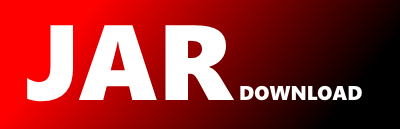
com.marvelution.maven.components.migration.manager.phases.AbstractPrompterMigrationPhase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-migration-manager Show documentation
Show all versions of maven-migration-manager Show documentation
Maven 2.x Plexus Migration Manager Component used by the maven-migrator-plugin
The newest version!
/*
* Licensed to Marvelution under one or more contributor license
* agreements. See the NOTICE file distributed with this work
* for additional information regarding copyright ownership.
* Marvelution licenses this file to you under the Apache License,
* Version 2.0 (the "License"); you may not use this file except
* in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.marvelution.maven.components.migration.manager.phases;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import org.codehaus.plexus.components.interactivity.Prompter;
import org.codehaus.plexus.components.interactivity.PrompterException;
import com.marvelution.utils.StringUtils;
/**
* Abstract Migration Phase with Plexus Prompter implementation
*
* @author Mark Rekveld
*/
public abstract class AbstractPrompterMigrationPhase extends AbstractMigrationPhase {
/**
* Plexus Prompter
*/
private Prompter prompter;
/**
* Sets the Plexus {@link Prompter}
*
* @param prompter the Plexus {@link Prompter}
*/
public void setPrompter(Prompter prompter) {
this.prompter = prompter;
}
/**
* Gets the Plxus {@link Prompter}
*
* @return {@link Prompter}
*/
public Prompter getPrompter() {
return prompter;
}
/**
* Prompts for a value for the given property
*
* @param propertyName the property name the value is for
* @return the value
* @throws PrompterException in case of {@link Prompter} exceptions
*/
protected String promptForStringResponse(String propertyName) throws PrompterException {
return promptForStringResponse(propertyName, "");
}
/**
* Prompts for a value for the given property with a default value
*
* @param propertyName the property name the value is for
* @param defaultValue default value for the property
* @return the value
* @throws PrompterException in case of {@link Prompter} exceptions
*/
protected String promptForStringResponse(String propertyName, String defaultValue) throws PrompterException {
String response = "";
if (StringUtils.isNotEmpty(defaultValue)) {
response = getPrompter().prompt("Define " + propertyName + " value:", defaultValue);
} else {
response = getPrompter().prompt("Define " + propertyName + " value");
}
return response;
}
/**
* Prompts to choose a value for the property from a {@link List} of values
*
* @param propertyName the property name the value is for
* @param options the {@link List} of possible values
* @return the value
* @throws PrompterException in case of {@link Prompter} exceptions
*/
protected String promptForStringResponse(String propertyName, List options) throws PrompterException {
return promptForStringResponse(propertyName, options, "");
}
/**
* Prompts to choose a value for the property from a {@link List} of values with a default value
*
* @param propertyName the property name the value is for
* @param options the {@link List} of possible values
* @param defaultValue default value for the property
* @return the value
* @throws PrompterException in case of {@link Prompter} exceptions
*/
protected String promptForStringResponse(String propertyName, List options, Object defaultValue)
throws PrompterException {
String query = "Choose ";
if (StringUtils.startsWithVowel(propertyName)) {
query += "an ";
} else {
query += "a ";
}
query += propertyName + ":\n";
int counter = 1, defaultValueIndex = -1;
final Map answerMap = new HashMap();
final List answers = new ArrayList();
for (final Iterator iter = options.iterator(); iter.hasNext();) {
final Object option = iter.next();
answerMap.put(String.valueOf(counter), option);
answers.add(String.valueOf(counter));
query += counter + ": " + option.toString() + "\n";
if (defaultValue.equals(option)) {
defaultValueIndex = counter;
}
counter++;
}
query += "Choose a number: ";
String answer;
if (defaultValueIndex != -1) {
answer = getPrompter().prompt(query, answers, String.valueOf(defaultValueIndex));
} else {
answer = getPrompter().prompt(query, answers);
}
return answerMap.get(answer).toString();
}
/**
* Prompt for a Yes/No answer to the question
*
* @param question the question that requires a Yes/No response
* @return {@link Boolean}
* @throws PrompterException in case of {@link Prompter} exceptions
*/
protected boolean promptForYesNoResponse(String question) throws PrompterException {
return promptForYesNoResponse(question, false);
}
/**
* Prompt for a Yes/No answer to the question
*
* @param question the question that requires a Yes/No response
* @param defaultValue the default answer
* @return {@link Boolean}
* @throws PrompterException in case of {@link Prompter} exceptions
*/
protected boolean promptForYesNoResponse(String question, boolean defaultValue) throws PrompterException {
final String response =
getPrompter().prompt(question, Arrays.asList(new String[] {"Yes", "No"}), (defaultValue ? "Yes" : "No"));
return (response.toLowerCase(Locale.ENGLISH).startsWith("y"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy