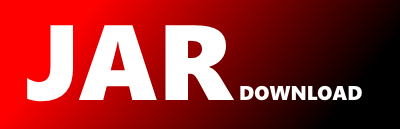
com.marvelution.bamboo.plugins.sonar.logger.SonarBuildLogFileWriter Maven / Gradle / Ivy
/*
* Licensed to Marvelution under one or more contributor license
* agreements. See the NOTICE file distributed with this work
* for additional information regarding copyright ownership.
* Marvelution licenses this file to you under the Apache License,
* Version 2.0 (the "License"); you may not use this file except
* in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.marvelution.bamboo.plugins.sonar.logger;
import com.atlassian.bamboo.build.LogEntry;
import com.atlassian.bamboo.repository.RepositoryException;
import com.atlassian.bamboo.v2.build.BuildContext;
import com.atlassian.bamboo.v2.build.repository.RepositoryV2;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import net.jcip.annotations.NotThreadSafe;
import org.jetbrains.annotations.NotNull;
/**
* Log {@link FileWriter} for the Sonar log file
*
* @author Mark Rekveld
*/
@NotThreadSafe
public class SonarBuildLogFileWriter {
@NotNull
private final File logFile;
private FileWriter writer;
/**
* Public constructor for the Sonar Log fiel writer
*
* @param buildContext the {@link BuildContext} of the currently running build
* @throws IOException in case the file writer cannot be created
* @throws RepositoryException in case the repository of the build cannot be found
*/
public SonarBuildLogFileWriter(@NotNull BuildContext buildContext) throws IOException, RepositoryException {
final RepositoryV2 repository = buildContext.getBuildPlanDefinition().getRepositoryV2();
final File logDirectory = repository.getSourceCodeDirectory(buildContext.getPlanKey());
if (!(logDirectory.exists())) {
logDirectory.mkdirs();
}
logFile = new File(logDirectory, SonarBuildLogUtils.getLogFileName(buildContext.getPlanKey(), buildContext
.getBuildNumber()));
writer = new FileWriter(logFile, false);
}
/**
* Write a {@link LogEntry} to the log file
*
* @param logEntry the {@link LogEntry} to write
* @throws IOException in case of write exceptions
*/
public void writeLog(@NotNull LogEntry logEntry) throws IOException {
writer.write(SonarBuildLogUtils.convertToLogFileEntry(logEntry));
writer.write("\n");
}
/**
* Close the connection to the {@link FileWriter}
*
* @throws IOException in case of exceptions
*/
public void close() throws IOException {
if (writer != null) {
writer.close();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy