDetails(
this.req_getFlowsWithStepDetails.on(this.dbClient)
);
}
private com.fasterxml.jackson.databind.JsonNode getFlowsWithStepDetails(BaseProxy.DBFunctionRequest request) {
return BaseProxy.JsonDocumentType.toJsonNode(
request.responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getFlowWithLatestJobInfo(String name) {
return getFlowWithLatestJobInfo(
this.req_getFlowWithLatestJobInfo.on(this.dbClient), name
);
}
private com.fasterxml.jackson.databind.JsonNode getFlowWithLatestJobInfo(BaseProxy.DBFunctionRequest request, String name) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("name", false, BaseProxy.StringType.fromString(name))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getFlowsWithLatestJobInfo() {
return getFlowsWithLatestJobInfo(
this.req_getFlowsWithLatestJobInfo.on(this.dbClient)
);
}
private com.fasterxml.jackson.databind.JsonNode getFlowsWithLatestJobInfo(BaseProxy.DBFunctionRequest request) {
return BaseProxy.JsonDocumentType.toJsonNode(
request.responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode removeStepFromFlow(String flowName, String stepNumber) {
return removeStepFromFlow(
this.req_removeStepFromFlow.on(this.dbClient), flowName, stepNumber
);
}
private com.fasterxml.jackson.databind.JsonNode removeStepFromFlow(BaseProxy.DBFunctionRequest request, String flowName, String stepNumber) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("flowName", false, BaseProxy.StringType.fromString(flowName)),
BaseProxy.atomicParam("stepNumber", false, BaseProxy.StringType.fromString(stepNumber))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode createFlow(String name, String description) {
return createFlow(
this.req_createFlow.on(this.dbClient), name, description
);
}
private com.fasterxml.jackson.databind.JsonNode createFlow(BaseProxy.DBFunctionRequest request, String name, String description) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("name", false, BaseProxy.StringType.fromString(name)),
BaseProxy.atomicParam("description", true, BaseProxy.StringType.fromString(description))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getFullFlow(String flowName) {
return getFullFlow(
this.req_getFullFlow.on(this.dbClient), flowName
);
}
private com.fasterxml.jackson.databind.JsonNode getFullFlow(BaseProxy.DBFunctionRequest request, String flowName) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("flowName", false, BaseProxy.StringType.fromString(flowName))
).responseSingle(false, Format.JSON)
);
}
}
return new FlowServiceImpl(db, serviceDeclaration);
}
/**
* Invokes the updateFlow operation on the database server
*
* @param name provides input
* @param description The description of the flow can be updated.
* @param stepIds The order of stepIds can be updated.
* @return Return the updated flow document
*/
com.fasterxml.jackson.databind.JsonNode updateFlow(String name, String description, com.fasterxml.jackson.databind.node.ArrayNode stepIds);
/**
* Invokes the getFlow operation on the database server
*
* @param name provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode getFlow(String name);
/**
* Invokes the deleteFlow operation on the database server
*
* @param name provides input
*
*/
void deleteFlow(String name);
/**
* Invokes the addStepToFlow operation on the database server
*
* @param flowName provides input
* @param stepName provides input
* @param stepDefinitionType provides input
* @return Return the updated flow document
*/
com.fasterxml.jackson.databind.JsonNode addStepToFlow(String flowName, String stepName, String stepDefinitionType);
/**
* Invokes the getFlowsWithStepDetails operation on the database server
*
*
* @return Return an array of flow documents, where each step has a few identifying data points and abstracts whether it's inline or referenced
*/
com.fasterxml.jackson.databind.JsonNode getFlowsWithStepDetails();
/**
* Invokes the getFlowWithLatestJobInfo operation on the database server
*
* @param name provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode getFlowWithLatestJobInfo(String name);
/**
* Invokes the getFlowsWithLatestJobInfo operation on the database server
*
*
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode getFlowsWithLatestJobInfo();
/**
* Invokes the removeStepFromFlow operation on the database server
*
* @param flowName provides input
* @param stepNumber provides input
* @return Return the updated flow document
*/
com.fasterxml.jackson.databind.JsonNode removeStepFromFlow(String flowName, String stepNumber);
/**
* Invokes the createFlow operation on the database server
*
* @param name provides input
* @param description provides input
* @return Return the created flow document
*/
com.fasterxml.jackson.databind.JsonNode createFlow(String name, String description);
/**
* Invokes the getFullFlow operation on the database server
*
* @param flowName provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode getFullFlow(String flowName);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy