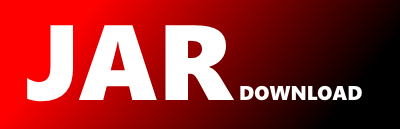
com.marklogic.hub.impl.CommandLineFlowInputs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marklogic-data-hub Show documentation
Show all versions of marklogic-data-hub Show documentation
Library for Creating an Operational Data Hub on MarkLogic
package com.marklogic.hub.impl;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.marklogic.hub.flow.FlowInputs;
import org.apache.commons.lang3.StringUtils;
import org.apache.commons.lang3.tuple.Pair;
import org.springframework.util.FileCopyUtils;
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Defines command-line-specific inputs for running a flow. Intended for use in the DHF Gradle plugin and the client JAR.
*/
public class CommandLineFlowInputs {
private String flowName;
private Integer batchSize;
private Integer threadCount;
private String inputFilePath;
private String inputFileType;
private String outputURIReplacement;
private String outputURIPrefix;
private String separator;
private Boolean failHard = false;
private List steps;
private String jobId;
private String optionsJSON;
private String optionsFile;
private Boolean showOptions = false;
public Pair buildFlowInputs() {
StringBuilder runFlowString = new StringBuilder("Running flow: [" + flowName + "]");
if (steps != null) {
runFlowString.append(", steps: ").append(steps);
}
FlowInputs flowInputs = new FlowInputs(flowName);
flowInputs.setSteps(steps);
flowInputs.setJobId(jobId);
flowInputs.setStepConfig(buildStepConfig(runFlowString));
Map flowOptions = buildFlowOptions();
flowInputs.setOptions(flowOptions);
if (showOptions && flowOptions != null) {
runFlowString.append("\n\tand options:");
for (Map.Entry entry : flowOptions.entrySet()) {
runFlowString.append("\n\t\t").append(entry.getKey()).append(" = ").append(entry.getValue());
}
}
return Pair.of(flowInputs, runFlowString.toString());
}
protected Map buildStepConfig(StringBuilder runFlowString) {
Map stepConfig = new HashMap<>();
if (batchSize != null) {
runFlowString.append("\n\twith batch size: ").append(batchSize);
stepConfig.put("batchSize", batchSize);
}
if (threadCount != null) {
runFlowString.append("\n\twith thread count: ").append(threadCount);
stepConfig.put("threadCount", threadCount);
}
if (failHard) {
runFlowString.append("\n\t\twith fail hard: ").append(failHard);
stepConfig.put("stopOnFailure", failHard);
}
if (inputFileType != null || inputFilePath != null || outputURIReplacement != null || separator != null || outputURIPrefix != null) {
runFlowString.append("\n\tWith File Locations Settings:");
Map fileLocations = new HashMap<>();
if (inputFileType != null) {
runFlowString.append("\n\t\tInput File Type: ").append(inputFileType);
fileLocations.put("inputFileType", inputFileType);
}
if (inputFilePath != null) {
runFlowString.append("\n\t\tInput File Path: ").append(inputFilePath);
fileLocations.put("inputFilePath", inputFilePath);
}
if (outputURIPrefix != null) {
runFlowString.append("\n\t\tOutput URI Prefix: ").append(outputURIPrefix);
fileLocations.put("outputURIPrefix", outputURIPrefix);
}
if (outputURIReplacement != null) {
runFlowString.append("\n\t\tOutput URI Replacement: ").append(outputURIReplacement);
fileLocations.put("outputURIReplacement", outputURIReplacement);
}
if (separator != null) {
if (inputFileType != null && !inputFileType.equalsIgnoreCase("csv")) {
throw new IllegalArgumentException("Invalid argument for file type " + inputFileType + ". When specifying a separator, the file type must be 'csv'");
}
runFlowString.append("\n\t\tSeparator: ").append(separator);
fileLocations.put("separator", separator);
}
stepConfig.put("fileLocations", fileLocations);
}
return stepConfig;
}
protected Map buildFlowOptions() {
String optionsString;
if (StringUtils.isNotEmpty(optionsFile)) {
try {
optionsString = new String(FileCopyUtils.copyToByteArray(new File(optionsFile)), StandardCharsets.UTF_8);
} catch (IOException ex) {
throw new RuntimeException("Unable to read options file at: " + optionsFile, ex);
}
} else {
optionsString = optionsJSON;
}
Map optionsMap = null;
if (StringUtils.isNotEmpty(optionsString)) {
try {
optionsMap = new ObjectMapper().readValue(optionsString, new TypeReference
© 2015 - 2024 Weber Informatics LLC | Privacy Policy