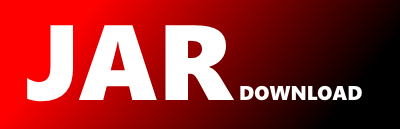
system.messaging.MessageQueue Maven / Gradle / Ivy
/*
* MIT License
*
* Copyright (c) 2024 MASES s.r.l.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**************************************************************************************
*
* This code was generated from a template using JCOReflector v. 1.14.0.0
*
* Manual changes to this file may cause unexpected behavior in your application.
* Manual changes to this file will be overwritten if the code is regenerated.
*
*************************************************************************************/
package system.messaging;
import org.mases.jcobridge.*;
import org.mases.jcobridge.netreflection.*;
import java.util.ArrayList;
// Import section
import system.componentmodel.Component;
import system.messaging.QueueAccessMode;
import system.Guid;
import system.IAsyncResult;
import system.IAsyncResultImplementation;
import system.TimeSpan;
import system.messaging.Cursor;
import system.messaging.PeekAction;
import system.AsyncCallback;
import system.messaging.Message;
import system.messaging.MessageLookupAction;
import system.messaging.MessageQueueTransaction;
import system.messaging.MessageQueueTransactionType;
import system.messaging.MessageEnumerator;
import system.messaging.MessageQueue;
import system.messaging.MessageQueueCriteria;
import system.messaging.MessageQueueEnumerator;
import system.messaging.SecurityContext;
import system.messaging.AccessControlList;
import system.messaging.MessageQueueAccessControlEntry;
import system.messaging.MessageQueueAccessRights;
import system.messaging.AccessControlEntryType;
import system.componentmodel.ISynchronizeInvoke;
import system.componentmodel.ISynchronizeInvokeImplementation;
import system.DateTime;
import system.messaging.DefaultPropertiesToSend;
import system.messaging.EncryptionRequired;
import system.messaging.IMessageFormatter;
import system.messaging.IMessageFormatterImplementation;
import system.messaging.MessagePropertyFilter;
import system.messaging.PeekCompletedEventHandler;
import system.messaging.ReceiveCompletedEventHandler;
/**
* The base .NET class managing System.Messaging.MessageQueue, System.Messaging, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b03f5f7f11d50a3a.
*
*
* .NET documentation at https://docs.microsoft.com/en-us/dotnet/api/System.Messaging.MessageQueue
*
*
* Powered by JCOBridge: more info at https://www.jcobridge.com
*
* @author MASES s.r.l https://masesgroup.com
* @version 1.14.0.0
*/
public class MessageQueue extends Component {
/**
* Fully assembly qualified name: System.Messaging, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b03f5f7f11d50a3a
*/
public static final String assemblyFullName = "System.Messaging, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b03f5f7f11d50a3a";
/**
* Assembly name: System.Messaging
*/
public static final String assemblyShortName = "System.Messaging";
/**
* Qualified class name: System.Messaging.MessageQueue
*/
public static final String className = "System.Messaging.MessageQueue";
static JCOBridge bridge = JCOBridgeInstance.getInstance(assemblyFullName);
/**
* The type managed from JCOBridge. See {@link JCType}
*/
public static JCType classType = createType();
static JCEnum enumInstance = null;
JCObject classInstance = null;
static JCType createType() {
try {
String classToCreate = className + ", "
+ (JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
if (JCOReflector.getDebug())
JCOReflector.writeLog("Creating %s", classToCreate);
JCType typeCreated = bridge.GetType(classToCreate);
if (JCOReflector.getDebug())
JCOReflector.writeLog("Created: %s",
(typeCreated != null) ? typeCreated.toString() : "Returned null value");
return typeCreated;
} catch (JCException e) {
JCOReflector.writeLog(e);
return null;
}
}
void addReference(String ref) throws Throwable {
try {
bridge.AddReference(ref);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
/**
* Internal constructor. Use with caution
*/
public MessageQueue(java.lang.Object instance) throws Throwable {
super(instance);
if (instance instanceof JCObject) {
classInstance = (JCObject) instance;
} else
throw new Exception("Cannot manage object, it is not a JCObject");
}
public String getJCOAssemblyName() {
return assemblyFullName;
}
public String getJCOClassName() {
return className;
}
public String getJCOObjectName() {
return className + ", " + (JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
}
public java.lang.Object getJCOInstance() {
return classInstance;
}
public void setJCOInstance(JCObject instance) {
classInstance = instance;
super.setJCOInstance(classInstance);
}
public JCType getJCOType() {
return classType;
}
/**
* Try to cast the {@link IJCOBridgeReflected} instance into {@link MessageQueue}, a cast assert is made to check if types are compatible.
* @param from {@link IJCOBridgeReflected} instance to be casted
* @return {@link MessageQueue} instance
* @throws java.lang.Throwable in case of error during cast operation
*/
public static MessageQueue cast(IJCOBridgeReflected from) throws Throwable {
NetType.AssertCast(classType, from);
return new MessageQueue(from.getJCOInstance());
}
// Constructors section
public MessageQueue() throws Throwable {
try {
// add reference to assemblyName.dll file
addReference(JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
setJCOInstance((JCObject)classType.NewObject());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public MessageQueue(java.lang.String path) throws Throwable {
try {
// add reference to assemblyName.dll file
addReference(JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
setJCOInstance((JCObject)classType.NewObject(path));
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public MessageQueue(java.lang.String path, boolean sharedModeDenyReceive) throws Throwable {
try {
// add reference to assemblyName.dll file
addReference(JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
setJCOInstance((JCObject)classType.NewObject(path, sharedModeDenyReceive));
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public MessageQueue(java.lang.String path, boolean sharedModeDenyReceive, boolean enableCache) throws Throwable {
try {
// add reference to assemblyName.dll file
addReference(JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
setJCOInstance((JCObject)classType.NewObject(path, sharedModeDenyReceive, enableCache));
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public MessageQueue(java.lang.String path, boolean sharedModeDenyReceive, boolean enableCache, QueueAccessMode accessMode) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.componentmodel.InvalidEnumArgumentException {
try {
// add reference to assemblyName.dll file
addReference(JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
setJCOInstance((JCObject)classType.NewObject(path, sharedModeDenyReceive, enableCache, accessMode == null ? null : accessMode.getJCOInstance()));
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public MessageQueue(java.lang.String path, QueueAccessMode accessMode) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.ArgumentOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException {
try {
// add reference to assemblyName.dll file
addReference(JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
setJCOInstance((JCObject)classType.NewObject(path, accessMode == null ? null : accessMode.getJCOInstance()));
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
// Methods section
public static boolean Exists(java.lang.String path) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.threading.AbandonedMutexException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (boolean)classType.Invoke("Exists", path);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static Guid GetMachineId(java.lang.String machineName) throws Throwable, system.NullReferenceException, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objGetMachineId = (JCObject)classType.Invoke("GetMachineId", machineName);
return new Guid(objGetMachineId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IAsyncResult BeginPeek() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.security.SecurityException, system.threading.WaitHandleCannotBeOpenedException, system.NotSupportedException, system.io.FileNotFoundException, system.io.DirectoryNotFoundException, system.UnauthorizedAccessException, system.io.IOException, system.io.PathTooLongException, system.io.DriveNotFoundException, system.OperationCanceledException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objBeginPeek = (JCObject)classInstance.Invoke("BeginPeek");
return new IAsyncResultImplementation(objBeginPeek);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IAsyncResult BeginPeek(TimeSpan timeout) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.security.SecurityException, system.threading.WaitHandleCannotBeOpenedException, system.NotSupportedException, system.io.FileNotFoundException, system.io.DirectoryNotFoundException, system.UnauthorizedAccessException, system.io.IOException, system.io.PathTooLongException, system.io.DriveNotFoundException, system.OperationCanceledException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objBeginPeek = (JCObject)classInstance.Invoke("BeginPeek", timeout == null ? null : timeout.getJCOInstance());
return new IAsyncResultImplementation(objBeginPeek);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IAsyncResult BeginPeek(TimeSpan timeout, Cursor cursor, PeekAction action, NetObject state, AsyncCallback callback) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.security.SecurityException, system.threading.WaitHandleCannotBeOpenedException, system.io.FileNotFoundException, system.io.DirectoryNotFoundException, system.UnauthorizedAccessException, system.io.IOException, system.io.PathTooLongException, system.io.DriveNotFoundException, system.OperationCanceledException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objBeginPeek = (JCObject)classInstance.Invoke("BeginPeek", timeout == null ? null : timeout.getJCOInstance(), cursor == null ? null : cursor.getJCOInstance(), action == null ? null : action.getJCOInstance(), state == null ? null : state.getJCOInstance(), callback);
return new IAsyncResultImplementation(objBeginPeek);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IAsyncResult BeginPeek(TimeSpan timeout, NetObject stateObject) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.security.SecurityException, system.threading.WaitHandleCannotBeOpenedException, system.NotSupportedException, system.io.FileNotFoundException, system.io.DirectoryNotFoundException, system.UnauthorizedAccessException, system.io.IOException, system.io.PathTooLongException, system.io.DriveNotFoundException, system.OperationCanceledException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objBeginPeek = (JCObject)classInstance.Invoke("BeginPeek", timeout == null ? null : timeout.getJCOInstance(), stateObject == null ? null : stateObject.getJCOInstance());
return new IAsyncResultImplementation(objBeginPeek);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IAsyncResult BeginPeek(TimeSpan timeout, NetObject stateObject, AsyncCallback callback) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.security.SecurityException, system.threading.WaitHandleCannotBeOpenedException, system.NotSupportedException, system.io.FileNotFoundException, system.io.DirectoryNotFoundException, system.UnauthorizedAccessException, system.io.IOException, system.io.PathTooLongException, system.io.DriveNotFoundException, system.OperationCanceledException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objBeginPeek = (JCObject)classInstance.Invoke("BeginPeek", timeout == null ? null : timeout.getJCOInstance(), stateObject == null ? null : stateObject.getJCOInstance(), callback);
return new IAsyncResultImplementation(objBeginPeek);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IAsyncResult BeginReceive() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.security.SecurityException, system.threading.WaitHandleCannotBeOpenedException, system.NotSupportedException, system.io.FileNotFoundException, system.io.DirectoryNotFoundException, system.UnauthorizedAccessException, system.io.IOException, system.io.PathTooLongException, system.io.DriveNotFoundException, system.OperationCanceledException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objBeginReceive = (JCObject)classInstance.Invoke("BeginReceive");
return new IAsyncResultImplementation(objBeginReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IAsyncResult BeginReceive(TimeSpan timeout) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.security.SecurityException, system.threading.WaitHandleCannotBeOpenedException, system.NotSupportedException, system.io.FileNotFoundException, system.io.DirectoryNotFoundException, system.UnauthorizedAccessException, system.io.IOException, system.io.PathTooLongException, system.io.DriveNotFoundException, system.OperationCanceledException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objBeginReceive = (JCObject)classInstance.Invoke("BeginReceive", timeout == null ? null : timeout.getJCOInstance());
return new IAsyncResultImplementation(objBeginReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IAsyncResult BeginReceive(TimeSpan timeout, Cursor cursor, NetObject state, AsyncCallback callback) throws Throwable, system.ArgumentNullException, system.ObjectDisposedException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.security.SecurityException, system.threading.WaitHandleCannotBeOpenedException, system.NotSupportedException, system.io.FileNotFoundException, system.io.DirectoryNotFoundException, system.UnauthorizedAccessException, system.io.IOException, system.io.PathTooLongException, system.io.DriveNotFoundException, system.OperationCanceledException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objBeginReceive = (JCObject)classInstance.Invoke("BeginReceive", timeout == null ? null : timeout.getJCOInstance(), cursor == null ? null : cursor.getJCOInstance(), state == null ? null : state.getJCOInstance(), callback);
return new IAsyncResultImplementation(objBeginReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IAsyncResult BeginReceive(TimeSpan timeout, NetObject stateObject) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.security.SecurityException, system.threading.WaitHandleCannotBeOpenedException, system.NotSupportedException, system.io.FileNotFoundException, system.io.DirectoryNotFoundException, system.UnauthorizedAccessException, system.io.IOException, system.io.PathTooLongException, system.io.DriveNotFoundException, system.OperationCanceledException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objBeginReceive = (JCObject)classInstance.Invoke("BeginReceive", timeout == null ? null : timeout.getJCOInstance(), stateObject == null ? null : stateObject.getJCOInstance());
return new IAsyncResultImplementation(objBeginReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IAsyncResult BeginReceive(TimeSpan timeout, NetObject stateObject, AsyncCallback callback) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.security.SecurityException, system.threading.WaitHandleCannotBeOpenedException, system.NotSupportedException, system.io.FileNotFoundException, system.io.DirectoryNotFoundException, system.UnauthorizedAccessException, system.io.IOException, system.io.PathTooLongException, system.io.DriveNotFoundException, system.OperationCanceledException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objBeginReceive = (JCObject)classInstance.Invoke("BeginReceive", timeout == null ? null : timeout.getJCOInstance(), stateObject == null ? null : stateObject.getJCOInstance(), callback);
return new IAsyncResultImplementation(objBeginReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Cursor CreateCursor() throws Throwable, system.ArgumentException, system.ArgumentNullException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.NullReferenceException, system.OutOfMemoryException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objCreateCursor = (JCObject)classInstance.Invoke("CreateCursor");
return new Cursor(objCreateCursor);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message EndPeek(IAsyncResult asyncResult) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.threading.AbandonedMutexException, system.messaging.MessageQueueException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objEndPeek = (JCObject)classInstance.Invoke("EndPeek", asyncResult == null ? null : asyncResult.getJCOInstance());
return new Message(objEndPeek);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message EndReceive(IAsyncResult asyncResult) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.threading.AbandonedMutexException, system.messaging.MessageQueueException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objEndReceive = (JCObject)classInstance.Invoke("EndReceive", asyncResult == null ? null : asyncResult.getJCOInstance());
return new Message(objEndReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Peek() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.OutOfMemoryException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objPeek = (JCObject)classInstance.Invoke("Peek");
return new Message(objPeek);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Peek(TimeSpan timeout) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.OutOfMemoryException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objPeek = (JCObject)classInstance.Invoke("Peek", timeout == null ? null : timeout.getJCOInstance());
return new Message(objPeek);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Peek(TimeSpan timeout, Cursor cursor, PeekAction action) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objPeek = (JCObject)classInstance.Invoke("Peek", timeout == null ? null : timeout.getJCOInstance(), cursor == null ? null : cursor.getJCOInstance(), action == null ? null : action.getJCOInstance());
return new Message(objPeek);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message PeekByCorrelationId(java.lang.String correlationId) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objPeekByCorrelationId = (JCObject)classInstance.Invoke("PeekByCorrelationId", correlationId);
return new Message(objPeekByCorrelationId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message PeekByCorrelationId(java.lang.String correlationId, TimeSpan timeout) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objPeekByCorrelationId = (JCObject)classInstance.Invoke("PeekByCorrelationId", correlationId, timeout == null ? null : timeout.getJCOInstance());
return new Message(objPeekByCorrelationId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message PeekById(java.lang.String id) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objPeekById = (JCObject)classInstance.Invoke("PeekById", id);
return new Message(objPeekById);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message PeekById(java.lang.String id, TimeSpan timeout) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objPeekById = (JCObject)classInstance.Invoke("PeekById", id, timeout == null ? null : timeout.getJCOInstance());
return new Message(objPeekById);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message PeekByLookupId(long lookupId) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.PlatformNotSupportedException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objPeekByLookupId = (JCObject)classInstance.Invoke("PeekByLookupId", lookupId);
return new Message(objPeekByLookupId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message PeekByLookupId(MessageLookupAction action, long lookupId) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.PlatformNotSupportedException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objPeekByLookupId = (JCObject)classInstance.Invoke("PeekByLookupId", action == null ? null : action.getJCOInstance(), lookupId);
return new Message(objPeekByLookupId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Receive() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.OutOfMemoryException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceive = (JCObject)classInstance.Invoke("Receive");
return new Message(objReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Receive(MessageQueueTransaction transaction) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.OutOfMemoryException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceive = (JCObject)classInstance.Invoke("Receive", transaction == null ? null : transaction.getJCOInstance());
return new Message(objReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Receive(MessageQueueTransactionType transactionType) throws Throwable, system.ArgumentNullException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceive = (JCObject)classInstance.Invoke("Receive", transactionType == null ? null : transactionType.getJCOInstance());
return new Message(objReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Receive(TimeSpan timeout) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.OutOfMemoryException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceive = (JCObject)classInstance.Invoke("Receive", timeout == null ? null : timeout.getJCOInstance());
return new Message(objReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Receive(TimeSpan timeout, Cursor cursor) throws Throwable, system.ArgumentNullException, system.ObjectDisposedException, system.ArgumentException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.OutOfMemoryException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceive = (JCObject)classInstance.Invoke("Receive", timeout == null ? null : timeout.getJCOInstance(), cursor == null ? null : cursor.getJCOInstance());
return new Message(objReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Receive(TimeSpan timeout, Cursor cursor, MessageQueueTransaction transaction) throws Throwable, system.ArgumentNullException, system.ObjectDisposedException, system.ArgumentException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.OutOfMemoryException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceive = (JCObject)classInstance.Invoke("Receive", timeout == null ? null : timeout.getJCOInstance(), cursor == null ? null : cursor.getJCOInstance(), transaction == null ? null : transaction.getJCOInstance());
return new Message(objReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Receive(TimeSpan timeout, Cursor cursor, MessageQueueTransactionType transactionType) throws Throwable, system.ArgumentNullException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceive = (JCObject)classInstance.Invoke("Receive", timeout == null ? null : timeout.getJCOInstance(), cursor == null ? null : cursor.getJCOInstance(), transactionType == null ? null : transactionType.getJCOInstance());
return new Message(objReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Receive(TimeSpan timeout, MessageQueueTransaction transaction) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.OutOfMemoryException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceive = (JCObject)classInstance.Invoke("Receive", timeout == null ? null : timeout.getJCOInstance(), transaction == null ? null : transaction.getJCOInstance());
return new Message(objReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message Receive(TimeSpan timeout, MessageQueueTransactionType transactionType) throws Throwable, system.ArgumentNullException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceive = (JCObject)classInstance.Invoke("Receive", timeout == null ? null : timeout.getJCOInstance(), transactionType == null ? null : transactionType.getJCOInstance());
return new Message(objReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveByCorrelationId(java.lang.String correlationId) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveByCorrelationId = (JCObject)classInstance.Invoke("ReceiveByCorrelationId", correlationId);
return new Message(objReceiveByCorrelationId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveByCorrelationId(java.lang.String correlationId, MessageQueueTransaction transaction) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveByCorrelationId = (JCObject)classInstance.Invoke("ReceiveByCorrelationId", correlationId, transaction == null ? null : transaction.getJCOInstance());
return new Message(objReceiveByCorrelationId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveByCorrelationId(java.lang.String correlationId, MessageQueueTransactionType transactionType) throws Throwable, system.ArgumentNullException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveByCorrelationId = (JCObject)classInstance.Invoke("ReceiveByCorrelationId", correlationId, transactionType == null ? null : transactionType.getJCOInstance());
return new Message(objReceiveByCorrelationId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveByCorrelationId(java.lang.String correlationId, TimeSpan timeout) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveByCorrelationId = (JCObject)classInstance.Invoke("ReceiveByCorrelationId", correlationId, timeout == null ? null : timeout.getJCOInstance());
return new Message(objReceiveByCorrelationId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveByCorrelationId(java.lang.String correlationId, TimeSpan timeout, MessageQueueTransaction transaction) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveByCorrelationId = (JCObject)classInstance.Invoke("ReceiveByCorrelationId", correlationId, timeout == null ? null : timeout.getJCOInstance(), transaction == null ? null : transaction.getJCOInstance());
return new Message(objReceiveByCorrelationId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveByCorrelationId(java.lang.String correlationId, TimeSpan timeout, MessageQueueTransactionType transactionType) throws Throwable, system.ArgumentNullException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveByCorrelationId = (JCObject)classInstance.Invoke("ReceiveByCorrelationId", correlationId, timeout == null ? null : timeout.getJCOInstance(), transactionType == null ? null : transactionType.getJCOInstance());
return new Message(objReceiveByCorrelationId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveById(java.lang.String id) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveById = (JCObject)classInstance.Invoke("ReceiveById", id);
return new Message(objReceiveById);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveById(java.lang.String id, MessageQueueTransaction transaction) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveById = (JCObject)classInstance.Invoke("ReceiveById", id, transaction == null ? null : transaction.getJCOInstance());
return new Message(objReceiveById);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveById(java.lang.String id, MessageQueueTransactionType transactionType) throws Throwable, system.ArgumentNullException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveById = (JCObject)classInstance.Invoke("ReceiveById", id, transactionType == null ? null : transactionType.getJCOInstance());
return new Message(objReceiveById);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveById(java.lang.String id, TimeSpan timeout) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveById = (JCObject)classInstance.Invoke("ReceiveById", id, timeout == null ? null : timeout.getJCOInstance());
return new Message(objReceiveById);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveById(java.lang.String id, TimeSpan timeout, MessageQueueTransaction transaction) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveById = (JCObject)classInstance.Invoke("ReceiveById", id, timeout == null ? null : timeout.getJCOInstance(), transaction == null ? null : transaction.getJCOInstance());
return new Message(objReceiveById);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveById(java.lang.String id, TimeSpan timeout, MessageQueueTransactionType transactionType) throws Throwable, system.ArgumentNullException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveById = (JCObject)classInstance.Invoke("ReceiveById", id, timeout == null ? null : timeout.getJCOInstance(), transactionType == null ? null : transactionType.getJCOInstance());
return new Message(objReceiveById);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveByLookupId(long lookupId) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.PlatformNotSupportedException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveByLookupId = (JCObject)classInstance.Invoke("ReceiveByLookupId", lookupId);
return new Message(objReceiveByLookupId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveByLookupId(MessageLookupAction action, long lookupId, MessageQueueTransaction transaction) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.PlatformNotSupportedException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveByLookupId = (JCObject)classInstance.Invoke("ReceiveByLookupId", action == null ? null : action.getJCOInstance(), lookupId, transaction == null ? null : transaction.getJCOInstance());
return new Message(objReceiveByLookupId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message ReceiveByLookupId(MessageLookupAction action, long lookupId, MessageQueueTransactionType transactionType) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.PlatformNotSupportedException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objReceiveByLookupId = (JCObject)classInstance.Invoke("ReceiveByLookupId", action == null ? null : action.getJCOInstance(), lookupId, transactionType == null ? null : transactionType.getJCOInstance());
return new Message(objReceiveByLookupId);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Message[] GetAllMessages() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classInstance.Invoke("GetAllMessages");
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(new Message(resultingObject));
}
Message[] resultingArray = new Message[resultingArrayList.size()];
resultingArray = resultingArrayList.toArray(resultingArray);
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public MessageEnumerator GetMessageEnumerator() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.collections.generic.KeyNotFoundException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objGetMessageEnumerator = (JCObject)classInstance.Invoke("GetMessageEnumerator");
return new MessageEnumerator(objGetMessageEnumerator);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public MessageEnumerator GetMessageEnumerator2() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.collections.generic.KeyNotFoundException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objGetMessageEnumerator2 = (JCObject)classInstance.Invoke("GetMessageEnumerator2");
return new MessageEnumerator(objGetMessageEnumerator2);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MessageQueue Create(java.lang.String path) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", path);
return new MessageQueue(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MessageQueue Create(java.lang.String path, boolean transactional) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", path, transactional);
return new MessageQueue(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MessageQueue[] GetPrivateQueuesByMachine(java.lang.String machineName) throws Throwable, system.NullReferenceException, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.AccessViolationException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classType.Invoke("GetPrivateQueuesByMachine", machineName);
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(new MessageQueue(resultingObject));
}
MessageQueue[] resultingArray = new MessageQueue[resultingArrayList.size()];
resultingArray = resultingArrayList.toArray(resultingArray);
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MessageQueue[] GetPublicQueues() throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classType.Invoke("GetPublicQueues");
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(new MessageQueue(resultingObject));
}
MessageQueue[] resultingArray = new MessageQueue[resultingArrayList.size()];
resultingArray = resultingArrayList.toArray(resultingArray);
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MessageQueue[] GetPublicQueues(MessageQueueCriteria criteria) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classType.Invoke("GetPublicQueues", criteria == null ? null : criteria.getJCOInstance());
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(new MessageQueue(resultingObject));
}
MessageQueue[] resultingArray = new MessageQueue[resultingArrayList.size()];
resultingArray = resultingArrayList.toArray(resultingArray);
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MessageQueue[] GetPublicQueuesByCategory(Guid category) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classType.Invoke("GetPublicQueuesByCategory", category == null ? null : category.getJCOInstance());
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(new MessageQueue(resultingObject));
}
MessageQueue[] resultingArray = new MessageQueue[resultingArrayList.size()];
resultingArray = resultingArrayList.toArray(resultingArray);
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MessageQueue[] GetPublicQueuesByLabel(java.lang.String label) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classType.Invoke("GetPublicQueuesByLabel", label);
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(new MessageQueue(resultingObject));
}
MessageQueue[] resultingArray = new MessageQueue[resultingArrayList.size()];
resultingArray = resultingArrayList.toArray(resultingArray);
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MessageQueue[] GetPublicQueuesByMachine(java.lang.String machineName) throws Throwable, system.NullReferenceException, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.security.SecurityException, system.componentmodel.InvalidEnumArgumentException, system.OutOfMemoryException, system.AccessViolationException, system.security.cryptography.CryptographicException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classType.Invoke("GetPublicQueuesByMachine", machineName);
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(new MessageQueue(resultingObject));
}
MessageQueue[] resultingArray = new MessageQueue[resultingArrayList.size()];
resultingArray = resultingArrayList.toArray(resultingArray);
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MessageQueueEnumerator GetMessageQueueEnumerator() throws Throwable {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objGetMessageQueueEnumerator = (JCObject)classType.Invoke("GetMessageQueueEnumerator");
return new MessageQueueEnumerator(objGetMessageQueueEnumerator);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MessageQueueEnumerator GetMessageQueueEnumerator(MessageQueueCriteria criteria) throws Throwable {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objGetMessageQueueEnumerator = (JCObject)classType.Invoke("GetMessageQueueEnumerator", criteria == null ? null : criteria.getJCOInstance());
return new MessageQueueEnumerator(objGetMessageQueueEnumerator);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static SecurityContext GetSecurityContext() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.messaging.MessageQueueException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objGetSecurityContext = (JCObject)classType.Invoke("GetSecurityContext");
return new SecurityContext(objGetSecurityContext);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static void ClearConnectionCache() throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentException, system.ArgumentNullException, system.InvalidOperationException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
classType.Invoke("ClearConnectionCache");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Close() throws Throwable, system.ArgumentException, system.ArgumentNullException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Close");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static void Delete(java.lang.String path) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
classType.Invoke("Delete", path);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Purge() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.collections.generic.KeyNotFoundException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Purge");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Refresh() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Refresh");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void ResetPermissions() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.collections.generic.KeyNotFoundException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("ResetPermissions");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Send(NetObject obj) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Send", obj == null ? null : obj.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Send(NetObject obj, MessageQueueTransaction transaction) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Send", obj == null ? null : obj.getJCOInstance(), transaction == null ? null : transaction.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Send(NetObject obj, MessageQueueTransactionType transactionType) throws Throwable, system.ArgumentNullException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.configuration.ConfigurationErrorsException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Send", obj == null ? null : obj.getJCOInstance(), transactionType == null ? null : transactionType.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Send(NetObject obj, java.lang.String label) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.collections.generic.KeyNotFoundException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.NullReferenceException, system.OutOfMemoryException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Send", obj == null ? null : obj.getJCOInstance(), label);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Send(NetObject obj, java.lang.String label, MessageQueueTransaction transaction) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.collections.generic.KeyNotFoundException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.NullReferenceException, system.OutOfMemoryException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Send", obj == null ? null : obj.getJCOInstance(), label, transaction == null ? null : transaction.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Send(NetObject obj, java.lang.String label, MessageQueueTransactionType transactionType) throws Throwable, system.ArgumentNullException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.PlatformNotSupportedException, system.transactions.TransactionPromotionException, system.transactions.TransactionException, system.transactions.TransactionInDoubtException, system.transactions.TransactionManagerCommunicationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Send", obj == null ? null : obj.getJCOInstance(), label, transactionType == null ? null : transactionType.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void SetPermissions(AccessControlList dacl) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.collections.generic.KeyNotFoundException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.NullReferenceException, system.security.SecurityException, system.PlatformNotSupportedException, system.componentmodel.Win32Exception, system.OutOfMemoryException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("SetPermissions", dacl == null ? null : dacl.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void SetPermissions(MessageQueueAccessControlEntry ace) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException, system.security.SecurityException, system.PlatformNotSupportedException, system.componentmodel.Win32Exception, system.OutOfMemoryException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("SetPermissions", ace == null ? null : ace.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void SetPermissions(java.lang.String user, MessageQueueAccessRights rights) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.IndexOutOfRangeException, system.componentmodel.InvalidEnumArgumentException, system.ArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.OutOfMemoryException, system.security.SecurityException, system.PlatformNotSupportedException, system.componentmodel.Win32Exception {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("SetPermissions", user, rights == null ? null : rights.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void SetPermissions(java.lang.String user, MessageQueueAccessRights rights, AccessControlEntryType entryType) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.IndexOutOfRangeException, system.componentmodel.InvalidEnumArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException, system.OutOfMemoryException, system.security.SecurityException, system.PlatformNotSupportedException, system.componentmodel.Win32Exception {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("SetPermissions", user, rights == null ? null : rights.getJCOInstance(), entryType == null ? null : entryType.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
// Properties section
public boolean getAuthenticate() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("Authenticate");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setAuthenticate(boolean Authenticate) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Authenticate", Authenticate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getCanRead() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.collections.generic.KeyNotFoundException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("CanRead");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getCanWrite() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.collections.generic.KeyNotFoundException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("CanWrite");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getDenySharedReceive() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("DenySharedReceive");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setDenySharedReceive(boolean DenySharedReceive) throws Throwable, system.ArgumentException, system.ArgumentNullException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("DenySharedReceive", DenySharedReceive);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static boolean getEnableConnectionCache() throws Throwable {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (boolean)classType.Get("EnableConnectionCache");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static void setEnableConnectionCache(boolean EnableConnectionCache) throws Throwable {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
classType.Set("EnableConnectionCache", EnableConnectionCache);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getTransactional() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.collections.generic.KeyNotFoundException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("Transactional");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getUseJournalQueue() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("UseJournalQueue");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setUseJournalQueue(boolean UseJournalQueue) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("UseJournalQueue", UseJournalQueue);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public short getBasePriority() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (short)classInstance.Get("BasePriority");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setBasePriority(short BasePriority) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("BasePriority", BasePriority);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public long getMaximumJournalSize() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (long)classInstance.Get("MaximumJournalSize");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setMaximumJournalSize(long MaximumJournalSize) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("MaximumJournalSize", MaximumJournalSize);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public long getMaximumQueueSize() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (long)classInstance.Get("MaximumQueueSize");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setMaximumQueueSize(long MaximumQueueSize) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("MaximumQueueSize", MaximumQueueSize);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public ISynchronizeInvoke getSynchronizingObject() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("SynchronizingObject");
return new ISynchronizeInvokeImplementation(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setSynchronizingObject(ISynchronizeInvoke SynchronizingObject) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("SynchronizingObject", SynchronizingObject == null ? null : SynchronizingObject.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public DateTime getCreateTime() throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException, system.InvalidTimeZoneException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("CreateTime");
return new DateTime(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public DateTime getLastModifyTime() throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException, system.InvalidTimeZoneException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("LastModifyTime");
return new DateTime(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Guid getCategory() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("Category");
return new Guid(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setCategory(Guid Category) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Category", Category == null ? null : Category.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Guid getId() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("Id");
return new Guid(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public DefaultPropertiesToSend getDefaultPropertiesToSend() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("DefaultPropertiesToSend");
return new DefaultPropertiesToSend(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setDefaultPropertiesToSend(DefaultPropertiesToSend DefaultPropertiesToSend) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("DefaultPropertiesToSend", DefaultPropertiesToSend == null ? null : DefaultPropertiesToSend.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public EncryptionRequired getEncryptionRequired() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("EncryptionRequired");
return new EncryptionRequired(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setEncryptionRequired(EncryptionRequired EncryptionRequired) throws Throwable, system.ArgumentNullException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("EncryptionRequired", EncryptionRequired == null ? null : EncryptionRequired.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IMessageFormatter getFormatter() throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ArgumentException, system.FormatException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("Formatter");
return new IMessageFormatterImplementation(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setFormatter(IMessageFormatter Formatter) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Formatter", Formatter == null ? null : Formatter.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public MessagePropertyFilter getMessageReadPropertyFilter() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("MessageReadPropertyFilter");
return new MessagePropertyFilter(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setMessageReadPropertyFilter(MessagePropertyFilter MessageReadPropertyFilter) throws Throwable, system.ArgumentNullException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("MessageReadPropertyFilter", MessageReadPropertyFilter == null ? null : MessageReadPropertyFilter.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public QueueAccessMode getAccessMode() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("AccessMode");
return new QueueAccessMode(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String getFormatName() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.collections.generic.KeyNotFoundException, system.FormatException, system.NotImplementedException, system.InvalidOperationException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.messaging.MessageQueueException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Get("FormatName");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String getLabel() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Get("Label");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setLabel(java.lang.String Label) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.IndexOutOfRangeException, system.collections.generic.KeyNotFoundException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Label", Label);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String getMachineName() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Get("MachineName");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setMachineName(java.lang.String MachineName) throws Throwable, system.ArgumentNullException, system.NullReferenceException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("MachineName", MachineName);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String getMulticastAddress() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.PlatformNotSupportedException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Get("MulticastAddress");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setMulticastAddress(java.lang.String MulticastAddress) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.PlatformNotSupportedException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.FormatException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("MulticastAddress", MulticastAddress);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String getPath() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Get("Path");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setPath(java.lang.String Path) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.threading.AbandonedMutexException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Path", Path);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String getQueueName() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.globalization.CultureNotFoundException, system.NotSupportedException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Get("QueueName");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setQueueName(java.lang.String QueueName) throws Throwable, system.ArgumentNullException, system.FormatException, system.ArgumentOutOfRangeException, system.ArgumentException, system.IndexOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.collections.generic.KeyNotFoundException, system.messaging.MessageQueueException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("QueueName", QueueName);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
// Instance Events section
public void addPeekCompleted(PeekCompletedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PeekCompleted", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePeekCompleted(PeekCompletedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PeekCompleted", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addReceiveCompleted(ReceiveCompletedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("ReceiveCompleted", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeReceiveCompleted(ReceiveCompletedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("ReceiveCompleted", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
}