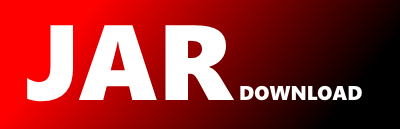
com.marvelution.maven.components.migration.manager.configuration.MigrationDescriptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-migration-manager Show documentation
Show all versions of maven-migration-manager Show documentation
Maven 2.x Plexus Migration Manager Component used by the maven-migrator-plugin
The newest version!
/*
* Licensed to Marvelution under one or more contributor license
* agreements. See the NOTICE file distributed with this work
* for additional information regarding copyright ownership.
* Marvelution licenses this file to you under the Apache License,
* Version 2.0 (the "License"); you may not use this file except
* in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.marvelution.maven.components.migration.manager.configuration;
import java.io.File;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
/**
* Migration Descriptor
*
* @author Mark Rekveld
*/
public class MigrationDescriptor {
/**
* Completed phase name
*/
private String completedPhase;
/**
* Working directory (projects basedir)
*/
private File workingDirectory;
/**
* Skip Migration of Directory structire
*/
private boolean skipDirectoryMigration;
/**
* POM Model version
*/
private String modelVersion;
/**
* Projects name
*/
private String projectName;
/**
* Projects URL
*/
private String projectUrl;
/**
* Project groupId
*/
private String groupId;
/**
* Project artifactId
*/
private String artifactId;
/**
* Project version
*/
private String version;
/**
* Project packaging
*/
private String packaging;
/**
* Project Parent
*/
private ParentProject parentProject;
/**
* Directories {@link Map}
*/
private Map directories;
/**
* JDK Revision
*/
private String jdkRevision;
/**
* Source directory
*/
private String sourceDirectory;
/**
* Resources directory
*/
private String resourcesDirectory;
/**
* Test source directory
*/
private String testSourceDirectory;
/**
* Test resources directory
*/
private String testResourcesDirectory;
/**
* WebApp directory
*/
private String webappDirectory;
/**
* EAR App directory
*/
private String earAppDirectory;
/**
* Library directory of
*/
private String libraryDirectory;
/**
* Resolved dependencies {@link Map}
*/
private Map resolvedDependencies;
/**
* {@link Set} of plugins required for the project
*/
private Set buildPlugins;
/**
* Goals to execute in prepare goals
*/
private String preparationGoals;
/**
* Goals to execute in perform goals
*/
private String performGoals;
/**
* Additional argument to pass to the MavenExecutor
*/
private String additionalArguments;
/**
* Default Constructor
*/
public MigrationDescriptor() {
this.modelVersion = "4.0.0";
this.projectName = "Migrated Project with the migrator of Marvelution.com";
this.projectUrl = "http://maven.marvelution.com";
}
/**
* Gets completed phase name
*
* @return the completedPhase
*/
public String getCompletedPhase() {
return completedPhase;
}
/**
* Sets completed phase name
*
* @param completedPhase the completedPhase to set
*/
public void setCompletedPhase(String completedPhase) {
this.completedPhase = completedPhase;
}
/**
* Gets working directory
*
* @return the workingDirectory
*/
public File getWorkingDirectory() {
return workingDirectory;
}
/**
* Sets working directory
*
* @param workingDirectory the workingDirectory to set
*/
public void setWorkingDirectory(File workingDirectory) {
this.workingDirectory = workingDirectory;
}
/**
* Gets if the directory structure needs to be migrated
*
* @return the skipDirectoryMigration
*/
public boolean isSkipDirectoryMigration() {
return skipDirectoryMigration;
}
/**
* Sets the migration if the directories is needed
*
* @param skipDirectoryMigration the skipDirectoryMigration to set
*/
public void setSkipDirectoryMigration(boolean skipDirectoryMigration) {
this.skipDirectoryMigration = skipDirectoryMigration;
}
/**
* Sets the migration if the directories is needed
*
* @param skipDirectoryMigration the skipDirectoryMigration to set
*/
public void setSkipDirectoryMigration(String skipDirectoryMigration) {
this.skipDirectoryMigration = Boolean.parseBoolean(skipDirectoryMigration);
}
/**
* Gets model version
*
* @return the modelVersion
*/
public String getModelVersion() {
return modelVersion;
}
/**
* Sets model version
*
* @param modelVersion the modelVersion to set
*/
public void setModelVersion(String modelVersion) {
this.modelVersion = modelVersion;
}
/**
* Gets project name
*
* @return the projectName
*/
public String getProjectName() {
return projectName;
}
/**
* Sets project name
*
* @param projectName the projectName to set
*/
public void setProjectName(String projectName) {
this.projectName = projectName;
}
/**
* Gets project url
*
* @return the projectUrl
*/
public String getProjectUrl() {
return projectUrl;
}
/**
* Sets project url
*
* @param projectUrl the projectUrl to set
*/
public void setProjectUrl(String projectUrl) {
this.projectUrl = projectUrl;
}
/**
* Gets groupId
*
* @return the groupId
*/
public String getGroupId() {
return groupId;
}
/**
* Sets groupId
*
* @param groupId the groupId to set
*/
public void setGroupId(String groupId) {
this.groupId = groupId;
}
/**
* Gets artifactId
*
* @return the artifactId
*/
public String getArtifactId() {
return artifactId;
}
/**
* Sets artifactId
*
* @param artifactId the artifactId to set
*/
public void setArtifactId(String artifactId) {
this.artifactId = artifactId;
}
/**
* Gets version
*
* @return the version
*/
public String getVersion() {
return version;
}
/**
* Sets version
*
* @param version the version to set
*/
public void setVersion(String version) {
this.version = version;
}
/**
* Gets packaging
*
* @return the packaging
*/
public String getPackaging() {
return packaging;
}
/**
* Sets packaging
*
* @param packaging the packaging to set
*/
public void setPackaging(String packaging) {
this.packaging = packaging;
}
/**
* Gets the {@link ParentProject} for this project
*
* @return the {@link ParentProject}
*/
public ParentProject getParentProject() {
return parentProject;
}
/**
* Sets the {@link ParentProject} for the project
*
* @param parent the {@link ParentProject}
*/
public void setParentProject(ParentProject parent) {
this.parentProject = parent;
}
/**
* Gets directories {@link Map}
*
* @return the directories
*/
public Map getDirectories() {
if (directories == null) {
directories = new HashMap();
}
return directories;
}
/**
* Sets directories {@link Map}
*
* @param directories the directories to set
*/
public void setDirectories(Map directories) {
this.directories = directories;
}
/**
* Gets the JDK Resivion
*
* @return the JDK Resivion
*/
public String getJdkRevision() {
return jdkRevision;
}
/**
* Sets the JDK Resivion
*
* @param jdkRevision the JDK Resivion to set
*/
public void setJdkRevision(String jdkRevision) {
this.jdkRevision = jdkRevision;
}
/**
* Gets source directory
*
* @return the sourceDirectory
*/
public String getSourceDirectory() {
return sourceDirectory;
}
/**
* Sets source directory
*
* @param sourceDirectory the sourceDirectory to set
*/
public void setSourceDirectory(String sourceDirectory) {
this.sourceDirectory = sourceDirectory;
}
/**
* Gets resource directory
*
* @return the resourceDirectory
*/
public String getResourcesDirectory() {
return resourcesDirectory;
}
/**
* Sets resource directory
*
* @param dirname the resourceDirectory to set
*/
public void setResourcesDirectory(String dirname) {
this.resourcesDirectory = dirname;
}
/**
* Gets test source directory
*
* @return the testSourceDirectory
*/
public String getTestSourceDirectory() {
return testSourceDirectory;
}
/**
* Sets test source directory
*
* @param testSourceDirectory the testSourceDirectory to set
*/
public void setTestSourceDirectory(String testSourceDirectory) {
this.testSourceDirectory = testSourceDirectory;
}
/**
* Gets test resource directory
*
* @return the testResourceDirectory
*/
public String getTestResourcesDirectory() {
return testResourcesDirectory;
}
/**
* Sets test resources directory
*
* @param dirname the testResourcesDirectory to set
*/
public void setTestResourcesDirectory(String dirname) {
this.testResourcesDirectory = dirname;
}
/**
* Gets WebApp directory
*
* @return the webappDirectory
*/
public String getWebappDirectory() {
return webappDirectory;
}
/**
* Sets WebApp directory
*
* @param webappDirectory the webappDirectory to set
*/
public void setWebappDirectory(String webappDirectory) {
this.webappDirectory = webappDirectory;
}
/**
* Gets EAR App directory
*
* @return the earAppDirectory
*/
public String getEarAppDirectory() {
return earAppDirectory;
}
/**
* Sets EAR app directory
*
* @param earAppDirectory the earAppDirectory to set
*/
public void setEarAppDirectory(String earAppDirectory) {
this.earAppDirectory = earAppDirectory;
}
/**
* @return the libraryDirectory
*/
public String getLibraryDirectory() {
return libraryDirectory;
}
/**
* Sets the Library directory
*
* @param libraryDirectory the libraryDirectory to set
*/
public void setLibraryDirectory(String libraryDirectory) {
this.libraryDirectory = libraryDirectory;
}
/**
* Gets resolved dependencies
*
* @return the resolvedDependencies
*/
public Map getResolvedDependencies() {
if (resolvedDependencies == null) {
resolvedDependencies = new HashMap();
}
return resolvedDependencies;
}
/**
* Sets the resolved dependencies
*
* @param resolvedDependencies the resolvedDependencies to set
*/
public void setResolvedDependencies(Map resolvedDependencies) {
this.resolvedDependencies = resolvedDependencies;
}
/**
* Gets the {@link Set} of {@link BuildPlugin}
*
* @return the buildPlugins
*/
public Set getBuildPlugins() {
if (buildPlugins == null) {
buildPlugins = new HashSet();
}
return buildPlugins;
}
/**
* Sets the {@link BuildPlugin} {@link Set}
*
* @param buildPlugins the buildPlugins to set
*/
public void setBuildPlugins(Set buildPlugins) {
this.buildPlugins = buildPlugins;
}
/**
* Gets the preparation goals
*
* @return the goals
*/
public String getPreparationGoals() {
return preparationGoals;
}
/**
* Sets the preparation goals
*
* @param preparationGoals the goals to set
*/
public void setPreparationGoals(String preparationGoals) {
this.preparationGoals = preparationGoals;
}
/**
* Gets the Perform goals
*
* @return the performGoals
*/
public String getPerformGoals() {
return performGoals;
}
/**
* Sets the Perform Goals
*
* @param performGoals the performGoals to set
*/
public void setPerformGoals(String performGoals) {
this.performGoals = performGoals;
}
/**
* Gets the additional arguments
*
* @return the additionalArguments
*/
public String getAdditionalArguments() {
return additionalArguments;
}
/**
* Sets the additional arguments
*
* @param additionalArguments the additionalArguments to set
*/
public void setAdditionalArguments(String additionalArguments) {
this.additionalArguments = additionalArguments;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy