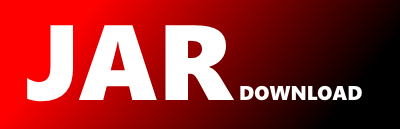
com.marvelution.hudson.plugins.apiv2.resources.model.build.Builds Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hudson-apiv2-plugin Show documentation
Show all versions of hudson-apiv2-plugin Show documentation
This plugin features a Wink REST API application.
The newest version!
/*
* Licensed to Marvelution under one or more contributor license
* agreements. See the NOTICE file distributed with this work
* for additional information regarding copyright ownership.
* Marvelution licenses this file to you under the Apache License,
* Version 2.0 (the "License"); you may not use this file except
* in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.marvelution.hudson.plugins.apiv2.resources.model.build;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementRef;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import com.google.common.base.Function;
import com.google.common.collect.Ordering;
import com.marvelution.hudson.plugins.apiv2.resources.model.ListableModel;
import com.marvelution.hudson.plugins.apiv2.resources.utils.NameSpaceUtils;
/**
* Builds XML object
*
* @author Mark Rekveld
*/
@XmlType(name = "BuildsType", namespace = NameSpaceUtils.BUILD_NAMESPACE, factoryClass = ObjectFactory.class,
factoryMethod = "createBuilds")
@XmlRootElement(name = "Builds", namespace = NameSpaceUtils.BUILD_NAMESPACE)
@XmlAccessorType(XmlAccessType.FIELD)
public class Builds extends ListableModel {
@XmlElementRef
private Collection items;
@XmlElement
private boolean hasMoreBuilds = false;
/**
* Default Constructor
*/
public Builds() {
items = new ArrayList();
}
/**
* {@inheritDoc}
*/
@Override
public Collection getItems() {
return items;
}
/**
* Getter for hasMoreBuilds
*
* @return the hasMoreBuilds
* @since 4.5.0
*/
public boolean isHasMoreBuilds() {
return hasMoreBuilds;
}
/**
* Setter for hasMoreBuilds
*
* @param hasMoreBuilds the hasMoreBuilds to set
* @since 4.5.0
*/
public void setHasMoreBuilds(boolean hasMoreBuilds) {
this.hasMoreBuilds = hasMoreBuilds;
}
/**
* Reverse sort the builds by execution time-stamp
*/
public void sortBuilds() {
Ordering ordering =
Ordering.natural().reverse().onResultOf(new Function() {
@Override
public Date apply(Build from) {
return new Date(from.getTimestamp());
}
});
items = ordering.sortedCopy(items);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy