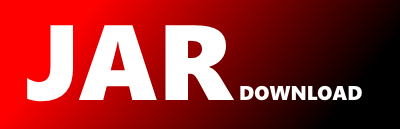
com.marklogic.hub.flow.RunFlowResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marklogic-data-hub Show documentation
Show all versions of marklogic-data-hub Show documentation
Library for Creating an Operational Data Hub on MarkLogic
package com.marklogic.hub.flow;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.SerializationFeature;
import com.marklogic.hub.step.RunStepResponse;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import static java.util.Optional.ofNullable;
public class RunFlowResponse {
String jobId;
String endTime;
String flowName;
String jobStatus;
String startTime;
String lastAttemptedStep;
String lastCompletedStep;
String user;
Map stepResponses;
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy