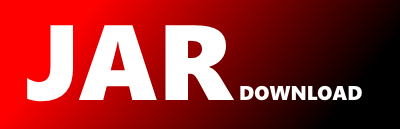
system.windows.UIElement Maven / Gradle / Ivy
/*
* MIT License
*
* Copyright (c) 2024 MASES s.r.l.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**************************************************************************************
*
* This code was generated from a template using JCOReflector v. 1.14.0.0
*
* Manual changes to this file may cause unexpected behavior in your application.
* Manual changes to this file will be overwritten if the code is regenerated.
*
*************************************************************************************/
package system.windows;
import org.mases.jcobridge.*;
import org.mases.jcobridge.netreflection.*;
import java.util.ArrayList;
// Import section
import system.windows.media.Visual;
import system.windows.input.TouchDevice;
import system.windows.input.TraversalRequest;
import system.windows.DependencyProperty;
import system.windows.DependencyObject;
import system.windows.input.FocusNavigationDirection;
import system.windows.IInputElement;
import system.windows.IInputElementImplementation;
import system.windows.Point;
import system.windows.UIElement;
import system.windows.RoutedEvent;
import system.windows.EventRoute;
import system.windows.RoutedEventArgs;
import system.windows.media.animation.AnimationClock;
import system.windows.media.animation.HandoffBehavior;
import system.windows.Rect;
import system.windows.media.animation.AnimationTimeline;
import system.windows.Size;
import system.windows.input.CommandBindingCollection;
import system.windows.input.InputBindingCollection;
import system.windows.media.Brush;
import system.windows.media.CacheMode;
import system.windows.media.effects.BitmapEffect;
import system.windows.media.effects.BitmapEffectInput;
import system.windows.media.effects.Effect;
import system.windows.media.Geometry;
import system.windows.media.Transform;
import system.windows.Visibility;
import system.EventHandler;
import system.windows.DependencyPropertyChangedEventHandler;
import system.windows.DragEventHandler;
import system.windows.GiveFeedbackEventHandler;
import system.windows.input.KeyboardFocusChangedEventHandler;
import system.windows.input.KeyEventHandler;
import system.windows.input.MouseButtonEventHandler;
import system.windows.input.MouseEventHandler;
import system.windows.input.MouseWheelEventHandler;
import system.windows.input.QueryCursorEventHandler;
import system.windows.input.StylusButtonEventHandler;
import system.windows.input.StylusDownEventHandler;
import system.windows.input.StylusEventHandler;
import system.windows.input.StylusSystemGestureEventHandler;
import system.windows.input.TextCompositionEventHandler;
import system.windows.QueryContinueDragEventHandler;
import system.windows.RoutedEventHandler;
/**
* The base .NET class managing System.Windows.UIElement, PresentationCore, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35.
*
*
* .NET documentation at https://docs.microsoft.com/en-us/dotnet/api/System.Windows.UIElement
*
*
* Powered by JCOBridge: more info at https://www.jcobridge.com
*
* @author MASES s.r.l https://masesgroup.com
* @version 1.14.0.0
*/
public class UIElement extends Visual {
/**
* Fully assembly qualified name: PresentationCore, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35
*/
public static final String assemblyFullName = "PresentationCore, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35";
/**
* Assembly name: PresentationCore
*/
public static final String assemblyShortName = "PresentationCore";
/**
* Qualified class name: System.Windows.UIElement
*/
public static final String className = "System.Windows.UIElement";
static JCOBridge bridge = JCOBridgeInstance.getInstance(assemblyFullName);
/**
* The type managed from JCOBridge. See {@link JCType}
*/
public static JCType classType = createType();
static JCEnum enumInstance = null;
JCObject classInstance = null;
static JCType createType() {
try {
String classToCreate = className + ", "
+ (JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
if (JCOReflector.getDebug())
JCOReflector.writeLog("Creating %s", classToCreate);
JCType typeCreated = bridge.GetType(classToCreate);
if (JCOReflector.getDebug())
JCOReflector.writeLog("Created: %s",
(typeCreated != null) ? typeCreated.toString() : "Returned null value");
return typeCreated;
} catch (JCException e) {
JCOReflector.writeLog(e);
return null;
}
}
void addReference(String ref) throws Throwable {
try {
bridge.AddReference(ref);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
/**
* Internal constructor. Use with caution
*/
public UIElement(java.lang.Object instance) throws Throwable {
super(instance);
if (instance instanceof JCObject) {
classInstance = (JCObject) instance;
} else
throw new Exception("Cannot manage object, it is not a JCObject");
}
public String getJCOAssemblyName() {
return assemblyFullName;
}
public String getJCOClassName() {
return className;
}
public String getJCOObjectName() {
return className + ", " + (JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
}
public java.lang.Object getJCOInstance() {
return classInstance;
}
public void setJCOInstance(JCObject instance) {
classInstance = instance;
super.setJCOInstance(classInstance);
}
public JCType getJCOType() {
return classType;
}
/**
* Try to cast the {@link IJCOBridgeReflected} instance into {@link UIElement}, a cast assert is made to check if types are compatible.
* @param from {@link IJCOBridgeReflected} instance to be casted
* @return {@link UIElement} instance
* @throws java.lang.Throwable in case of error during cast operation
*/
public static UIElement cast(IJCOBridgeReflected from) throws Throwable {
NetType.AssertCast(classType, from);
return new UIElement(from.getJCOInstance());
}
// Constructors section
public UIElement() throws Throwable, system.ArgumentException, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.componentmodel.InvalidEnumArgumentException, system.componentmodel.Win32Exception, system.InvalidOperationException, system.NotImplementedException {
try {
// add reference to assemblyName.dll file
addReference(JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
setJCOInstance((JCObject)classType.NewObject());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
// Methods section
public boolean CaptureMouse() throws Throwable, system.ArgumentException, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.componentmodel.InvalidEnumArgumentException, system.componentmodel.Win32Exception, system.InvalidOperationException, system.OverflowException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Invoke("CaptureMouse");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean CaptureStylus() throws Throwable, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.ArgumentException, system.ArgumentNullException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Invoke("CaptureStylus");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean CaptureTouch(TouchDevice touchDevice) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentException, system.ArgumentOutOfRangeException, system.NotSupportedException, system.componentmodel.InvalidEnumArgumentException, system.MulticastNotSupportedException, system.collections.generic.KeyNotFoundException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Invoke("CaptureTouch", touchDevice == null ? null : touchDevice.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean Focus() throws Throwable, system.ArgumentException, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.componentmodel.InvalidEnumArgumentException, system.componentmodel.Win32Exception, system.InvalidOperationException, system.OverflowException, system.PlatformNotSupportedException, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Invoke("Focus");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean MoveFocus(TraversalRequest request) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Invoke("MoveFocus", request == null ? null : request.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean ReleaseTouchCapture(TouchDevice touchDevice) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentException, system.ArgumentOutOfRangeException, system.NotSupportedException, system.componentmodel.InvalidEnumArgumentException, system.MulticastNotSupportedException, system.collections.generic.KeyNotFoundException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Invoke("ReleaseTouchCapture", touchDevice == null ? null : touchDevice.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean ShouldSerializeCommandBindings() throws Throwable, system.ArgumentNullException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Invoke("ShouldSerializeCommandBindings");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean ShouldSerializeInputBindings() throws Throwable, system.ArgumentNullException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Invoke("ShouldSerializeInputBindings");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public NetObject GetAnimationBaseValue(DependencyProperty dp) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.ArgumentOutOfRangeException, system.NotSupportedException, system.InvalidOperationException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.security.SecurityException, system.io.IOException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objGetAnimationBaseValue = (JCObject)classInstance.Invoke("GetAnimationBaseValue", dp == null ? null : dp.getJCOInstance());
return new NetObject(objGetAnimationBaseValue);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public DependencyObject PredictFocus(FocusNavigationDirection direction) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objPredictFocus = (JCObject)classInstance.Invoke("PredictFocus", direction == null ? null : direction.getJCOInstance());
return new DependencyObject(objPredictFocus);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public IInputElement InputHitTest(Point point) throws Throwable, system.InvalidOperationException, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.ArgumentException, system.OverflowException, system.componentmodel.Win32Exception, system.ObjectDisposedException, system.security.SecurityException, system.io.IOException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objInputHitTest = (JCObject)classInstance.Invoke("InputHitTest", point == null ? null : point.getJCOInstance());
return new IInputElementImplementation(objInputHitTest);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Point TranslatePoint(Point point, UIElement relativeTo) throws Throwable, system.ArgumentException, system.ArgumentNullException, system.InvalidOperationException, system.OverflowException, system.componentmodel.Win32Exception, system.ObjectDisposedException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objTranslatePoint = (JCObject)classInstance.Invoke("TranslatePoint", point == null ? null : point.getJCOInstance(), relativeTo == null ? null : relativeTo.getJCOInstance());
return new Point(objTranslatePoint);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void AddToEventRoute(EventRoute route, RoutedEventArgs e) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.ObjectDisposedException, system.threading.AbandonedMutexException, system.ArgumentOutOfRangeException, system.InvalidOperationException, system.resources.MissingManifestResourceException, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("AddToEventRoute", route == null ? null : route.getJCOInstance(), e == null ? null : e.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void ApplyAnimationClock(DependencyProperty dp, AnimationClock clock) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.ArgumentOutOfRangeException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.InvalidOperationException, system.NotSupportedException, system.MulticastNotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("ApplyAnimationClock", dp == null ? null : dp.getJCOInstance(), clock == null ? null : clock.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void ApplyAnimationClock(DependencyProperty dp, AnimationClock clock, HandoffBehavior handoffBehavior) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.ArgumentOutOfRangeException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.threading.AbandonedMutexException, system.NotSupportedException, system.MulticastNotSupportedException, system.windows.media.animation.AnimationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("ApplyAnimationClock", dp == null ? null : dp.getJCOInstance(), clock == null ? null : clock.getJCOInstance(), handoffBehavior == null ? null : handoffBehavior.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Arrange(Rect finalRect) throws Throwable, system.ArgumentException, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.InvalidOperationException, system.componentmodel.InvalidEnumArgumentException, system.componentmodel.Win32Exception, system.IndexOutOfRangeException, system.OutOfMemoryException, system.OverflowException, system.TimeoutException, system.security.SecurityException, system.PlatformNotSupportedException, system.NotSupportedException, system.FormatException, system.MulticastNotSupportedException, system.NotImplementedException, system.ObjectDisposedException, system.threading.AbandonedMutexException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Arrange", finalRect == null ? null : finalRect.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void BeginAnimation(DependencyProperty dp, AnimationTimeline animation) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.ArgumentOutOfRangeException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.InvalidOperationException, system.NotSupportedException, system.MulticastNotSupportedException, system.windows.media.animation.AnimationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("BeginAnimation", dp == null ? null : dp.getJCOInstance(), animation == null ? null : animation.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void BeginAnimation(DependencyProperty dp, AnimationTimeline animation, HandoffBehavior handoffBehavior) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.ArgumentOutOfRangeException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.threading.AbandonedMutexException, system.NotSupportedException, system.componentmodel.Win32Exception, system.windows.media.animation.AnimationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("BeginAnimation", dp == null ? null : dp.getJCOInstance(), animation == null ? null : animation.getJCOInstance(), handoffBehavior == null ? null : handoffBehavior.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void InvalidateArrange() throws Throwable, system.ArgumentException, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.InvalidOperationException, system.componentmodel.InvalidEnumArgumentException, system.componentmodel.Win32Exception, system.IndexOutOfRangeException, system.OutOfMemoryException, system.OverflowException, system.TimeoutException, system.security.SecurityException, system.PlatformNotSupportedException, system.NotSupportedException, system.FormatException, system.MulticastNotSupportedException, system.ObjectDisposedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("InvalidateArrange");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void InvalidateMeasure() throws Throwable, system.ArgumentException, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.InvalidOperationException, system.componentmodel.InvalidEnumArgumentException, system.componentmodel.Win32Exception, system.IndexOutOfRangeException, system.OutOfMemoryException, system.OverflowException, system.TimeoutException, system.security.SecurityException, system.PlatformNotSupportedException, system.NotSupportedException, system.FormatException, system.MulticastNotSupportedException, system.NotImplementedException, system.ObjectDisposedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("InvalidateMeasure");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void InvalidateVisual() throws Throwable, system.ArgumentException, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.componentmodel.InvalidEnumArgumentException, system.InvalidOperationException, system.componentmodel.Win32Exception, system.security.SecurityException, system.FormatException, system.MulticastNotSupportedException, system.ObjectDisposedException, system.OutOfMemoryException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("InvalidateVisual");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Measure(Size availableSize) throws Throwable, system.ArgumentException, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.InvalidOperationException, system.componentmodel.InvalidEnumArgumentException, system.componentmodel.Win32Exception, system.IndexOutOfRangeException, system.OutOfMemoryException, system.OverflowException, system.TimeoutException, system.security.SecurityException, system.PlatformNotSupportedException, system.NotSupportedException, system.FormatException, system.MulticastNotSupportedException, system.NotImplementedException, system.ObjectDisposedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Measure", availableSize == null ? null : availableSize.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void RaiseEvent(RoutedEventArgs e) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.configuration.ConfigurationException, system.configuration.ConfigurationErrorsException, system.resources.MissingManifestResourceException, system.ArgumentOutOfRangeException, system.InvalidCastException, system.OutOfMemoryException, system.InvalidOperationException, system.ObjectDisposedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("RaiseEvent", e == null ? null : e.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void ReleaseAllTouchCaptures() throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.InvalidOperationException, system.ArgumentException, system.componentmodel.InvalidEnumArgumentException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("ReleaseAllTouchCaptures");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void ReleaseMouseCapture() throws Throwable, system.ArgumentException, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.componentmodel.InvalidEnumArgumentException, system.componentmodel.Win32Exception, system.InvalidOperationException, system.OverflowException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("ReleaseMouseCapture");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void ReleaseStylusCapture() throws Throwable, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.ArgumentException, system.ArgumentNullException, system.IndexOutOfRangeException, system.FormatException, system.componentmodel.InvalidEnumArgumentException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("ReleaseStylusCapture");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void UpdateLayout() throws Throwable, system.ArgumentException, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.InvalidOperationException, system.componentmodel.InvalidEnumArgumentException, system.componentmodel.Win32Exception, system.IndexOutOfRangeException, system.OutOfMemoryException, system.OverflowException, system.TimeoutException, system.security.SecurityException, system.PlatformNotSupportedException, system.NotSupportedException, system.FormatException, system.MulticastNotSupportedException, system.NotImplementedException, system.ObjectDisposedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("UpdateLayout");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
// Properties section
public boolean getAllowDrop() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("AllowDrop");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setAllowDrop(boolean AllowDrop) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("AllowDrop", AllowDrop);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getAreAnyTouchesCaptured() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("AreAnyTouchesCaptured");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getAreAnyTouchesCapturedWithin() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("AreAnyTouchesCapturedWithin");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getAreAnyTouchesDirectlyOver() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("AreAnyTouchesDirectlyOver");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getAreAnyTouchesOver() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("AreAnyTouchesOver");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getClipToBounds() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("ClipToBounds");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setClipToBounds(boolean ClipToBounds) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("ClipToBounds", ClipToBounds);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getFocusable() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("Focusable");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setFocusable(boolean Focusable) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Focusable", Focusable);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getHasAnimatedProperties() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("HasAnimatedProperties");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsArrangeValid() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsArrangeValid");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsEnabled() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsEnabled");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setIsEnabled(boolean IsEnabled) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("IsEnabled", IsEnabled);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsFocused() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsFocused");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsHitTestVisible() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsHitTestVisible");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setIsHitTestVisible(boolean IsHitTestVisible) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("IsHitTestVisible", IsHitTestVisible);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsInputMethodEnabled() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsInputMethodEnabled");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsKeyboardFocused() throws Throwable, system.ArgumentException, system.ArgumentNullException, system.componentmodel.Win32Exception, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.OverflowException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsKeyboardFocused");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsKeyboardFocusWithin() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsKeyboardFocusWithin");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsManipulationEnabled() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsManipulationEnabled");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setIsManipulationEnabled(boolean IsManipulationEnabled) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("IsManipulationEnabled", IsManipulationEnabled);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsMeasureValid() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsMeasureValid");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsMouseCaptured() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsMouseCaptured");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsMouseCaptureWithin() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsMouseCaptureWithin");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsMouseDirectlyOver() throws Throwable, system.ArgumentException, system.ArgumentNullException, system.componentmodel.Win32Exception, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.OverflowException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsMouseDirectlyOver");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsMouseOver() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsMouseOver");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsStylusCaptured() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsStylusCaptured");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsStylusCaptureWithin() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsStylusCaptureWithin");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsStylusDirectlyOver() throws Throwable, system.io.IOException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ArgumentException, system.componentmodel.InvalidEnumArgumentException, system.ObjectDisposedException, system.componentmodel.Win32Exception, system.security.SecurityException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsStylusDirectlyOver");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsStylusOver() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsStylusOver");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getIsVisible() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsVisible");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getSnapsToDevicePixels() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("SnapsToDevicePixels");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setSnapsToDevicePixels(boolean SnapsToDevicePixels) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("SnapsToDevicePixels", SnapsToDevicePixels);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public double getOpacity() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (double)classInstance.Get("Opacity");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setOpacity(double Opacity) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Opacity", Opacity);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public int getPersistId() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (int)classInstance.Get("PersistId");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public java.lang.String getUid() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Get("Uid");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setUid(java.lang.String Uid) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Uid", Uid);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public CommandBindingCollection getCommandBindings() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("CommandBindings");
return new CommandBindingCollection(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public InputBindingCollection getInputBindings() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("InputBindings");
return new InputBindingCollection(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Brush getOpacityMask() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("OpacityMask");
return new Brush(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setOpacityMask(Brush OpacityMask) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("OpacityMask", OpacityMask == null ? null : OpacityMask.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public CacheMode getCacheMode() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("CacheMode");
return new CacheMode(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setCacheMode(CacheMode CacheMode) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("CacheMode", CacheMode == null ? null : CacheMode.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public BitmapEffect getBitmapEffect() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("BitmapEffect");
return new BitmapEffect(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setBitmapEffect(BitmapEffect BitmapEffect) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("BitmapEffect", BitmapEffect == null ? null : BitmapEffect.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public BitmapEffectInput getBitmapEffectInput() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("BitmapEffectInput");
return new BitmapEffectInput(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setBitmapEffectInput(BitmapEffectInput BitmapEffectInput) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("BitmapEffectInput", BitmapEffectInput == null ? null : BitmapEffectInput.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Effect getEffect() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("Effect");
return new Effect(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setEffect(Effect Effect) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Effect", Effect == null ? null : Effect.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Geometry getClip() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("Clip");
return new Geometry(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setClip(Geometry Clip) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Clip", Clip == null ? null : Clip.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Transform getRenderTransform() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("RenderTransform");
return new Transform(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setRenderTransform(Transform RenderTransform) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("RenderTransform", RenderTransform == null ? null : RenderTransform.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Point getRenderTransformOrigin() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("RenderTransformOrigin");
return new Point(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setRenderTransformOrigin(Point RenderTransformOrigin) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("RenderTransformOrigin", RenderTransformOrigin == null ? null : RenderTransformOrigin.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Size getDesiredSize() throws Throwable, system.ArgumentNullException, system.ArgumentException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("DesiredSize");
return new Size(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Size getRenderSize() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("RenderSize");
return new Size(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setRenderSize(Size RenderSize) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.InvalidOperationException, system.ArgumentException, system.OverflowException, system.IndexOutOfRangeException, system.ArgumentOutOfRangeException, system.componentmodel.Win32Exception, system.security.SecurityException, system.ObjectDisposedException, system.OutOfMemoryException, system.MulticastNotSupportedException, system.InvalidCastException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("RenderSize", RenderSize == null ? null : RenderSize.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Visibility getVisibility() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("Visibility");
return new Visibility(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setVisibility(Visibility Visibility) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Visibility", Visibility == null ? null : Visibility.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
// Instance Events section
public void addLayoutUpdated(EventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("LayoutUpdated", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeLayoutUpdated(EventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("LayoutUpdated", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addFocusableChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("FocusableChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeFocusableChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("FocusableChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addIsEnabledChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("IsEnabledChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeIsEnabledChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("IsEnabledChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addIsHitTestVisibleChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("IsHitTestVisibleChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeIsHitTestVisibleChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("IsHitTestVisibleChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addIsKeyboardFocusedChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("IsKeyboardFocusedChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeIsKeyboardFocusedChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("IsKeyboardFocusedChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addIsKeyboardFocusWithinChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("IsKeyboardFocusWithinChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeIsKeyboardFocusWithinChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("IsKeyboardFocusWithinChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addIsMouseCapturedChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("IsMouseCapturedChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeIsMouseCapturedChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("IsMouseCapturedChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addIsMouseCaptureWithinChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("IsMouseCaptureWithinChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeIsMouseCaptureWithinChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("IsMouseCaptureWithinChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addIsMouseDirectlyOverChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("IsMouseDirectlyOverChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeIsMouseDirectlyOverChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("IsMouseDirectlyOverChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addIsStylusCapturedChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("IsStylusCapturedChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeIsStylusCapturedChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("IsStylusCapturedChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addIsStylusCaptureWithinChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("IsStylusCaptureWithinChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeIsStylusCaptureWithinChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("IsStylusCaptureWithinChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addIsStylusDirectlyOverChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("IsStylusDirectlyOverChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeIsStylusDirectlyOverChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("IsStylusDirectlyOverChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addIsVisibleChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("IsVisibleChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeIsVisibleChanged(DependencyPropertyChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("IsVisibleChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addDragEnter(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("DragEnter", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeDragEnter(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("DragEnter", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addDragLeave(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("DragLeave", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeDragLeave(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("DragLeave", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addDragOver(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("DragOver", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeDragOver(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("DragOver", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addDrop(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("Drop", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeDrop(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("Drop", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewDragEnter(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewDragEnter", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewDragEnter(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewDragEnter", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewDragLeave(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewDragLeave", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewDragLeave(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewDragLeave", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewDragOver(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewDragOver", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewDragOver(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewDragOver", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewDrop(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewDrop", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewDrop(DragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewDrop", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addGiveFeedback(GiveFeedbackEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("GiveFeedback", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeGiveFeedback(GiveFeedbackEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("GiveFeedback", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewGiveFeedback(GiveFeedbackEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewGiveFeedback", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewGiveFeedback(GiveFeedbackEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewGiveFeedback", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addGotKeyboardFocus(KeyboardFocusChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("GotKeyboardFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeGotKeyboardFocus(KeyboardFocusChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("GotKeyboardFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addLostKeyboardFocus(KeyboardFocusChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("LostKeyboardFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeLostKeyboardFocus(KeyboardFocusChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("LostKeyboardFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewGotKeyboardFocus(KeyboardFocusChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewGotKeyboardFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewGotKeyboardFocus(KeyboardFocusChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewGotKeyboardFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewLostKeyboardFocus(KeyboardFocusChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewLostKeyboardFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewLostKeyboardFocus(KeyboardFocusChangedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewLostKeyboardFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addKeyDown(KeyEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("KeyDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeKeyDown(KeyEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("KeyDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addKeyUp(KeyEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("KeyUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeKeyUp(KeyEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("KeyUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewKeyDown(KeyEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewKeyDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewKeyDown(KeyEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewKeyDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewKeyUp(KeyEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewKeyUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewKeyUp(KeyEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewKeyUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addMouseDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("MouseDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeMouseDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("MouseDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addMouseLeftButtonDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("MouseLeftButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeMouseLeftButtonDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("MouseLeftButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addMouseLeftButtonUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("MouseLeftButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeMouseLeftButtonUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("MouseLeftButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addMouseRightButtonDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("MouseRightButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeMouseRightButtonDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("MouseRightButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addMouseRightButtonUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("MouseRightButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeMouseRightButtonUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("MouseRightButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addMouseUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("MouseUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeMouseUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("MouseUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewMouseDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewMouseDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewMouseDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewMouseDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewMouseLeftButtonDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewMouseLeftButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewMouseLeftButtonDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewMouseLeftButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewMouseLeftButtonUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewMouseLeftButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewMouseLeftButtonUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewMouseLeftButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewMouseRightButtonDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewMouseRightButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewMouseRightButtonDown(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewMouseRightButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewMouseRightButtonUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewMouseRightButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewMouseRightButtonUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewMouseRightButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewMouseUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewMouseUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewMouseUp(MouseButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewMouseUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addGotMouseCapture(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("GotMouseCapture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeGotMouseCapture(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("GotMouseCapture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addLostMouseCapture(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("LostMouseCapture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeLostMouseCapture(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("LostMouseCapture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addMouseEnter(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("MouseEnter", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeMouseEnter(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("MouseEnter", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addMouseLeave(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("MouseLeave", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeMouseLeave(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("MouseLeave", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addMouseMove(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("MouseMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeMouseMove(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("MouseMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewMouseMove(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewMouseMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewMouseMove(MouseEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewMouseMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addMouseWheel(MouseWheelEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("MouseWheel", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeMouseWheel(MouseWheelEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("MouseWheel", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewMouseWheel(MouseWheelEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewMouseWheel", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewMouseWheel(MouseWheelEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewMouseWheel", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addQueryCursor(QueryCursorEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("QueryCursor", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeQueryCursor(QueryCursorEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("QueryCursor", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewStylusButtonDown(StylusButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewStylusButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewStylusButtonDown(StylusButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewStylusButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewStylusButtonUp(StylusButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewStylusButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewStylusButtonUp(StylusButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewStylusButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStylusButtonDown(StylusButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StylusButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStylusButtonDown(StylusButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StylusButtonDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStylusButtonUp(StylusButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StylusButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStylusButtonUp(StylusButtonEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StylusButtonUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewStylusDown(StylusDownEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewStylusDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewStylusDown(StylusDownEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewStylusDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStylusDown(StylusDownEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StylusDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStylusDown(StylusDownEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StylusDown", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addGotStylusCapture(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("GotStylusCapture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeGotStylusCapture(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("GotStylusCapture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addLostStylusCapture(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("LostStylusCapture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeLostStylusCapture(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("LostStylusCapture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewStylusInAirMove(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewStylusInAirMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewStylusInAirMove(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewStylusInAirMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewStylusInRange(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewStylusInRange", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewStylusInRange(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewStylusInRange", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewStylusMove(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewStylusMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewStylusMove(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewStylusMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewStylusOutOfRange(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewStylusOutOfRange", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewStylusOutOfRange(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewStylusOutOfRange", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewStylusUp(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewStylusUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewStylusUp(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewStylusUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStylusEnter(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StylusEnter", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStylusEnter(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StylusEnter", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStylusInAirMove(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StylusInAirMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStylusInAirMove(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StylusInAirMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStylusInRange(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StylusInRange", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStylusInRange(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StylusInRange", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStylusLeave(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StylusLeave", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStylusLeave(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StylusLeave", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStylusMove(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StylusMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStylusMove(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StylusMove", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStylusOutOfRange(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StylusOutOfRange", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStylusOutOfRange(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StylusOutOfRange", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStylusUp(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StylusUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStylusUp(StylusEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StylusUp", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewStylusSystemGesture(StylusSystemGestureEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewStylusSystemGesture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewStylusSystemGesture(StylusSystemGestureEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewStylusSystemGesture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStylusSystemGesture(StylusSystemGestureEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StylusSystemGesture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStylusSystemGesture(StylusSystemGestureEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StylusSystemGesture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewTextInput(TextCompositionEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewTextInput", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewTextInput(TextCompositionEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewTextInput", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addTextInput(TextCompositionEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("TextInput", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeTextInput(TextCompositionEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("TextInput", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addPreviewQueryContinueDrag(QueryContinueDragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("PreviewQueryContinueDrag", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removePreviewQueryContinueDrag(QueryContinueDragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("PreviewQueryContinueDrag", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addQueryContinueDrag(QueryContinueDragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("QueryContinueDrag", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeQueryContinueDrag(QueryContinueDragEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("QueryContinueDrag", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addGotFocus(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("GotFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeGotFocus(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("GotFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addLostFocus(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("LostFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeLostFocus(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("LostFocus", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
}