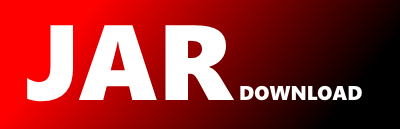
system.windows.controls.InkCanvas Maven / Gradle / Ivy
Show all versions of jcoreflector_net462 Show documentation
/*
* MIT License
*
* Copyright (c) 2024 MASES s.r.l.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**************************************************************************************
*
* This code was generated from a template using JCOReflector v. 1.14.0.0
*
* Manual changes to this file may cause unexpected behavior in your application.
* Manual changes to this file will be overwritten if the code is regenerated.
*
*************************************************************************************/
package system.windows.controls;
import org.mases.jcobridge.*;
import org.mases.jcobridge.netreflection.*;
import java.util.ArrayList;
// Import section
import system.windows.FrameworkElement;
import system.windows.UIElement;
import system.windows.controls.InkCanvasSelectionHitResult;
import system.windows.Point;
import system.windows.ink.StrokeCollection;
import system.windows.Rect;
import system.windows.controls.InkCanvasEditingMode;
import system.windows.controls.UIElementCollection;
import system.windows.ink.DrawingAttributes;
import system.windows.ink.StylusShape;
import system.windows.input.StylusPointDescription;
import system.windows.media.Brush;
import system.EventHandler;
import system.windows.controls.InkCanvasGestureEventHandler;
import system.windows.controls.InkCanvasSelectionChangingEventHandler;
import system.windows.controls.InkCanvasSelectionEditingEventHandler;
import system.windows.controls.InkCanvasStrokeCollectedEventHandler;
import system.windows.controls.InkCanvasStrokeErasingEventHandler;
import system.windows.controls.InkCanvasStrokesReplacedEventHandler;
import system.windows.ink.DrawingAttributesReplacedEventHandler;
import system.windows.RoutedEventHandler;
import system.windows.markup.IAddChild;
import system.windows.markup.IAddChildImplementation;
/**
* The base .NET class managing System.Windows.Controls.InkCanvas, PresentationFramework, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35.
*
*
* .NET documentation at https://docs.microsoft.com/en-us/dotnet/api/System.Windows.Controls.InkCanvas
*
*
* Powered by JCOBridge: more info at https://www.jcobridge.com
*
* @author MASES s.r.l https://masesgroup.com
* @version 1.14.0.0
*/
public class InkCanvas extends FrameworkElement implements system.windows.markup.IAddChild {
/**
* Fully assembly qualified name: PresentationFramework, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35
*/
public static final String assemblyFullName = "PresentationFramework, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35";
/**
* Assembly name: PresentationFramework
*/
public static final String assemblyShortName = "PresentationFramework";
/**
* Qualified class name: System.Windows.Controls.InkCanvas
*/
public static final String className = "System.Windows.Controls.InkCanvas";
static JCOBridge bridge = JCOBridgeInstance.getInstance(assemblyFullName);
/**
* The type managed from JCOBridge. See {@link JCType}
*/
public static JCType classType = createType();
static JCEnum enumInstance = null;
JCObject classInstance = null;
static JCType createType() {
try {
String classToCreate = className + ", "
+ (JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
if (JCOReflector.getDebug())
JCOReflector.writeLog("Creating %s", classToCreate);
JCType typeCreated = bridge.GetType(classToCreate);
if (JCOReflector.getDebug())
JCOReflector.writeLog("Created: %s",
(typeCreated != null) ? typeCreated.toString() : "Returned null value");
return typeCreated;
} catch (JCException e) {
JCOReflector.writeLog(e);
return null;
}
}
void addReference(String ref) throws Throwable {
try {
bridge.AddReference(ref);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
/**
* Internal constructor. Use with caution
*/
public InkCanvas(java.lang.Object instance) throws Throwable {
super(instance);
if (instance instanceof JCObject) {
classInstance = (JCObject) instance;
} else
throw new Exception("Cannot manage object, it is not a JCObject");
}
public String getJCOAssemblyName() {
return assemblyFullName;
}
public String getJCOClassName() {
return className;
}
public String getJCOObjectName() {
return className + ", " + (JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
}
public java.lang.Object getJCOInstance() {
return classInstance;
}
public void setJCOInstance(JCObject instance) {
classInstance = instance;
super.setJCOInstance(classInstance);
}
public JCType getJCOType() {
return classType;
}
/**
* Try to cast the {@link IJCOBridgeReflected} instance into {@link InkCanvas}, a cast assert is made to check if types are compatible.
* @param from {@link IJCOBridgeReflected} instance to be casted
* @return {@link InkCanvas} instance
* @throws java.lang.Throwable in case of error during cast operation
*/
public static InkCanvas cast(IJCOBridgeReflected from) throws Throwable {
NetType.AssertCast(classType, from);
return new InkCanvas(from.getJCOInstance());
}
// Constructors section
public InkCanvas() throws Throwable, system.InvalidOperationException, system.ArgumentNullException, system.ArgumentException, system.ArgumentOutOfRangeException, system.NotImplementedException, system.InvalidCastException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.componentmodel.InvalidEnumArgumentException, system.NotSupportedException, system.OutOfMemoryException, system.componentmodel.Win32Exception {
try {
// add reference to assemblyName.dll file
addReference(JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
setJCOInstance((JCObject)classType.NewObject());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
// Methods section
public boolean CanPaste() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.threading.ThreadStateException, system.SystemException, system.MulticastNotSupportedException, system.security.XmlSyntaxException, system.NullReferenceException, system.ArgumentOutOfRangeException, system.deployment.application.InvalidDeploymentException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Invoke("CanPaste");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static double GetBottom(UIElement element) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (double)classType.Invoke("GetBottom", element == null ? null : element.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static double GetLeft(UIElement element) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (double)classType.Invoke("GetLeft", element == null ? null : element.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static double GetRight(UIElement element) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (double)classType.Invoke("GetRight", element == null ? null : element.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static double GetTop(UIElement element) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
return (double)classType.Invoke("GetTop", element == null ? null : element.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public InkCanvasSelectionHitResult HitTestSelection(Point point) throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.configuration.ConfigurationException, system.configuration.ConfigurationErrorsException, system.ArgumentOutOfRangeException, system.OutOfMemoryException, system.IndexOutOfRangeException, system.componentmodel.Win32Exception, system.NotSupportedException, system.componentmodel.InvalidEnumArgumentException, system.xaml.XamlException, system.security.SecurityException, system.windows.markup.XamlParseException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objHitTestSelection = (JCObject)classInstance.Invoke("HitTestSelection", point == null ? null : point.getJCOInstance());
return new InkCanvasSelectionHitResult(objHitTestSelection);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public StrokeCollection GetSelectedStrokes() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.componentmodel.InvalidEnumArgumentException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objGetSelectedStrokes = (JCObject)classInstance.Invoke("GetSelectedStrokes");
return new StrokeCollection(objGetSelectedStrokes);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Rect GetSelectionBounds() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.componentmodel.InvalidEnumArgumentException, system.IndexOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objGetSelectionBounds = (JCObject)classInstance.Invoke("GetSelectionBounds");
return new Rect(objGetSelectionBounds);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void CopySelection() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.componentmodel.InvalidEnumArgumentException, system.InvalidCastException, system.security.SecurityException, system.ArgumentOutOfRangeException, system.NotSupportedException, system.IndexOutOfRangeException, system.componentmodel.Win32Exception, system.threading.ThreadStateException, system.SystemException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("CopySelection");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void CutSelection() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.componentmodel.InvalidEnumArgumentException, system.InvalidCastException, system.security.SecurityException, system.ArgumentOutOfRangeException, system.NotSupportedException, system.IndexOutOfRangeException, system.componentmodel.Win32Exception, system.threading.ThreadStateException, system.SystemException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("CutSelection");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Paste() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.threading.ThreadStateException, system.SystemException, system.MulticastNotSupportedException, system.security.XmlSyntaxException, system.NullReferenceException, system.ArgumentOutOfRangeException, system.deployment.application.InvalidDeploymentException, system.componentmodel.InvalidEnumArgumentException, system.NotSupportedException, system.IndexOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Paste");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Paste(Point point) throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.threading.ThreadStateException, system.SystemException, system.componentmodel.Win32Exception, system.MulticastNotSupportedException, system.security.XmlSyntaxException, system.NotSupportedException, system.NullReferenceException, system.deployment.application.InvalidDeploymentException, system.MemberAccessException, system.componentmodel.InvalidEnumArgumentException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Paste", point == null ? null : point.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Select(StrokeCollection selectedStrokes) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException, system.componentmodel.InvalidEnumArgumentException, system.MulticastNotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Select", selectedStrokes == null ? null : selectedStrokes.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static void SetBottom(UIElement element, double length) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
classType.Invoke("SetBottom", element == null ? null : element.getJCOInstance(), length);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static void SetLeft(UIElement element, double length) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
classType.Invoke("SetLeft", element == null ? null : element.getJCOInstance(), length);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static void SetRight(UIElement element, double length) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
classType.Invoke("SetRight", element == null ? null : element.getJCOInstance(), length);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static void SetTop(UIElement element, double length) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
classType.Invoke("SetTop", element == null ? null : element.getJCOInstance(), length);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
/**
* @deprecated Not for public use because the method is implemented in .NET with an explicit interface.
* Use the static ToIAddChild method available in IAddChild to obtain an object with an invocable method
*/
@Deprecated
public void AddChild(NetObject value) throws Throwable {
throw new UnsupportedOperationException("Not for public use because the method is implemented with an explicit interface. Use ToIAddChild to obtain the full interface.");
}
/**
* @deprecated Not for public use because the method is implemented in .NET with an explicit interface.
* Use the static ToIAddChild method available in IAddChild to obtain an object with an invocable method
*/
@Deprecated
public void AddText(java.lang.String text) throws Throwable {
throw new UnsupportedOperationException("Not for public use because the method is implemented with an explicit interface. Use ToIAddChild to obtain the full interface.");
}
// Properties section
public boolean getIsGestureRecognizerAvailable() throws Throwable, system.ArgumentException, system.ArgumentNullException, system.componentmodel.Win32Exception, system.InvalidOperationException, system.ObjectDisposedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("IsGestureRecognizerAvailable");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getMoveEnabled() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("MoveEnabled");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setMoveEnabled(boolean MoveEnabled) throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("MoveEnabled", MoveEnabled);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getResizeEnabled() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("ResizeEnabled");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setResizeEnabled(boolean ResizeEnabled) throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("ResizeEnabled", ResizeEnabled);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public boolean getUseCustomCursor() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (boolean)classInstance.Get("UseCustomCursor");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setUseCustomCursor(boolean UseCustomCursor) throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.componentmodel.Win32Exception, system.OverflowException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("UseCustomCursor", UseCustomCursor);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public InkCanvasEditingMode getActiveEditingMode() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("ActiveEditingMode");
return new InkCanvasEditingMode(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public InkCanvasEditingMode getEditingMode() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("EditingMode");
return new InkCanvasEditingMode(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setEditingMode(InkCanvasEditingMode EditingMode) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("EditingMode", EditingMode == null ? null : EditingMode.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public InkCanvasEditingMode getEditingModeInverted() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("EditingModeInverted");
return new InkCanvasEditingMode(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setEditingModeInverted(InkCanvasEditingMode EditingModeInverted) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("EditingModeInverted", EditingModeInverted == null ? null : EditingModeInverted.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public UIElementCollection getChildren() throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.InvalidOperationException, system.ArgumentException, system.NotImplementedException, system.componentmodel.InvalidEnumArgumentException, system.InvalidCastException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("Children");
return new UIElementCollection(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public DrawingAttributes getDefaultDrawingAttributes() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("DefaultDrawingAttributes");
return new DrawingAttributes(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setDefaultDrawingAttributes(DrawingAttributes DefaultDrawingAttributes) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("DefaultDrawingAttributes", DefaultDrawingAttributes == null ? null : DefaultDrawingAttributes.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public StrokeCollection getStrokes() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("Strokes");
return new StrokeCollection(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setStrokes(StrokeCollection Strokes) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Strokes", Strokes == null ? null : Strokes.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public StylusShape getEraserShape() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("EraserShape");
return new StylusShape(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setEraserShape(StylusShape EraserShape) throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("EraserShape", EraserShape == null ? null : EraserShape.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public StylusPointDescription getDefaultStylusPointDescription() throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("DefaultStylusPointDescription");
return new StylusPointDescription(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setDefaultStylusPointDescription(StylusPointDescription DefaultStylusPointDescription) throws Throwable, system.ArgumentNullException, system.FormatException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("DefaultStylusPointDescription", DefaultStylusPointDescription == null ? null : DefaultStylusPointDescription.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Brush getBackground() throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("Background");
return new Brush(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void setBackground(Brush Background) throws Throwable, system.ArgumentNullException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ArgumentException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Set("Background", Background == null ? null : Background.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
// Instance Events section
public void addSelectionChanged(EventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("SelectionChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeSelectionChanged(EventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("SelectionChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addSelectionMoved(EventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("SelectionMoved", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeSelectionMoved(EventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("SelectionMoved", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addSelectionResized(EventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("SelectionResized", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeSelectionResized(EventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("SelectionResized", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addGesture(InkCanvasGestureEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("Gesture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeGesture(InkCanvasGestureEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("Gesture", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addSelectionChanging(InkCanvasSelectionChangingEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("SelectionChanging", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeSelectionChanging(InkCanvasSelectionChangingEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("SelectionChanging", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addSelectionMoving(InkCanvasSelectionEditingEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("SelectionMoving", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeSelectionMoving(InkCanvasSelectionEditingEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("SelectionMoving", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addSelectionResizing(InkCanvasSelectionEditingEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("SelectionResizing", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeSelectionResizing(InkCanvasSelectionEditingEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("SelectionResizing", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStrokeCollected(InkCanvasStrokeCollectedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StrokeCollected", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStrokeCollected(InkCanvasStrokeCollectedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StrokeCollected", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStrokeErasing(InkCanvasStrokeErasingEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StrokeErasing", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStrokeErasing(InkCanvasStrokeErasingEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StrokeErasing", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStrokesReplaced(InkCanvasStrokesReplacedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StrokesReplaced", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStrokesReplaced(InkCanvasStrokesReplacedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StrokesReplaced", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addDefaultDrawingAttributesReplaced(DrawingAttributesReplacedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("DefaultDrawingAttributesReplaced", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeDefaultDrawingAttributesReplaced(DrawingAttributesReplacedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("DefaultDrawingAttributesReplaced", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addActiveEditingModeChanged(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("ActiveEditingModeChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeActiveEditingModeChanged(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("ActiveEditingModeChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addEditingModeChanged(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("EditingModeChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeEditingModeChanged(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("EditingModeChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addEditingModeInvertedChanged(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("EditingModeInvertedChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeEditingModeInvertedChanged(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("EditingModeInvertedChanged", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void addStrokeErased(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.RegisterEventListener("StrokeErased", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void removeStrokeErased(RoutedEventHandler handler) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.UnregisterEventListener("StrokeErased", handler);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
}