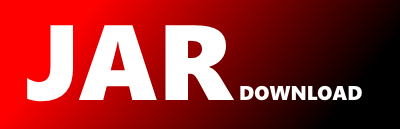
com.marklogic.hub.dataservices.MasteringService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marklogic-data-hub Show documentation
Show all versions of marklogic-data-hub Show documentation
Library for Creating an Operational Data Hub on MarkLogic
package com.marklogic.hub.dataservices;
// IMPORTANT: Do not edit. This file is generated.
import com.marklogic.client.DatabaseClient;
import com.marklogic.client.impl.BaseProxy;
import com.marklogic.client.io.Format;
import com.marklogic.client.io.marker.JSONWriteHandle;
import java.util.stream.Stream;
/**
* Provides a set of operations on the database server
*/
public interface MasteringService {
/**
* Creates a MasteringService object for executing operations on the database server.
*
* The DatabaseClientFactory class can create the DatabaseClient parameter. A single
* client object can be used for any number of requests and in multiple threads.
*
* @param db provides a client for communicating with the database server
* @return an object for executing database operations
*/
static MasteringService on(DatabaseClient db) {
return on(db, null);
}
/**
* Creates a MasteringService object for executing operations on the database server.
*
* The DatabaseClientFactory class can create the DatabaseClient parameter. A single
* client object can be used for any number of requests and in multiple threads.
*
* The service declaration uses a custom implementation of the same service instead
* of the default implementation of the service by specifying an endpoint directory
* in the modules database with the implementation. A service.json file with the
* declaration can be read with FileHandle or a string serialization of the JSON
* declaration with StringHandle.
*
* @param db provides a client for communicating with the database server
* @param serviceDeclaration substitutes a custom implementation of the service
* @return an object for executing database operations
*/
static MasteringService on(DatabaseClient db, JSONWriteHandle serviceDeclaration) {
final class MasteringServiceImpl implements MasteringService {
private final DatabaseClient dbClient;
private final BaseProxy baseProxy;
private final BaseProxy.DBFunctionRequest req_calculateMergingActivity;
private final BaseProxy.DBFunctionRequest req_validateMatchingStep;
private final BaseProxy.DBFunctionRequest req_updateMergeOptions;
private final BaseProxy.DBFunctionRequest req_calculateMatchingActivity;
private final BaseProxy.DBFunctionRequest req_updateMatchOptions;
private final BaseProxy.DBFunctionRequest req_previewMatchingActivity;
private final BaseProxy.DBFunctionRequest req_getDefaultCollections;
private final BaseProxy.DBFunctionRequest req_validateMergingStep;
MasteringServiceImpl(DatabaseClient dbClient, JSONWriteHandle servDecl) {
this.dbClient = dbClient;
this.baseProxy = new BaseProxy("/data-hub/data-services/mastering/", servDecl);
this.req_calculateMergingActivity = this.baseProxy.request(
"calculateMergingActivity.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_validateMatchingStep = this.baseProxy.request(
"validateMatchingStep.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_updateMergeOptions = this.baseProxy.request(
"updateMergeOptions.mjs", BaseProxy.ParameterValuesKind.SINGLE_NODE);
this.req_calculateMatchingActivity = this.baseProxy.request(
"calculateMatchingActivity.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_updateMatchOptions = this.baseProxy.request(
"updateMatchOptions.mjs", BaseProxy.ParameterValuesKind.SINGLE_NODE);
this.req_previewMatchingActivity = this.baseProxy.request(
"previewMatchingActivity.mjs", BaseProxy.ParameterValuesKind.MULTIPLE_ATOMICS);
this.req_getDefaultCollections = this.baseProxy.request(
"getDefaultCollections.mjs", BaseProxy.ParameterValuesKind.SINGLE_ATOMIC);
this.req_validateMergingStep = this.baseProxy.request(
"validateMergingStep.mjs", BaseProxy.ParameterValuesKind.MULTIPLE_ATOMICS);
}
@Override
public com.fasterxml.jackson.databind.JsonNode calculateMergingActivity(String stepName) {
return calculateMergingActivity(
this.req_calculateMergingActivity.on(this.dbClient), stepName
);
}
private com.fasterxml.jackson.databind.JsonNode calculateMergingActivity(BaseProxy.DBFunctionRequest request, String stepName) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("stepName", false, BaseProxy.StringType.fromString(stepName))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode validateMatchingStep(String stepName) {
return validateMatchingStep(
this.req_validateMatchingStep.on(this.dbClient), stepName
);
}
private com.fasterxml.jackson.databind.JsonNode validateMatchingStep(BaseProxy.DBFunctionRequest request, String stepName) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("stepName", false, BaseProxy.StringType.fromString(stepName))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode updateMergeOptions(com.fasterxml.jackson.databind.JsonNode options) {
return updateMergeOptions(
this.req_updateMergeOptions.on(this.dbClient), options
);
}
private com.fasterxml.jackson.databind.JsonNode updateMergeOptions(BaseProxy.DBFunctionRequest request, com.fasterxml.jackson.databind.JsonNode options) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.documentParam("options", false, BaseProxy.JsonDocumentType.fromJsonNode(options))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode calculateMatchingActivity(String stepName) {
return calculateMatchingActivity(
this.req_calculateMatchingActivity.on(this.dbClient), stepName
);
}
private com.fasterxml.jackson.databind.JsonNode calculateMatchingActivity(BaseProxy.DBFunctionRequest request, String stepName) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("stepName", false, BaseProxy.StringType.fromString(stepName))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode updateMatchOptions(com.fasterxml.jackson.databind.JsonNode options) {
return updateMatchOptions(
this.req_updateMatchOptions.on(this.dbClient), options
);
}
private com.fasterxml.jackson.databind.JsonNode updateMatchOptions(BaseProxy.DBFunctionRequest request, com.fasterxml.jackson.databind.JsonNode options) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.documentParam("options", false, BaseProxy.JsonDocumentType.fromJsonNode(options))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode previewMatchingActivity(Integer sampleSize, Stream uris, String stepName, Boolean restrictToUris, Boolean nonMatches) {
return previewMatchingActivity(
this.req_previewMatchingActivity.on(this.dbClient), sampleSize, uris, stepName, restrictToUris, nonMatches
);
}
private com.fasterxml.jackson.databind.JsonNode previewMatchingActivity(BaseProxy.DBFunctionRequest request, Integer sampleSize, Stream uris, String stepName, Boolean restrictToUris, Boolean nonMatches) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("sampleSize", false, BaseProxy.IntegerType.fromInteger(sampleSize)),
BaseProxy.atomicParam("uris", true, BaseProxy.StringType.fromString(uris)),
BaseProxy.atomicParam("stepName", false, BaseProxy.StringType.fromString(stepName)),
BaseProxy.atomicParam("restrictToUris", false, BaseProxy.BooleanType.fromBoolean(restrictToUris)),
BaseProxy.atomicParam("nonMatches", false, BaseProxy.BooleanType.fromBoolean(nonMatches))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode getDefaultCollections(String entityType) {
return getDefaultCollections(
this.req_getDefaultCollections.on(this.dbClient), entityType
);
}
private com.fasterxml.jackson.databind.JsonNode getDefaultCollections(BaseProxy.DBFunctionRequest request, String entityType) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("entityType", false, BaseProxy.StringType.fromString(entityType))
).responseSingle(false, Format.JSON)
);
}
@Override
public com.fasterxml.jackson.databind.JsonNode validateMergingStep(String stepName, String view, String entityPropertyPath) {
return validateMergingStep(
this.req_validateMergingStep.on(this.dbClient), stepName, view, entityPropertyPath
);
}
private com.fasterxml.jackson.databind.JsonNode validateMergingStep(BaseProxy.DBFunctionRequest request, String stepName, String view, String entityPropertyPath) {
return BaseProxy.JsonDocumentType.toJsonNode(
request
.withParams(
BaseProxy.atomicParam("stepName", false, BaseProxy.StringType.fromString(stepName)),
BaseProxy.atomicParam("view", false, BaseProxy.StringType.fromString(view)),
BaseProxy.atomicParam("entityPropertyPath", true, BaseProxy.StringType.fromString(entityPropertyPath))
).responseSingle(false, Format.JSON)
);
}
}
return new MasteringServiceImpl(db, serviceDeclaration);
}
/**
* Calculates tangential information about a merging step to provide configuration insights. Returns a list of source names that apply to the target Entity Type.
*
* @param stepName provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode calculateMergingActivity(String stepName);
/**
* Invokes the validateMatchingStep operation on the database server
*
* @param stepName provides input
* @return Returns an array of zero or more warning objects; each object has "level" and "message" properties
*/
com.fasterxml.jackson.databind.JsonNode validateMatchingStep(String stepName);
/**
* Invokes the updateMergeOptions operation on the database server
*
* @param options provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode updateMergeOptions(com.fasterxml.jackson.databind.JsonNode options);
/**
* Invokes the calculateMatchingActivity operation on the database server
*
* @param stepName provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode calculateMatchingActivity(String stepName);
/**
* Invokes the updateMatchOptions operation on the database server
*
* @param options provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode updateMatchOptions(com.fasterxml.jackson.databind.JsonNode options);
/**
* Invokes the previewMatchingActivity operation on the database server
*
* @param sampleSize provides input
* @param uris provides input
* @param stepName provides input
* @param restrictToUris provides input
* @param nonMatches provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode previewMatchingActivity(Integer sampleSize, Stream uris, String stepName, Boolean restrictToUris, Boolean nonMatches);
/**
* Invokes the getDefaultCollections operation on the database server
*
* @param entityType provides input
* @return as output
*/
com.fasterxml.jackson.databind.JsonNode getDefaultCollections(String entityType);
/**
* Provides feedback in the form of errors and warnings about a merge step.
*
* @param stepName provides input
* @param view Designates the view the messages are for. Valid values are 'settings' or 'rules'
* @param entityPropertyPath Restricts property warnings to a given entity property path
* @return Returns an array of zero or more warning objects; each object has "level" and "message" properties
*/
com.fasterxml.jackson.databind.JsonNode validateMergingStep(String stepName, String view, String entityPropertyPath);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy