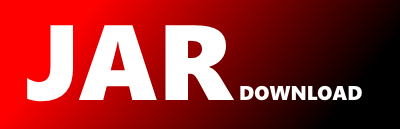
com.marvelution.maven.components.migration.manager.phases.AbstractExecuteGoalsMigrationPhase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-migration-manager Show documentation
Show all versions of maven-migration-manager Show documentation
Maven 2.x Plexus Migration Manager Component used by the maven-migrator-plugin
The newest version!
/*
* Licensed to Marvelution under one or more contributor license
* agreements. See the NOTICE file distributed with this work
* for additional information regarding copyright ownership.
* Marvelution licenses this file to you under the Apache License,
* Version 2.0 (the "License"); you may not use this file except
* in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.marvelution.maven.components.migration.manager.phases;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import com.marvelution.maven.components.maven.executor.ExecutionResult;
import com.marvelution.maven.components.maven.executor.ExecutionRequest;
import com.marvelution.maven.components.maven.executor.MavenExecutor;
import com.marvelution.maven.components.maven.executor.exception.MavenExecutorException;
import com.marvelution.maven.components.migration.manager.MigrationResult;
import com.marvelution.maven.components.migration.manager.configuration.MigrationDescriptor;
import com.marvelution.maven.components.migration.manager.environment.MigrationEnvironment;
import com.marvelution.maven.components.migration.manager.exception.MigrationExecutionException;
import com.marvelution.maven.components.migration.manager.exception.MigrationFailureException;
import com.marvelution.utils.StringUtils;
/**
* Abstract {@link MigrationPhase} to execute Goals
*
* @author Mark Rekveld
*/
public abstract class AbstractExecuteGoalsMigrationPhase extends AbstractMigrationPhase {
/**
* Component map to assist in executing Maven.
*/
private Map mavenExecutors;
/**
* {@inheritDoc}
*/
public MigrationResult execute(final MigrationDescriptor descriptor, final MigrationEnvironment environment)
throws MigrationExecutionException, MigrationFailureException {
final MigrationResult result = new MigrationResult();
try {
if (StringUtils.isNotEmpty(getGoals(descriptor)) && getGoals(descriptor).trim().length() > 0) {
getLogger().info("Executing goals '" + getGoals(descriptor).trim() + "'...");
final MavenExecutor executor = (MavenExecutor) mavenExecutors.get(environment.getMavenExecutorId());
if (executor == null) {
throw new MigrationFailureException("Cannot find MavenExecutor for id: "
+ environment.getMavenExecutorId());
}
final ExecutionResult executionResult = executor.execute(createRequest(descriptor, environment));
if (executionResult.getResultCode() != ExecutionResult.SUCCESS) {
if (executionResult.getExecutionException() != null) {
throw new MigrationExecutionException("Failed to execute goals '"
+ getGoals(descriptor).trim() + "'", executionResult.getExecutionException());
} else {
throw new MigrationExecutionException("Failed to invoke goals '" + getGoals(descriptor)
+ "' on Maven build");
}
}
} else {
getLogger().info("No goals to execute...");
}
} catch (MavenExecutorException e) {
throw new MigrationExecutionException(e.getMessage(), e);
}
result.setResultCode(MigrationResult.SUCCESS);
return result;
}
/**
* Adds a {@link MavenExecutor} to the available list of executor
*
* @param executorId the Id of the {@link MavenExecutor}
* @param executor the {@link MavenExecutor}
*/
public void setMavenExecutor(String executorId, MavenExecutor executor) {
if (mavenExecutors == null) {
mavenExecutors = new HashMap();
}
mavenExecutors.put(executorId, executor);
}
/**
* Create a {@link ExecutionRequest} by combining information from the {@link MigrationDescriptor} and the
* {@link MigrationEnvironment}
*
* @param descriptor the {@link MigrationDescriptor} to use
* @param environment the {@link MigrationEnvironment} to use
* @return the {@link ExecutionRequest}
*/
protected ExecutionRequest createRequest(final MigrationDescriptor descriptor,
final MigrationEnvironment environment) {
final ExecutionRequest request = new ExecutionRequest();
request.setBasedir(descriptor.getWorkingDirectory());
request.setGoals(Arrays.asList(StringUtils.split(getGoals(descriptor), ", ")));
request.setAdditionalArguments(descriptor.getAdditionalArguments());
request.setMavenHome(environment.getMavenHome());
request.setMavenSettings(environment.getMavenSettings());
request.setMavenExecutorId(environment.getMavenExecutorId());
request.setLocalRepository(environment.getLocalRepository());
request.setRemoteRepositories(environment.getRemoteRepositories());
request.setJavaHome(environment.getJavaHome());
return request;
}
/**
* Gets the goals to execute
*
* @param descriptor the {@link MigrationDescriptor} configuration to get the Goals from
* @return the Goals separated by a comma or space
*/
protected abstract String getGoals(final MigrationDescriptor descriptor);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy