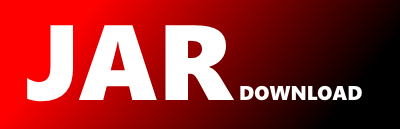
com.marvelution.maven.components.migration.manager.DefaultMigrationManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-migration-manager Show documentation
Show all versions of maven-migration-manager Show documentation
Maven 2.x Plexus Migration Manager Component used by the maven-migrator-plugin
The newest version!
/*
* Licensed to Marvelution under one or more contributor license
* agreements. See the NOTICE file distributed with this work
* for additional information regarding copyright ownership.
* Marvelution licenses this file to you under the Apache License,
* Version 2.0 (the "License"); you may not use this file except
* in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.marvelution.maven.components.migration.manager;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import org.codehaus.plexus.logging.AbstractLogEnabled;
import com.marvelution.maven.components.migration.manager.configuration.MigrationDescriptor;
import com.marvelution.maven.components.migration.manager.configuration.MigrationDescriptorStore;
import com.marvelution.maven.components.migration.manager.configuration.MigrationDescriptorStoreException;
import com.marvelution.maven.components.migration.manager.environment.MigrationEnvironment;
import com.marvelution.maven.components.migration.manager.exception.MigrationExecutionException;
import com.marvelution.maven.components.migration.manager.exception.MigrationFailureException;
import com.marvelution.maven.components.migration.manager.phases.MigrationPhase;
import com.marvelution.utils.StringUtils;
/**
* Default Migration Manager
*
* @author Mark Rekveld
*/
public class DefaultMigrationManager extends AbstractLogEnabled implements MigrationManager {
/**
* Configuration Store
*/
private MigrationDescriptorStore configStore;
/**
* Available Migration Phases
*/
private Map migrationPhases;
/**
* Phases to run to prepare a migration
*/
private List preparePhases;
/**
* Phases to run to perform the migration
*/
private List performPhases;
/**
* Phases to run to roll-back a prepared migration
*/
private List rollbackPhases;
/**
* Setter for {@link #configStore}
*
* @param configStore the {@link MigrationDescriptorStore}
*/
public void setConfigStore(MigrationDescriptorStore configStore) {
this.configStore = configStore;
}
/**
* {@inheritDoc}
*/
public void prepare(MigrationDescriptor descriptor, MigrationEnvironment environment)
throws MigrationExecutionException, MigrationFailureException {
prepare(descriptor, environment, false, true);
}
/**
* {@inheritDoc}
*/
// TODO MVNMIGRMAN-2, Implement batch-mode support
public void prepare(MigrationDescriptor descriptor, MigrationEnvironment environment, boolean dryRun,
boolean resume) throws MigrationExecutionException, MigrationFailureException {
if (!environment.isInteractive()) {
throw new MigrationFailureException("Migration preparation does not support batch-mode");
}
MigrationDescriptor config;
if (resume) {
getLogger().debug("Continuing from previous execution...");
config = readMigrationDescriptor(descriptor);
} else {
getLogger().debug("Starting from scratch...");
config = descriptor;
// Not resuming so we cannot have a completedPhase yet...
config.setCompletedPhase("");
}
final int index = preparePhases.indexOf(config.getCompletedPhase());
if (index == preparePhases.size() - 1) {
getLogger().info(getFinishedPreparationMessage("Migration preparation already completed."));
} else if (index > 0) {
getLogger().info("Resuming migration preparation from phase '" + preparePhases.get(index + 1) + "'");
} else {
getLogger().info("Starting migration preparation...");
}
final MigrationResult result = new MigrationResult();
result.setStartTime(System.currentTimeMillis());
for (int i = index + 1; i < preparePhases.size(); i++) {
final String phaseName = (String) preparePhases.get(i);
final MigrationPhase phase = getMigrationPhaseForName(phaseName);
MigrationResult phaseResult = null;
try {
if (dryRun) {
getLogger().info("[migrator:prepare {simulation: " + phaseName + "}]");
phaseResult = phase.simulate(config, environment);
} else {
getLogger().info("[migrator:prepare {execution: " + phaseName + "}]");
phaseResult = phase.execute(config, environment);
}
} finally {
if (phaseResult != null && phaseResult.getResultCode() != MigrationResult.SUCCESS) {
throw new MigrationFailureException("Execution of phase '" + phaseName + "' failed.");
} else if (phaseResult != null) {
result.setResultCode(phaseResult.getResultCode());
result.setEndTime(System.currentTimeMillis());
}
}
config.setCompletedPhase(phaseName);
try {
configStore.write(config);
} catch (MigrationDescriptorStoreException e) {
// TODO Roll-back or write a property to auto set resume to true??
throw new MigrationExecutionException("Error writing release properties after completing phase."
+ " Reason: " + e.getMessage(), e);
}
}
}
/**
* {@inheritDoc}
*/
public void perform(MigrationDescriptor descriptor, MigrationEnvironment environment)
throws MigrationExecutionException, MigrationFailureException {
perform(descriptor, environment, false);
}
/**
* {@inheritDoc}
*/
public void perform(MigrationDescriptor descriptor, MigrationEnvironment environment, boolean clean)
throws MigrationExecutionException, MigrationFailureException {
final MigrationDescriptor config = readMigrationDescriptor(descriptor);
executePhaseList(config, environment, performPhases, "perform");
if (clean) {
clean(config, environment);
}
}
/**
* {@inheritDoc}
*/
public void rollback(MigrationDescriptor descriptor, MigrationEnvironment environment)
throws MigrationExecutionException, MigrationFailureException {
final MigrationDescriptor config = readMigrationDescriptor(descriptor);
executePhaseList(config, environment, rollbackPhases, "rollback");
clean(config, environment);
}
/**
* {@inheritDoc}
*/
public void clean(MigrationDescriptor descriptor, MigrationEnvironment environment)
throws MigrationExecutionException, MigrationFailureException {
final MigrationDescriptor config = readMigrationDescriptor(descriptor);
getLogger().info("Cleaning up after migration...");
configStore.delete(config);
for (final Iterator iter = preparePhases.iterator(); iter.hasNext();) {
final MigrationPhase phase = getMigrationPhaseForName((String) iter.next());
phase.clean(config, environment);
}
}
/**
* Read {@link MigrationDescriptor} from {@link MigrationDescriptorStore}
*
* @param descriptor {@link MigrationDescriptor} to read
* @return the read {@link MigrationDescriptor}
* @throws MigrationExecutionException in case of read errors
*/
private MigrationDescriptor readMigrationDescriptor(final MigrationDescriptor descriptor)
throws MigrationExecutionException {
try {
return configStore.read(descriptor);
} catch (MigrationDescriptorStoreException e) {
throw new MigrationExecutionException("Error reading stored configuration. Reason: " + e.getMessage(), e);
}
}
/**
* Method to get the {@link MigrationPhase} for a given name
*
* @param name the name of the MigrationPhase to get
* @return the {@link MigrationPhase}
* @throws MigrationExecutionException in case no {@link MigrationPhase} is available under the given name
*/
private MigrationPhase getMigrationPhaseForName(final String name) throws MigrationExecutionException {
final MigrationPhase phase = (MigrationPhase) migrationPhases.get(name);
if (phase == null) {
throw new MigrationExecutionException("Unable to find Migration Phase '" + name + "' to execute");
}
return phase;
}
/**
* Executes all MigrationPhases from a {@link List}
*
* @param config the {@link MigrationDescriptor}
* @param environment the {@link MigrationEnvironment}
* @param phases {@link List} of MigrationPhases to execute
* @param goalName the name of the goal being executed
* @return the result of executing all the MigrationPhases
* @throws MigrationExecutionException in case of execution exceptions
* @throws MigrationFailureException in case of execution failures
*/
private MigrationResult executePhaseList(MigrationDescriptor config, MigrationEnvironment environment,
List phases, String goalName) throws MigrationExecutionException, MigrationFailureException {
final MigrationResult result = new MigrationResult();
result.setStartTime(System.currentTimeMillis());
for (final Iterator iter = phases.iterator(); iter.hasNext();) {
final String phaseName = (String) iter.next();
final MigrationPhase phase = getMigrationPhaseForName(phaseName);
MigrationResult phaseResult = null;
try {
getLogger().info("[migrator:" + goalName + " {execution: " + phaseName + "}]");
phaseResult = phase.execute(config, environment);
} finally {
if (phaseResult != null && phaseResult.getResultCode() != MigrationResult.SUCCESS) {
throw new MigrationFailureException("Execution of phase '" + phaseName + "' failed.");
} else if (phaseResult != null) {
result.setResultCode(phaseResult.getResultCode());
result.setEndTime(System.currentTimeMillis());
}
}
}
return result;
}
/**
* Gets the prepare finished message
*
* @param message the message to add to the beginning of the Tip message
* @return {@link String}
*/
public static String getFinishedPreparationMessage(String message) {
final StringBuffer stringBuffer = new StringBuffer();
StringUtils.append(stringBuffer, message, 0);
StringUtils.append(stringBuffer, "", 0);
StringUtils.append(stringBuffer, StringUtils.repeat("-", 64), 0);
StringUtils.append(stringBuffer, "You can now continue with migrator:perform", 1);
StringUtils.append(stringBuffer, StringUtils.repeat("-", 64), 0);
return stringBuffer.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy