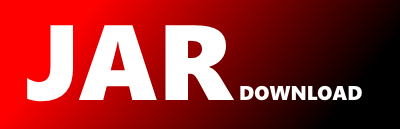
com.marvelution.jira.plugins.sonar.rest.ProjectComponentsRestResource Maven / Gradle / Ivy
/*
* Licensed to Marvelution under one or more contributor license
* agreements. See the NOTICE file distributed with this work
* for additional information regarding copyright ownership.
* Marvelution licenses this file to you under the Apache License,
* Version 2.0 (the "License"); you may not use this file except
* in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.marvelution.jira.plugins.sonar.rest;
import java.util.Collection;
import javax.ws.rs.Consumes;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import com.atlassian.jira.bc.project.component.ProjectComponent;
import com.atlassian.jira.bc.project.component.ProjectComponentManager;
import com.atlassian.plugins.rest.common.security.AnonymousAllowed;
import com.marvelution.jira.plugins.sonar.rest.model.ProjectComponentResource;
import com.marvelution.jira.plugins.sonar.rest.model.ProjectComponentsResource;
/**
* {@link ProjectComponent} REST resource
*
* @author Mark rekveld
*
* @since 3.0.0
*/
@Path("/projectComponents")
@AnonymousAllowed
@Consumes({ MediaType.APPLICATION_JSON, MediaType.APPLICATION_XML, MediaType.APPLICATION_FORM_URLENCODED })
@Produces({ MediaType.APPLICATION_JSON, MediaType.APPLICATION_XML, MediaType.APPLICATION_FORM_URLENCODED })
public class ProjectComponentsRestResource {
private final ProjectComponentManager componentManager;
/**
* Constructor
*
* @param componentManager the {@link ProjectComponentManager} implementation
*/
public ProjectComponentsRestResource(ProjectComponentManager componentManager) {
this.componentManager = componentManager;
}
/**
* Get all the {@link ProjectComponent} objects for a Jira Project
*
* @param projectId the Project Id to get all the components for
* @return the Collection of {@link ProjectComponentResource} objects in a JSON response
*/
@GET
public Response getProjectComponents(@QueryParam("projectId") Long projectId) {
final ProjectComponentsResource components = new ProjectComponentsResource();
final Collection projectComponents = componentManager.findAllForProject(projectId);
for (ProjectComponent component : projectComponents) {
components.getComponents().add(new ProjectComponentResource(component.getId(), component.getName()));
}
return Response.ok(components).build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy