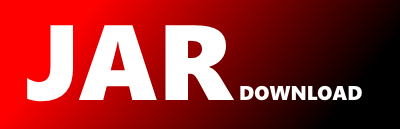
system.text.regularexpressions.Regex Maven / Gradle / Ivy
/*
* MIT License
*
* Copyright (c) 2024 MASES s.r.l.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**************************************************************************************
*
* This code was generated from a template using JCOReflector v. 1.14.0.0
*
* Manual changes to this file may cause unexpected behavior in your application.
* Manual changes to this file will be overwritten if the code is regenerated.
*
*************************************************************************************/
package system.text.regularexpressions;
import org.mases.jcobridge.*;
import org.mases.jcobridge.netreflection.*;
import java.util.ArrayList;
// Import section
import system.text.regularexpressions.RegexOptions;
import system.TimeSpan;
import system.text.regularexpressions.MatchEvaluator;
import system.text.regularexpressions.Match;
import system.text.regularexpressions.MatchCollection;
import system.text.regularexpressions.RegexCompilationInfo;
import system.reflection.AssemblyName;
import system.reflection.emit.CustomAttributeBuilder;
import system.runtime.serialization.SerializationInfo;
import system.runtime.serialization.StreamingContext;
import system.runtime.serialization.ISerializable;
import system.runtime.serialization.ISerializableImplementation;
/**
* The base .NET class managing System.Text.RegularExpressions.Regex, System, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089.
*
*
* .NET documentation at https://docs.microsoft.com/en-us/dotnet/api/System.Text.RegularExpressions.Regex
*
*
* Powered by JCOBridge: more info at https://www.jcobridge.com
*
* @author MASES s.r.l https://masesgroup.com
* @version 1.14.0.0
*/
public class Regex extends NetObject implements system.runtime.serialization.ISerializable {
/**
* Fully assembly qualified name: System, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089
*/
public static final String assemblyFullName = "System, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089";
/**
* Assembly name: System
*/
public static final String assemblyShortName = "System";
/**
* Qualified class name: System.Text.RegularExpressions.Regex
*/
public static final String className = "System.Text.RegularExpressions.Regex";
static JCOBridge bridge = JCOBridgeInstance.getInstance(assemblyFullName);
/**
* The type managed from JCOBridge. See {@link JCType}
*/
public static JCType classType = createType();
static JCEnum enumInstance = null;
JCObject classInstance = null;
static JCType createType() {
try {
String classToCreate = className + ", "
+ (JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
if (JCOReflector.getDebug())
JCOReflector.writeLog("Creating %s", classToCreate);
JCType typeCreated = bridge.GetType(classToCreate);
if (JCOReflector.getDebug())
JCOReflector.writeLog("Created: %s",
(typeCreated != null) ? typeCreated.toString() : "Returned null value");
return typeCreated;
} catch (JCException e) {
JCOReflector.writeLog(e);
return null;
}
}
void addReference(String ref) throws Throwable {
try {
bridge.AddReference(ref);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
/**
* Internal constructor. Use with caution
*/
public Regex(java.lang.Object instance) throws Throwable {
super(instance);
if (instance instanceof JCObject) {
classInstance = (JCObject) instance;
} else
throw new Exception("Cannot manage object, it is not a JCObject");
}
public String getJCOAssemblyName() {
return assemblyFullName;
}
public String getJCOClassName() {
return className;
}
public String getJCOObjectName() {
return className + ", " + (JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
}
public java.lang.Object getJCOInstance() {
return classInstance;
}
public void setJCOInstance(JCObject instance) {
classInstance = instance;
super.setJCOInstance(classInstance);
}
public JCType getJCOType() {
return classType;
}
/**
* Try to cast the {@link IJCOBridgeReflected} instance into {@link Regex}, a cast assert is made to check if types are compatible.
* @param from {@link IJCOBridgeReflected} instance to be casted
* @return {@link Regex} instance
* @throws java.lang.Throwable in case of error during cast operation
*/
public static Regex cast(IJCOBridgeReflected from) throws Throwable {
NetType.AssertCast(classType, from);
return new Regex(from.getJCOInstance());
}
// Constructors section
public Regex() throws Throwable {
}
public Regex(java.lang.String pattern) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.FormatException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.RankException, system.security.SecurityException {
try {
// add reference to assemblyName.dll file
addReference(JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
setJCOInstance((JCObject)classType.NewObject(pattern));
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Regex(java.lang.String pattern, RegexOptions options) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.FormatException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.RankException, system.security.SecurityException {
try {
// add reference to assemblyName.dll file
addReference(JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
setJCOInstance((JCObject)classType.NewObject(pattern, options == null ? null : options.getJCOInstance()));
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Regex(java.lang.String pattern, RegexOptions options, TimeSpan matchTimeout) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.FormatException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.RankException, system.security.SecurityException {
try {
// add reference to assemblyName.dll file
addReference(JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
setJCOInstance((JCObject)classType.NewObject(pattern, options == null ? null : options.getJCOInstance(), matchTimeout == null ? null : matchTimeout.getJCOInstance()));
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
// Methods section
public boolean IsMatch(java.lang.String input) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Invoke("IsMatch", input);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean IsMatch(java.lang.String input, int startat) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Invoke("IsMatch", input, startat);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static boolean IsMatch(java.lang.String input, java.lang.String pattern) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (boolean)classType.Invoke("IsMatch", input, pattern);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static boolean IsMatch(java.lang.String input, java.lang.String pattern, RegexOptions options) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (boolean)classType.Invoke("IsMatch", input, pattern, options == null ? null : options.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static boolean IsMatch(java.lang.String input, java.lang.String pattern, RegexOptions options, TimeSpan matchTimeout) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.FormatException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.RankException, system.security.SecurityException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (boolean)classType.Invoke("IsMatch", input, pattern, options == null ? null : options.getJCOInstance(), matchTimeout == null ? null : matchTimeout.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public int GroupNumberFromName(java.lang.String name) throws Throwable, system.ArgumentNullException, system.ObjectDisposedException, system.threading.AbandonedMutexException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (int)classInstance.Invoke("GroupNumberFromName", name);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public int[] GetGroupNumbers() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classInstance.Invoke("GetGroupNumbers");
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(resultingObject);
}
int[] resultingArray = new int[resultingArrayList.size()];
for(int indexGetGroupNumbers = 0; indexGetGroupNumbers < resultingArrayList.size(); indexGetGroupNumbers++ ) {
resultingArray[indexGetGroupNumbers] = (int)resultingArrayList.get(indexGetGroupNumbers);
}
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static java.lang.String Escape(java.lang.String str) throws Throwable, system.ArgumentNullException, system.FormatException, system.ArgumentOutOfRangeException, system.InvalidOperationException, system.OutOfMemoryException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (java.lang.String)classType.Invoke("Escape", str);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String GroupNameFromNumber(int i) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.ObjectDisposedException, system.threading.AbandonedMutexException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Invoke("GroupNameFromNumber", i);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String Replace(java.lang.String input, java.lang.String replacement) throws Throwable, system.ArgumentNullException, system.NullReferenceException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotSupportedException, system.OutOfMemoryException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.FormatException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Invoke("Replace", input, replacement);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String Replace(java.lang.String input, java.lang.String replacement, int count) throws Throwable, system.ArgumentNullException, system.NullReferenceException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.NotSupportedException, system.OutOfMemoryException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.FormatException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Invoke("Replace", input, replacement, count);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String Replace(java.lang.String input, java.lang.String replacement, int count, int startat) throws Throwable, system.ArgumentNullException, system.NullReferenceException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.ArgumentOutOfRangeException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Invoke("Replace", input, replacement, count, startat);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static java.lang.String Replace(java.lang.String input, java.lang.String pattern, java.lang.String replacement) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (java.lang.String)classType.Invoke("Replace", input, pattern, replacement);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static java.lang.String Replace(java.lang.String input, java.lang.String pattern, java.lang.String replacement, RegexOptions options) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (java.lang.String)classType.Invoke("Replace", input, pattern, replacement, options == null ? null : options.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static java.lang.String Replace(java.lang.String input, java.lang.String pattern, java.lang.String replacement, RegexOptions options, TimeSpan matchTimeout) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.FormatException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.RankException, system.security.SecurityException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (java.lang.String)classType.Invoke("Replace", input, pattern, replacement, options == null ? null : options.getJCOInstance(), matchTimeout == null ? null : matchTimeout.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static java.lang.String Replace(java.lang.String input, java.lang.String pattern, MatchEvaluator evaluator) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (java.lang.String)classType.Invoke("Replace", input, pattern, evaluator);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static java.lang.String Replace(java.lang.String input, java.lang.String pattern, MatchEvaluator evaluator, RegexOptions options) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (java.lang.String)classType.Invoke("Replace", input, pattern, evaluator, options == null ? null : options.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static java.lang.String Replace(java.lang.String input, java.lang.String pattern, MatchEvaluator evaluator, RegexOptions options, TimeSpan matchTimeout) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.FormatException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.RankException, system.security.SecurityException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (java.lang.String)classType.Invoke("Replace", input, pattern, evaluator, options == null ? null : options.getJCOInstance(), matchTimeout == null ? null : matchTimeout.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String Replace(java.lang.String input, MatchEvaluator evaluator) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException, system.OutOfMemoryException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Invoke("Replace", input, evaluator);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String Replace(java.lang.String input, MatchEvaluator evaluator, int count) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException, system.OutOfMemoryException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Invoke("Replace", input, evaluator, count);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String Replace(java.lang.String input, MatchEvaluator evaluator, int count, int startat) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Invoke("Replace", input, evaluator, count, startat);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static java.lang.String Unescape(java.lang.String str) throws Throwable, system.ArgumentNullException, system.FormatException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.OutOfMemoryException, system.NotImplementedException, system.resources.MissingManifestResourceException, system.ObjectDisposedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (java.lang.String)classType.Invoke("Unescape", str);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String[] GetGroupNames() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classInstance.Invoke("GetGroupNames");
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(resultingObject);
}
java.lang.String[] resultingArray = new java.lang.String[resultingArrayList.size()];
for(int indexGetGroupNames = 0; indexGetGroupNames < resultingArrayList.size(); indexGetGroupNames++ ) {
resultingArray[indexGetGroupNames] = (java.lang.String)resultingArrayList.get(indexGetGroupNames);
}
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String[] Split(java.lang.String input) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException, system.RankException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classInstance.Invoke("Split", input);
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(resultingObject);
}
java.lang.String[] resultingArray = new java.lang.String[resultingArrayList.size()];
for(int indexSplit = 0; indexSplit < resultingArrayList.size(); indexSplit++ ) {
resultingArray[indexSplit] = (java.lang.String)resultingArrayList.get(indexSplit);
}
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String[] Split(java.lang.String input, int count) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException, system.RankException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classInstance.Invoke("Split", input, count);
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(resultingObject);
}
java.lang.String[] resultingArray = new java.lang.String[resultingArrayList.size()];
for(int indexSplit = 0; indexSplit < resultingArrayList.size(); indexSplit++ ) {
resultingArray[indexSplit] = (java.lang.String)resultingArrayList.get(indexSplit);
}
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String[] Split(java.lang.String input, int count, int startat) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException, system.RankException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classInstance.Invoke("Split", input, count, startat);
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(resultingObject);
}
java.lang.String[] resultingArray = new java.lang.String[resultingArrayList.size()];
for(int indexSplit = 0; indexSplit < resultingArrayList.size(); indexSplit++ ) {
resultingArray[indexSplit] = (java.lang.String)resultingArrayList.get(indexSplit);
}
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static java.lang.String[] Split(java.lang.String input, java.lang.String pattern) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classType.Invoke("Split", input, pattern);
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(resultingObject);
}
java.lang.String[] resultingArray = new java.lang.String[resultingArrayList.size()];
for(int indexSplit = 0; indexSplit < resultingArrayList.size(); indexSplit++ ) {
resultingArray[indexSplit] = (java.lang.String)resultingArrayList.get(indexSplit);
}
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static java.lang.String[] Split(java.lang.String input, java.lang.String pattern, RegexOptions options) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classType.Invoke("Split", input, pattern, options == null ? null : options.getJCOInstance());
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(resultingObject);
}
java.lang.String[] resultingArray = new java.lang.String[resultingArrayList.size()];
for(int indexSplit = 0; indexSplit < resultingArrayList.size(); indexSplit++ ) {
resultingArray[indexSplit] = (java.lang.String)resultingArrayList.get(indexSplit);
}
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static java.lang.String[] Split(java.lang.String input, java.lang.String pattern, RegexOptions options, TimeSpan matchTimeout) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.FormatException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.RankException, system.security.SecurityException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
ArrayList resultingArrayList = new ArrayList();
JCObject resultingObjects = (JCObject)classType.Invoke("Split", input, pattern, options == null ? null : options.getJCOInstance(), matchTimeout == null ? null : matchTimeout.getJCOInstance());
for (java.lang.Object resultingObject : resultingObjects) {
resultingArrayList.add(resultingObject);
}
java.lang.String[] resultingArray = new java.lang.String[resultingArrayList.size()];
for(int indexSplit = 0; indexSplit < resultingArrayList.size(); indexSplit++ ) {
resultingArray[indexSplit] = (java.lang.String)resultingArrayList.get(indexSplit);
}
return resultingArray;
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Match Match(java.lang.String input) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objMatch = (JCObject)classInstance.Invoke("Match", input);
return new Match(objMatch);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Match Match(java.lang.String input, int startat) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objMatch = (JCObject)classInstance.Invoke("Match", input, startat);
return new Match(objMatch);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Match Match(java.lang.String input, int beginning, int length) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objMatch = (JCObject)classInstance.Invoke("Match", input, beginning, length);
return new Match(objMatch);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static Match Match(java.lang.String input, java.lang.String pattern) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objMatch = (JCObject)classType.Invoke("Match", input, pattern);
return new Match(objMatch);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static Match Match(java.lang.String input, java.lang.String pattern, RegexOptions options) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objMatch = (JCObject)classType.Invoke("Match", input, pattern, options == null ? null : options.getJCOInstance());
return new Match(objMatch);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static Match Match(java.lang.String input, java.lang.String pattern, RegexOptions options, TimeSpan matchTimeout) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.FormatException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.RankException, system.security.SecurityException, system.OverflowException, system.text.regularexpressions.RegexMatchTimeoutException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objMatch = (JCObject)classType.Invoke("Match", input, pattern, options == null ? null : options.getJCOInstance(), matchTimeout == null ? null : matchTimeout.getJCOInstance());
return new Match(objMatch);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public MatchCollection Matches(java.lang.String input) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objMatches = (JCObject)classInstance.Invoke("Matches", input);
return new MatchCollection(objMatches);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public MatchCollection Matches(java.lang.String input, int startat) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objMatches = (JCObject)classInstance.Invoke("Matches", input, startat);
return new MatchCollection(objMatches);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MatchCollection Matches(java.lang.String input, java.lang.String pattern) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objMatches = (JCObject)classType.Invoke("Matches", input, pattern);
return new MatchCollection(objMatches);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MatchCollection Matches(java.lang.String input, java.lang.String pattern, RegexOptions options) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.NotSupportedException, system.RankException, system.security.SecurityException, system.NotImplementedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objMatches = (JCObject)classType.Invoke("Matches", input, pattern, options == null ? null : options.getJCOInstance());
return new MatchCollection(objMatches);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static MatchCollection Matches(java.lang.String input, java.lang.String pattern, RegexOptions options, TimeSpan matchTimeout) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.TypeLoadException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotSupportedException, system.globalization.CultureNotFoundException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.FormatException, system.NullReferenceException, system.collections.generic.KeyNotFoundException, system.RankException, system.security.SecurityException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objMatches = (JCObject)classType.Invoke("Matches", input, pattern, options == null ? null : options.getJCOInstance(), matchTimeout == null ? null : matchTimeout.getJCOInstance());
return new MatchCollection(objMatches);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static void CompileToAssembly(RegexCompilationInfo[] regexinfos, AssemblyName assemblyname) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.security.SecurityException, system.NotSupportedException, system.FormatException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.InvalidOperationException, system.NullReferenceException, system.TypeLoadException, system.MissingMethodException, system.reflection.TargetInvocationException, system.io.FileNotFoundException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.collections.generic.KeyNotFoundException, system.RankException, system.UnauthorizedAccessException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
classType.Invoke("CompileToAssembly", toObjectFromArray(regexinfos), assemblyname == null ? null : assemblyname.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static void CompileToAssembly(RegexCompilationInfo[] regexinfos, AssemblyName assemblyname, CustomAttributeBuilder[] attributes) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.security.SecurityException, system.NotSupportedException, system.FormatException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.InvalidOperationException, system.NullReferenceException, system.TypeLoadException, system.MissingMethodException, system.reflection.TargetInvocationException, system.io.FileNotFoundException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.collections.generic.KeyNotFoundException, system.RankException, system.UnauthorizedAccessException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
classType.Invoke("CompileToAssembly", toObjectFromArray(regexinfos), assemblyname == null ? null : assemblyname.getJCOInstance(), toObjectFromArray(attributes));
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static void CompileToAssembly(RegexCompilationInfo[] regexinfos, AssemblyName assemblyname, CustomAttributeBuilder[] attributes, java.lang.String resourceFile) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.security.SecurityException, system.NotSupportedException, system.FormatException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.InvalidOperationException, system.NullReferenceException, system.TypeLoadException, system.MissingMethodException, system.reflection.TargetInvocationException, system.io.FileNotFoundException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.collections.generic.KeyNotFoundException, system.RankException, system.UnauthorizedAccessException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
classType.Invoke("CompileToAssembly", toObjectFromArray(regexinfos), assemblyname == null ? null : assemblyname.getJCOInstance(), toObjectFromArray(attributes), resourceFile);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
/**
* @deprecated Not for public use because the method is implemented in .NET with an explicit interface.
* Use the static ToISerializable method available in ISerializable to obtain an object with an invocable method
*/
@Deprecated
public void GetObjectData(SerializationInfo info, StreamingContext context) throws Throwable {
throw new UnsupportedOperationException("Not for public use because the method is implemented with an explicit interface. Use ToISerializable to obtain the full interface.");
}
// Properties section
public boolean getRightToLeft() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("RightToLeft");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static int getCacheSize() throws Throwable {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (int)classType.Get("CacheSize");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static void setCacheSize(int CacheSize) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentException, system.ArgumentNullException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
classType.Set("CacheSize", CacheSize);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public RegexOptions getOptions() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("Options");
return new RegexOptions(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public TimeSpan getMatchTimeout() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("MatchTimeout");
return new TimeSpan(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
// Instance Events section
}