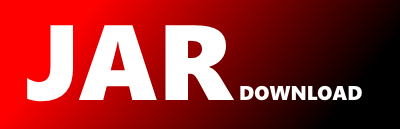
com.marklogic.hub.flow.FlowInputs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marklogic-data-hub Show documentation
Show all versions of marklogic-data-hub Show documentation
Library for Creating an Operational Data Hub on MarkLogic
package com.marklogic.hub.flow;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class FlowInputs {
private String flowName;
private List steps;
private String jobId;
private Map options;
private Map stepConfig;
public FlowInputs() {
}
public FlowInputs(String flowName) {
this.flowName = flowName;
}
public FlowInputs(String flowName, String... steps) {
this.flowName = flowName;
this.steps = Arrays.asList(steps);
}
/**
* A common step configuration to override at runtime is the inputFilePath for an ingestion step. This convenience
* method allows for doing that without modifying any existing step configuration.
*
* @param inputFilePath
*/
public void setInputFilePath(String inputFilePath) {
if (stepConfig == null) {
stepConfig = new HashMap<>();
}
@SuppressWarnings("unchecked")
Map fileLocations = (Map)stepConfig.get("fileLocations");
if (fileLocations == null) {
fileLocations = new HashMap<>();
stepConfig.put("fileLocations", fileLocations);
}
fileLocations.put("inputFilePath", inputFilePath);
}
public String getFlowName() {
return flowName;
}
public void setFlowName(String flowName) {
this.flowName = flowName;
}
public List getSteps() {
return steps;
}
public void setSteps(List steps) {
this.steps = steps;
}
public FlowInputs withSteps(String... steps) {
setSteps(Arrays.asList(steps));
return this;
}
public String getJobId() {
return jobId;
}
public void setJobId(String jobId) {
this.jobId = jobId;
}
public FlowInputs withJobId(String jobId) {
setJobId(jobId);
return this;
}
public Map getOptions() {
return options;
}
public void setOptions(Map options) {
this.options = options;
}
/**
* Convenience method for adding one option at a time.
*
* @param name
* @param value
* @return
*/
public FlowInputs withOption(String name, Object value) {
if (options == null) {
options = new HashMap<>();
}
options.put(name, value);
return this;
}
public FlowInputs withOptions(Map options) {
setOptions(options);
return this;
}
public Map getStepConfig() {
return stepConfig;
}
public void setStepConfig(Map stepConfig) {
this.stepConfig = stepConfig;
}
public FlowInputs withStepConfig(Map stepConfig) {
setStepConfig(stepConfig);
return this;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy