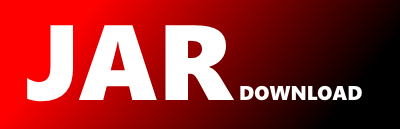
ml-modules.root.data-hub.data-services.entitySearch.exportSearchAsCSV.mjs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of marklogic-data-hub Show documentation
Show all versions of marklogic-data-hub Show documentation
Library for Creating an Operational Data Hub on MarkLogic
/*
Copyright (c) 2021 MarkLogic Corporation
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
'use strict';
xdmp.securityAssert("http://marklogic.com/data-hub/hub-central/privileges/export-entities", "execute");
import entityLib from "/data-hub/5/impl/entity-lib.mjs";
import hubUtils from "/data-hub/5/impl/hub-utils.mjs";
import httpUtils from "/data-hub/5/impl/http-utils.mjs";
import op from '/MarkLogic/optic';
const search = require('/MarkLogic/appservices/search/search.xqy');
const returnFlags = `false
false
false
false
false
false
false
false
false
false
true `;
const stylesheet = fn.head(xdmp.unquote(`
${returnFlags}
`));
const replaceHyphenWithUnderscore = (str) => {
return str.replace(/-/g, '_');
};
const filterObjectAndArrayTypeProperties = (name) => {
const entityType = entityLib.findEntityTypeByEntityName(name);
if (!entityType) {
httpUtils.throwNotFound(`Could not find an Entity Model document with name: ${name}`);
}
const filteredProperties = new Set();
const properties = entityType.properties;
Object.keys(properties).forEach((property) => {
if (!properties[property].hasOwnProperty("$ref") && properties[property].datatype !== "array") {
filteredProperties.add(property);
}
});
return filteredProperties;
};
let viewName = external.viewName;
let schemaName = external.schemaName;
let limit = external.limit;
let structuredQuery = external.structuredQuery;
let searchText = external.searchText;
let queryOptions = external.queryOptions;
let sortOrder = external.sortOrder;
let columns = external.columns;
structuredQuery = fn.head(xdmp.unquote(structuredQuery)).root;
searchText = searchText || '';
queryOptions = fn.head(xdmp.unquote(queryOptions)).root;
/*
* Filtering out columns (properties) that are of object/array type since we don't support them for now.
* Also replacing hyphen with underscore for column names (entity property names), schema names and view names since TDE's do the same.
*/
const simplePropertySet = filterObjectAndArrayTypeProperties(schemaName);
// Using Sequence.from
columns = hubUtils.normalizeToArray(columns).filter(column => simplePropertySet.has(column)).map(column => replaceHyphenWithUnderscore(column));
viewName = replaceHyphenWithUnderscore(viewName);
schemaName = replaceHyphenWithUnderscore(schemaName);
const newOptions = fn.head(xdmp.xsltEval(stylesheet, queryOptions)).root;
const searchResponse = fn.head(search.resolve(structuredQuery, newOptions));
const searchTxtResponse = fn.head(search.parse(searchText, newOptions));
const qry = cts.query(searchResponse.xpath('./*/*'));
const qryTxt = cts.query(searchTxtResponse);
const ctsQry = cts.andQuery([qry, qryTxt]);
let orderDefinitions = [];
if (sortOrder) {
// convert ArrayNode to Array with .toObject()
for (let sort of sortOrder.toObject()) {
const col = op.col(replaceHyphenWithUnderscore(sort.propertyName));
if (sort.sortDirection === "ascending") {
orderDefinitions.push(op.asc(col));
} else {
orderDefinitions.push(op.desc(col));
}
}
}
const columnIdentifiers = columns.map((colName) => op.col(colName));
// Order of the functions applied to the Optic Plan can affect the execution order, so limit must be last for proper sorting
let opticPlan = op.fromView(schemaName, viewName).select(columnIdentifiers);
if (orderDefinitions.length > 0) {
opticPlan = opticPlan.orderBy(orderDefinitions);
}
opticPlan = opticPlan.where(ctsQry);
if (limit) {
opticPlan = opticPlan.limit(limit);
}
// Not using the rows REST API due to https://bugtrack.marklogic.com/55338
let result = opticPlan.result('object');
result = hubUtils.normalizeToSequence(result);
xdmp.quote(result, {method:'sparql-results-csv'});
© 2015 - 2024 Weber Informatics LLC | Privacy Policy