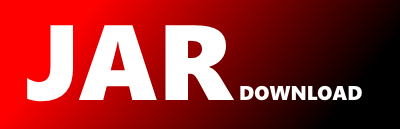
com.marvelution.maven.components.migration.manager.phases.prepare.GeneratePomPhase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of maven-migration-manager Show documentation
Show all versions of maven-migration-manager Show documentation
Maven 2.x Plexus Migration Manager Component used by the maven-migrator-plugin
The newest version!
/*
* Licensed to Marvelution under one or more contributor license
* agreements. See the NOTICE file distributed with this work
* for additional information regarding copyright ownership.
* Marvelution licenses this file to you under the Apache License,
* Version 2.0 (the "License"); you may not use this file except
* in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.marvelution.maven.components.migration.manager.phases.prepare;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
import java.util.Map.Entry;
import org.apache.maven.model.Build;
import org.apache.maven.model.Dependency;
import org.apache.maven.model.Model;
import org.apache.maven.model.Parent;
import org.codehaus.plexus.util.FileUtils;
import com.marvelution.maven.components.migration.manager.MigrationResult;
import com.marvelution.maven.components.migration.manager.configuration.BuildPlugin;
import com.marvelution.maven.components.migration.manager.configuration.MigrationDescriptor;
import com.marvelution.maven.components.migration.manager.configuration.ParentProject;
import com.marvelution.maven.components.migration.manager.environment.MigrationEnvironment;
import com.marvelution.maven.components.migration.manager.exception.MigrationExecutionException;
import com.marvelution.maven.components.migration.manager.exception.MigrationFailureException;
import com.marvelution.maven.components.migration.manager.phases.AbstractMigrationPhase;
import com.marvelution.utils.StringUtils;
import com.marvelution.utils.maven.model.BuildUtils;
import com.marvelution.utils.maven.model.ModelUtils;
import com.marvelution.utils.maven.model.PluginUtils;
/**
* {@link MigrationPhase} to generate the pom.xml
for a project
*
* @author Mark Rekveld
*/
public class GeneratePomPhase extends AbstractMigrationPhase {
/**
* {@inheritDoc}
*/
public MigrationResult execute(final MigrationDescriptor descriptor, final MigrationEnvironment environment)
throws MigrationExecutionException, MigrationFailureException {
final MigrationResult result = new MigrationResult();
final Model pomModel = generateProjectObjectModel(descriptor);
if (descriptor.isSkipDirectoryMigration()) {
generatePomBuildElement(pomModel, descriptor);
}
addPluginsToBuild(pomModel.getBuild(), descriptor.getBuildPlugins());
writeGeneratedPomModelToFile(pomModel, descriptor.getWorkingDirectory());
result.setResultCode(MigrationResult.SUCCESS);
return result;
}
/**
* {@inheritDoc}
*/
public MigrationResult simulate(final MigrationDescriptor descriptor, final MigrationEnvironment environment)
throws MigrationExecutionException, MigrationFailureException {
final Model pomModel = generateProjectObjectModel(descriptor);
generatePomBuildElement(pomModel, descriptor);
addPluginsToBuild(pomModel.getBuild(), descriptor.getBuildPlugins());
writeGeneratedPomModelToFile(pomModel, descriptor.getWorkingDirectory());
return getMigrationResultSuccess();
}
/**
* {@inheritDoc}
*/
public MigrationResult clean(MigrationDescriptor descriptor, MigrationEnvironment environment) {
final MigrationResult result = getMigrationResultSuccess();
result.setStartTime(System.currentTimeMillis());
final File backup = new File(descriptor.getWorkingDirectory(), ModelUtils.POMV4 + ".migrationBackup");
if (backup.exists()) {
try {
FileUtils.forceDelete(backup);
} catch (IOException e) {
result.setResultCode(MigrationResult.ERROR);
}
}
result.setEndTime(System.currentTimeMillis());
return result;
}
/**
* Write the generated {@link Model} to {@link File}
*
* @param model the {@link Model} to write
* @param basedir the base directory of the project
* @throws MigrationExecutionException in case of write exceptions
*/
private void writeGeneratedPomModelToFile(Model model, File basedir) throws MigrationExecutionException {
final File pomFile = new File(basedir, ModelUtils.POMV4);
try {
ModelUtils.write(model, pomFile);
getLogger().info("Wrote POM Model to pom File: " + pomFile.getAbsolutePath());
} catch (Exception e) {
throw new MigrationExecutionException("Error writing POM Model to File: " + pomFile.getAbsolutePath(), e);
}
}
/**
* Validate Migration Descriptor for Projects GAV (GroupId, ArtifactId and Version)
*
* @param descriptor the {@link MigrationDescriptor} to validate
* @throws MigrationFailureException in case the groupId, artifactId or version is empty
*/
private void validateMigrationDescriptorGav(MigrationDescriptor descriptor) throws MigrationFailureException {
if (StringUtils.isEmpty(descriptor.getGroupId())) {
throw new MigrationFailureException("groupId may not be empty");
} else if (StringUtils.isEmpty(descriptor.getArtifactId())) {
throw new MigrationFailureException("artifactId may not be empty");
} else if (StringUtils.isEmpty(descriptor.getVersion())) {
throw new MigrationFailureException("version may not be empty");
}
}
/**
* Generates the POM {@link Model} from the {@link MigrationDescriptor} provided
*
* @param descriptor the configuration to use
* @return the generated {@link Model}
* @throws MigrationFailureException in case the {@link MigrationDescriptor} has invalid GAV settings
*/
// TODO Maybe add scm information to the POM this will need an extra phase to obtain the scm information
private Model generateProjectObjectModel(MigrationDescriptor descriptor) throws MigrationFailureException {
getLogger().info("Populating POM Model in preparation for writing the projects new " + ModelUtils.POMV4);
validateMigrationDescriptorGav(descriptor);
final Model pomModel = getProjectModel(descriptor);
pomModel.setModelVersion(descriptor.getModelVersion());
pomModel.setGroupId(descriptor.getGroupId());
pomModel.setArtifactId(descriptor.getArtifactId());
pomModel.setVersion(descriptor.getVersion());
pomModel.setPackaging(descriptor.getPackaging());
if (descriptor.getParentProject() != null) {
final ParentProject parentProject = descriptor.getParentProject();
final Parent pomParent = new Parent();
pomParent.setGroupId(parentProject.getGroupId());
pomParent.setArtifactId(parentProject.getArtifactId());
pomParent.setVersion(parentProject.getVersion());
pomModel.setParent(pomParent);
} else {
pomModel.setParent(null);
}
pomModel.setName(descriptor.getProjectName());
pomModel.setUrl(descriptor.getProjectUrl());
for (final Iterator iter = descriptor.getResolvedDependencies().entrySet().iterator(); iter.hasNext();) {
final Entry entry = (Entry) iter.next();
pomModel.addDependency((Dependency) entry.getValue());
}
pomModel.setBuild(new Build());
return pomModel;
}
/**
* Gets the Project Model object (new or from an existing pom.xml)
*
* @param descriptor the {@link MigrationDescriptor}
* @return the {@link Model}
*/
private Model getProjectModel(MigrationDescriptor descriptor) {
Model model;
final File pom = new File(descriptor.getWorkingDirectory(), ModelUtils.POMV4);
if (pom.exists()) {
try {
model = ModelUtils.read(pom);
final File backup = new File(descriptor.getWorkingDirectory(), ModelUtils.POMV4 + ".migrationBackup");
if (!backup.exists()) {
getLogger().info("Creating backup from original pom file");
// Create a backup of the existing pom.xml file for roll-back
FileUtils.copyFile(pom, backup);
} else {
getLogger().warn("Skipping creating backup from orginial pom file. Backup file allready exists.");
}
} catch (Exception e) {
getLogger().warn("Failed to read Model from pom file '" + pom.getAbsolutePath()
+ "'. Using a black one instead.");
model = new Model();
}
} else {
model = new Model();
}
return model;
}
/**
* Generates the {@link Build} element of the POM
*
* @param pom the {@link Model} to add the {@link Build} element to
* @param descriptor the configuration to use to generate the {@link Build} element
*/
private void generatePomBuildElement(Model pom, MigrationDescriptor descriptor) {
getLogger().info("Projects directory structure will not be migrated; configuring directories in POM");
final List resources = new ArrayList();
resources.add(descriptor.getResourcesDirectory());
final List testResources = new ArrayList();
testResources.add(descriptor.getTestResourcesDirectory());
pom.setBuild(BuildUtils.createBuildAndSetDirectories(descriptor.getSourceDirectory(), descriptor
.getTestSourceDirectory(), resources, testResources));
}
/**
* adds the BuildPlugins to the {@link Build}
*
* @param build the {@link Build} element to add the plugins to
* @param buildPlugins the {@link Set} of BuildPlugins to add
*/
private void addPluginsToBuild(final Build build, final Set buildPlugins) {
final List plugins = build.getPlugins();
for (final Iterator iter = buildPlugins.iterator(); iter.hasNext();) {
final BuildPlugin buildPlugin = (BuildPlugin) iter.next();
getLogger().info("Configuration required plugin: " + buildPlugin.getManagementKey());
plugins.add(PluginUtils.createPlugin(buildPlugin.getGroupId(), buildPlugin.getArtifactId(), buildPlugin
.getVersion(), buildPlugin.getConfiguration()));
}
build.setPlugins(plugins);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy