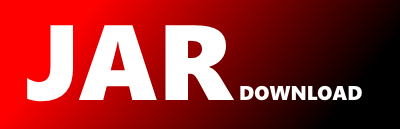
system.xml.XmlWriter Maven / Gradle / Ivy
/*
* MIT License
*
* Copyright (c) 2024 MASES s.r.l.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**************************************************************************************
*
* This code was generated from a template using JCOReflector v. 1.14.0.0
*
* Manual changes to this file may cause unexpected behavior in your application.
* Manual changes to this file will be overwritten if the code is regenerated.
*
*************************************************************************************/
package system.xml;
import org.mases.jcobridge.*;
import org.mases.jcobridge.netreflection.*;
import java.util.ArrayList;
// Import section
import system.threading.tasks.Task;
import system.xml.XmlReader;
import system.xml.xpath.XPathNavigator;
import system.xml.XmlWriter;
import system.io.Stream;
import system.xml.XmlWriterSettings;
import system.io.TextWriter;
import system.text.StringBuilder;
import system.Single;
import system.DateTime;
import system.DateTimeOffset;
import system.Decimal;
import system.xml.WriteState;
import system.xml.XmlSpace;
/**
* The base .NET class managing System.Xml.XmlWriter, System.Xml, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089.
*
*
* .NET documentation at https://docs.microsoft.com/en-us/dotnet/api/System.Xml.XmlWriter
*
*
* Powered by JCOBridge: more info at https://www.jcobridge.com
*
* @author MASES s.r.l https://masesgroup.com
* @version 1.14.0.0
*/
public class XmlWriter extends NetObject implements AutoCloseable {
/**
* Fully assembly qualified name: System.Xml, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089
*/
public static final String assemblyFullName = "System.Xml, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089";
/**
* Assembly name: System.Xml
*/
public static final String assemblyShortName = "System.Xml";
/**
* Qualified class name: System.Xml.XmlWriter
*/
public static final String className = "System.Xml.XmlWriter";
static JCOBridge bridge = JCOBridgeInstance.getInstance(assemblyFullName);
/**
* The type managed from JCOBridge. See {@link JCType}
*/
public static JCType classType = createType();
static JCEnum enumInstance = null;
JCObject classInstance = null;
static JCType createType() {
try {
String classToCreate = className + ", "
+ (JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
if (JCOReflector.getDebug())
JCOReflector.writeLog("Creating %s", classToCreate);
JCType typeCreated = bridge.GetType(classToCreate);
if (JCOReflector.getDebug())
JCOReflector.writeLog("Created: %s",
(typeCreated != null) ? typeCreated.toString() : "Returned null value");
return typeCreated;
} catch (JCException e) {
JCOReflector.writeLog(e);
return null;
}
}
void addReference(String ref) throws Throwable {
try {
bridge.AddReference(ref);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
/**
* Internal constructor. Use with caution
*/
public XmlWriter(java.lang.Object instance) throws Throwable {
super(instance);
if (instance instanceof JCObject) {
classInstance = (JCObject) instance;
} else
throw new Exception("Cannot manage object, it is not a JCObject");
}
public String getJCOAssemblyName() {
return assemblyFullName;
}
public String getJCOClassName() {
return className;
}
public String getJCOObjectName() {
return className + ", " + (JCOReflector.getUseFullAssemblyName() ? assemblyFullName : assemblyShortName);
}
public java.lang.Object getJCOInstance() {
return classInstance;
}
public void setJCOInstance(JCObject instance) {
classInstance = instance;
super.setJCOInstance(classInstance);
}
public JCType getJCOType() {
return classType;
}
/**
* Try to cast the {@link IJCOBridgeReflected} instance into {@link XmlWriter}, a cast assert is made to check if types are compatible.
* @param from {@link IJCOBridgeReflected} instance to be casted
* @return {@link XmlWriter} instance
* @throws java.lang.Throwable in case of error during cast operation
*/
public static XmlWriter cast(IJCOBridgeReflected from) throws Throwable {
NetType.AssertCast(classType, from);
return new XmlWriter(from.getJCOInstance());
}
// Constructors section
public XmlWriter() throws Throwable {
}
// Methods section
public java.lang.String LookupPrefix(java.lang.String ns) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Invoke("LookupPrefix", ns);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task FlushAsync() throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objFlushAsync = (JCObject)classInstance.Invoke("FlushAsync");
return new Task(objFlushAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteAttributesAsync(XmlReader reader, boolean defattr) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.security.SecurityException, system.InvalidOperationException, system.ArgumentException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteAttributesAsync = (JCObject)classInstance.Invoke("WriteAttributesAsync", reader == null ? null : reader.getJCOInstance(), defattr);
return new Task(objWriteAttributesAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteAttributeStringAsync(java.lang.String prefix, java.lang.String localName, java.lang.String ns, java.lang.String value) throws Throwable, system.NotImplementedException, system.ArgumentNullException, system.ArgumentException, system.ArgumentOutOfRangeException, system.security.SecurityException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteAttributeStringAsync = (JCObject)classInstance.Invoke("WriteAttributeStringAsync", prefix, localName, ns, value);
return new Task(objWriteAttributeStringAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteBase64Async(byte[] buffer, int index, int count) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteBase64Async = (JCObject)classInstance.Invoke("WriteBase64Async", buffer, index, count);
return new Task(objWriteBase64Async);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteBase64Async(JCORefOut dupParam0, int dupParam1, int dupParam2) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteBase64Async = (JCObject)classInstance.Invoke("WriteBase64Async", dupParam0.getJCRefOut(), dupParam1, dupParam2);
return new Task(objWriteBase64Async);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteBinHexAsync(byte[] buffer, int index, int count) throws Throwable, system.ArgumentNullException, system.security.SecurityException, system.InvalidOperationException, system.NullReferenceException, system.ArgumentException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteBinHexAsync = (JCObject)classInstance.Invoke("WriteBinHexAsync", buffer, index, count);
return new Task(objWriteBinHexAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteBinHexAsync(JCORefOut dupParam0, int dupParam1, int dupParam2) throws Throwable, system.ArgumentNullException, system.security.SecurityException, system.InvalidOperationException, system.NullReferenceException, system.ArgumentException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteBinHexAsync = (JCObject)classInstance.Invoke("WriteBinHexAsync", dupParam0.getJCRefOut(), dupParam1, dupParam2);
return new Task(objWriteBinHexAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteCDataAsync(java.lang.String text) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteCDataAsync = (JCObject)classInstance.Invoke("WriteCDataAsync", text);
return new Task(objWriteCDataAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteCharEntityAsync(char ch) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteCharEntityAsync = (JCObject)classInstance.Invoke("WriteCharEntityAsync", ch);
return new Task(objWriteCharEntityAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteCharsAsync(char[] buffer, int index, int count) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteCharsAsync = (JCObject)classInstance.Invoke("WriteCharsAsync", buffer, index, count);
return new Task(objWriteCharsAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteCharsAsync(JCORefOut dupParam0, int dupParam1, int dupParam2) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteCharsAsync = (JCObject)classInstance.Invoke("WriteCharsAsync", dupParam0.getJCRefOut(), dupParam1, dupParam2);
return new Task(objWriteCharsAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteCommentAsync(java.lang.String text) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteCommentAsync = (JCObject)classInstance.Invoke("WriteCommentAsync", text);
return new Task(objWriteCommentAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteDocTypeAsync(java.lang.String name, java.lang.String pubid, java.lang.String sysid, java.lang.String subset) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteDocTypeAsync = (JCObject)classInstance.Invoke("WriteDocTypeAsync", name, pubid, sysid, subset);
return new Task(objWriteDocTypeAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteElementStringAsync(java.lang.String prefix, java.lang.String localName, java.lang.String ns, java.lang.String value) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.security.SecurityException, system.InvalidOperationException, system.ArgumentException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteElementStringAsync = (JCObject)classInstance.Invoke("WriteElementStringAsync", prefix, localName, ns, value);
return new Task(objWriteElementStringAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteEndDocumentAsync() throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteEndDocumentAsync = (JCObject)classInstance.Invoke("WriteEndDocumentAsync");
return new Task(objWriteEndDocumentAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteEndElementAsync() throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteEndElementAsync = (JCObject)classInstance.Invoke("WriteEndElementAsync");
return new Task(objWriteEndElementAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteEntityRefAsync(java.lang.String name) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteEntityRefAsync = (JCObject)classInstance.Invoke("WriteEntityRefAsync", name);
return new Task(objWriteEntityRefAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteFullEndElementAsync() throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteFullEndElementAsync = (JCObject)classInstance.Invoke("WriteFullEndElementAsync");
return new Task(objWriteFullEndElementAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteNameAsync(java.lang.String name) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.FormatException, system.ArgumentException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteNameAsync = (JCObject)classInstance.Invoke("WriteNameAsync", name);
return new Task(objWriteNameAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteNmTokenAsync(java.lang.String name) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteNmTokenAsync = (JCObject)classInstance.Invoke("WriteNmTokenAsync", name);
return new Task(objWriteNmTokenAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteNodeAsync(XmlReader reader, boolean defattr) throws Throwable, system.ArgumentNullException, system.security.SecurityException, system.InvalidOperationException, system.NullReferenceException, system.ArgumentException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteNodeAsync = (JCObject)classInstance.Invoke("WriteNodeAsync", reader == null ? null : reader.getJCOInstance(), defattr);
return new Task(objWriteNodeAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteNodeAsync(XPathNavigator navigator, boolean defattr) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.security.SecurityException, system.InvalidOperationException, system.ArgumentException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteNodeAsync = (JCObject)classInstance.Invoke("WriteNodeAsync", navigator == null ? null : navigator.getJCOInstance(), defattr);
return new Task(objWriteNodeAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteProcessingInstructionAsync(java.lang.String name, java.lang.String text) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteProcessingInstructionAsync = (JCObject)classInstance.Invoke("WriteProcessingInstructionAsync", name, text);
return new Task(objWriteProcessingInstructionAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteQualifiedNameAsync(java.lang.String localName, java.lang.String ns) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.security.SecurityException, system.InvalidOperationException, system.ArgumentException, system.NullReferenceException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteQualifiedNameAsync = (JCObject)classInstance.Invoke("WriteQualifiedNameAsync", localName, ns);
return new Task(objWriteQualifiedNameAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteRawAsync(char[] buffer, int index, int count) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteRawAsync = (JCObject)classInstance.Invoke("WriteRawAsync", buffer, index, count);
return new Task(objWriteRawAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteRawAsync(JCORefOut dupParam0, int dupParam1, int dupParam2) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteRawAsync = (JCObject)classInstance.Invoke("WriteRawAsync", dupParam0.getJCRefOut(), dupParam1, dupParam2);
return new Task(objWriteRawAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteRawAsync(java.lang.String data) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteRawAsync = (JCObject)classInstance.Invoke("WriteRawAsync", data);
return new Task(objWriteRawAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteStartDocumentAsync() throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteStartDocumentAsync = (JCObject)classInstance.Invoke("WriteStartDocumentAsync");
return new Task(objWriteStartDocumentAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteStartDocumentAsync(boolean standalone) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteStartDocumentAsync = (JCObject)classInstance.Invoke("WriteStartDocumentAsync", standalone);
return new Task(objWriteStartDocumentAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteStartElementAsync(java.lang.String prefix, java.lang.String localName, java.lang.String ns) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteStartElementAsync = (JCObject)classInstance.Invoke("WriteStartElementAsync", prefix, localName, ns);
return new Task(objWriteStartElementAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteStringAsync(java.lang.String text) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteStringAsync = (JCObject)classInstance.Invoke("WriteStringAsync", text);
return new Task(objWriteStringAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteSurrogateCharEntityAsync(char lowChar, char highChar) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteSurrogateCharEntityAsync = (JCObject)classInstance.Invoke("WriteSurrogateCharEntityAsync", lowChar, highChar);
return new Task(objWriteSurrogateCharEntityAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public Task WriteWhitespaceAsync(java.lang.String ws) throws Throwable, system.NotImplementedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject objWriteWhitespaceAsync = (JCObject)classInstance.Invoke("WriteWhitespaceAsync", ws);
return new Task(objWriteWhitespaceAsync);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static XmlWriter Create(Stream output) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ObjectDisposedException, system.threading.AbandonedMutexException, system.ArgumentException, system.InvalidOperationException, system.NotSupportedException, system.NotImplementedException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", output == null ? null : output.getJCOInstance());
return new XmlWriter(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static XmlWriter Create(Stream output, XmlWriterSettings settings) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ObjectDisposedException, system.threading.AbandonedMutexException, system.ArgumentException, system.InvalidOperationException, system.NotSupportedException, system.NotImplementedException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", output == null ? null : output.getJCOInstance(), settings == null ? null : settings.getJCOInstance());
return new XmlWriter(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static XmlWriter Create(TextWriter output) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.NotSupportedException, system.IndexOutOfRangeException, system.ObjectDisposedException, system.resources.MissingManifestResourceException, system.InvalidOperationException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", output == null ? null : output.getJCOInstance());
return new XmlWriter(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static XmlWriter Create(TextWriter output, XmlWriterSettings settings) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.NotSupportedException, system.IndexOutOfRangeException, system.ObjectDisposedException, system.resources.MissingManifestResourceException, system.InvalidOperationException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", output == null ? null : output.getJCOInstance(), settings == null ? null : settings.getJCOInstance());
return new XmlWriter(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static XmlWriter Create(java.lang.String outputFileName) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.xml.XmlException, system.ArgumentException, system.io.PathTooLongException, system.io.FileNotFoundException, system.io.DirectoryNotFoundException, system.UnauthorizedAccessException, system.io.IOException, system.io.DriveNotFoundException, system.OperationCanceledException, system.NotSupportedException, system.InvalidOperationException, system.NullReferenceException, system.security.SecurityException, system.ObjectDisposedException, system.NotImplementedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", outputFileName);
return new XmlWriter(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static XmlWriter Create(java.lang.String outputFileName, XmlWriterSettings settings) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.InvalidOperationException, system.xml.XmlException, system.ArgumentException, system.io.PathTooLongException, system.NotSupportedException, system.NullReferenceException, system.security.SecurityException, system.io.IOException, system.ObjectDisposedException, system.threading.AbandonedMutexException, system.NotImplementedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", outputFileName, settings == null ? null : settings.getJCOInstance());
return new XmlWriter(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static XmlWriter Create(StringBuilder output) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.NotSupportedException, system.IndexOutOfRangeException, system.ObjectDisposedException, system.resources.MissingManifestResourceException, system.InvalidOperationException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", output == null ? null : output.getJCOInstance());
return new XmlWriter(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static XmlWriter Create(StringBuilder output, XmlWriterSettings settings) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.NotSupportedException, system.IndexOutOfRangeException, system.ObjectDisposedException, system.resources.MissingManifestResourceException, system.InvalidOperationException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", output == null ? null : output.getJCOInstance(), settings == null ? null : settings.getJCOInstance());
return new XmlWriter(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static XmlWriter Create(XmlWriter output) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ArgumentException, system.NotImplementedException, system.NotSupportedException, system.IndexOutOfRangeException, system.ObjectDisposedException, system.InvalidOperationException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", output == null ? null : output.getJCOInstance());
return new XmlWriter(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public static XmlWriter Create(XmlWriter output, XmlWriterSettings settings) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.NotImplementedException, system.NotSupportedException, system.IndexOutOfRangeException, system.ObjectDisposedException {
if (classType == null)
throw new UnsupportedOperationException("classType is null.");
try {
JCObject objCreate = (JCObject)classType.Invoke("Create", output == null ? null : output.getJCOInstance(), settings == null ? null : settings.getJCOInstance());
return new XmlWriter(objCreate);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Close() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Close");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Dispose() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Dispose");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void Flush() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Flush");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteAttributes(XmlReader reader, boolean defattr) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.IndexOutOfRangeException, system.xml.XmlException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteAttributes", reader == null ? null : reader.getJCOInstance(), defattr);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteAttributeString(java.lang.String localName, java.lang.String value) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteAttributeString", localName, value);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteAttributeString(java.lang.String localName, java.lang.String ns, java.lang.String value) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteAttributeString", localName, ns, value);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteAttributeString(java.lang.String prefix, java.lang.String localName, java.lang.String ns, java.lang.String value) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteAttributeString", prefix, localName, ns, value);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteBase64(byte[] buffer, int index, int count) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteBase64", buffer, index, count);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteBase64(JCORefOut dupParam0, int dupParam1, int dupParam2) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteBase64", dupParam0.getJCRefOut(), dupParam1, dupParam2);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteBinHex(byte[] buffer, int index, int count) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteBinHex", buffer, index, count);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteBinHex(JCORefOut dupParam0, int dupParam1, int dupParam2) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteBinHex", dupParam0.getJCRefOut(), dupParam1, dupParam2);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteCData(java.lang.String text) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteCData", text);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteCharEntity(char ch) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteCharEntity", ch);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteChars(char[] buffer, int index, int count) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteChars", buffer, index, count);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteChars(JCORefOut dupParam0, int dupParam1, int dupParam2) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteChars", dupParam0.getJCRefOut(), dupParam1, dupParam2);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteComment(java.lang.String text) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteComment", text);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteDocType(java.lang.String name, java.lang.String pubid, java.lang.String sysid, java.lang.String subset) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteDocType", name, pubid, sysid, subset);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteElementString(java.lang.String localName, java.lang.String value) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteElementString", localName, value);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteElementString(java.lang.String localName, java.lang.String ns, java.lang.String value) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteElementString", localName, ns, value);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteElementString(java.lang.String prefix, java.lang.String localName, java.lang.String ns, java.lang.String value) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteElementString", prefix, localName, ns, value);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteEndAttribute() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteEndAttribute");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteEndDocument() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteEndDocument");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteEndElement() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteEndElement");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteEntityRef(java.lang.String name) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteEntityRef", name);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteFullEndElement() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteFullEndElement");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteName(java.lang.String name) throws Throwable, system.ArgumentNullException, system.ArgumentOutOfRangeException, system.FormatException, system.ArgumentException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteName", name);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteNmToken(java.lang.String name) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteNmToken", name);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteNode(XmlReader reader, boolean defattr) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.resources.MissingManifestResourceException, system.NotImplementedException, system.ObjectDisposedException, system.InvalidOperationException, system.ArgumentOutOfRangeException, system.IndexOutOfRangeException, system.xml.XmlException, system.NotSupportedException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteNode", reader == null ? null : reader.getJCOInstance(), defattr);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteNode(XPathNavigator navigator, boolean defattr) throws Throwable, system.ArgumentNullException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteNode", navigator == null ? null : navigator.getJCOInstance(), defattr);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteProcessingInstruction(java.lang.String name, java.lang.String text) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteProcessingInstruction", name, text);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteQualifiedName(java.lang.String localName, java.lang.String ns) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.NotSupportedException, system.ArgumentOutOfRangeException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.FormatException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteQualifiedName", localName, ns);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteRaw(char[] buffer, int index, int count) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteRaw", buffer, index, count);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteRaw(JCORefOut dupParam0, int dupParam1, int dupParam2) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteRaw", dupParam0.getJCRefOut(), dupParam1, dupParam2);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteRaw(java.lang.String data) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteRaw", data);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteStartAttribute(java.lang.String localName) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteStartAttribute", localName);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteStartAttribute(java.lang.String localName, java.lang.String ns) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteStartAttribute", localName, ns);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteStartAttribute(java.lang.String prefix, java.lang.String localName, java.lang.String ns) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteStartAttribute", prefix, localName, ns);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteStartDocument() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteStartDocument");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteStartDocument(boolean standalone) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteStartDocument", standalone);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteStartElement(java.lang.String localName) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteStartElement", localName);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteStartElement(java.lang.String localName, java.lang.String ns) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteStartElement", localName, ns);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteStartElement(java.lang.String prefix, java.lang.String localName, java.lang.String ns) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteStartElement", prefix, localName, ns);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteString(java.lang.String text) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteString", text);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteSurrogateCharEntity(char lowChar, char highChar) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteSurrogateCharEntity", lowChar, highChar);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteValue(boolean value) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteValue", value);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteValue(double value) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteValue", value);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteValue(int value) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteValue", value);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteValue(long value) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteValue", value);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteValue(Single value) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteValue", value == null ? null : value.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteValue(DateTime value) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentException, system.OverflowException, system.ArgumentNullException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.IndexOutOfRangeException, system.FormatException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteValue", value == null ? null : value.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteValue(DateTimeOffset value) throws Throwable, system.ArgumentOutOfRangeException, system.ArgumentException, system.OverflowException, system.ArgumentNullException, system.NotImplementedException, system.globalization.CultureNotFoundException, system.IndexOutOfRangeException, system.resources.MissingManifestResourceException, system.ObjectDisposedException, system.InvalidOperationException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteValue", value == null ? null : value.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteValue(Decimal value) throws Throwable, system.ArgumentNullException, system.ArgumentException, system.InvalidOperationException, system.MissingMethodException, system.reflection.TargetInvocationException, system.ArgumentOutOfRangeException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteValue", value == null ? null : value.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteValue(NetObject value) throws Throwable, system.ArgumentNullException {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteValue", value == null ? null : value.getJCOInstance());
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteValue(java.lang.String value) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteValue", value);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void WriteWhitespace(java.lang.String ws) throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("WriteWhitespace", ws);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public void close() throws Exception {
try {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
classInstance.Invoke("Dispose");
}
catch (JCNativeException jcne) {
throw translateException(jcne);
}
} catch (Throwable t) {
throw new Exception(t);
}
}
// Properties section
public java.lang.String getXmlLang() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
return (java.lang.String)classInstance.Get("XmlLang");
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public WriteState getWriteState() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("WriteState");
return new WriteState(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public XmlSpace getXmlSpace() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("XmlSpace");
return new XmlSpace(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
public XmlWriterSettings getSettings() throws Throwable {
if (classInstance == null)
throw new UnsupportedOperationException("classInstance is null.");
try {
JCObject val = (JCObject)classInstance.Get("Settings");
return new XmlWriterSettings(val);
} catch (JCNativeException jcne) {
throw translateException(jcne);
}
}
// Instance Events section
}