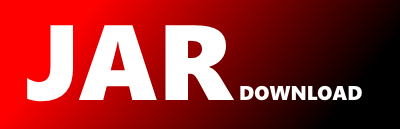
com.mastercard.api.mediameasurement.MediaMeasurements Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of media-measurement Show documentation
Show all versions of media-measurement Show documentation
Java Core SDK for use with SDKs available on MasterCard Developer Zone (https://developer.mastercard.com)
/*
* Copyright 2016 MasterCard International.
*
* Redistribution and use in source and binary forms, with or without modification, are
* permitted provided that the following conditions are met:
*
* Redistributions of source code must retain the above copyright notice, this list of
* conditions and the following disclaimer.
* Redistributions in binary form must reproduce the above copyright notice, this list of
* conditions and the following disclaimer in the documentation and/or other materials
* provided with the distribution.
* Neither the name of the MasterCard International Incorporated nor the names of its
* contributors may be used to endorse or promote products derived from this software
* without specific prior written permission.
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY
* EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT
* SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED
* TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS;
* OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER
* IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING
* IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF
* SUCH DAMAGE.
*
*/
package com.mastercard.api.mediameasurement;
import com.mastercard.api.core.exception.*;
import com.mastercard.api.core.model.*;
import com.mastercard.api.core.security.*;
import java.util.Arrays;
import java.util.List;
public class MediaMeasurements extends BaseObject {
public MediaMeasurements() {
}
public MediaMeasurements(BaseObject o) {
putAll(o);
}
public MediaMeasurements(RequestMap requestMap) {
putAll(requestMap);
}
@Override
protected String getResourcePath(Action action) throws IllegalArgumentException {
if (action == null) {
throw new IllegalArgumentException("Action cannot be null");
}
if (action == Action.create) {
return "/mediameasurement/v1/mediameasurementsvc.svc/mediameasurements";
}
throw new IllegalArgumentException("Invalid action supplied: " + action);
}
@Override
protected List getHeaderParams(Action action) throws IllegalArgumentException {
if (action == null) {
throw new IllegalArgumentException("Action cannot be null");
}
if (action == Action.create) {
return Arrays.asList();
}
throw new IllegalArgumentException("Invalid action supplied: " + action);
}
@Override
protected List getQueryParams(Action action) throws IllegalArgumentException {
if (action == null) {
throw new IllegalArgumentException("Action cannot be null");
}
else if (action == Action.create) {
return Arrays.asList();
}
throw new IllegalArgumentException("Invalid action supplied: " + action);
}
@Override
protected String getApiVersion() {
return "1.0.2";
}
/**
* Creates a MediaMeasurements
object
*
* @param map a map of parameters to create a MediaMeasurements
object
*
* @return a MediaMeasurements object.
*
* @throws ApiCommunicationException
* @throws AuthenticationException
* @throws InvalidRequestException
* @throws MessageSignerException
* @throws NotAllowedException
* @throws ObjectNotFoundException
* @throws SystemException
*/
public static MediaMeasurements create(RequestMap map)
throws ApiCommunicationException, AuthenticationException, InvalidRequestException,
MessageSignerException, NotAllowedException, ObjectNotFoundException, SystemException {
return create(null, map);
}
/**
* Creates a MediaMeasurements
object
*
* @param auth Authentication object overriding ApiConfig.setAuthentication(authentication)
* @param map a map of parameters to create a MediaMeasurements
object
*
* @return a MediaMeasurements object.
*
* @throws ApiCommunicationException
* @throws AuthenticationException
* @throws InvalidRequestException
* @throws MessageSignerException
* @throws NotAllowedException
* @throws ObjectNotFoundException
* @throws SystemException
*/
public static MediaMeasurements create(Authentication auth, RequestMap map)
throws ApiCommunicationException, AuthenticationException, InvalidRequestException,
MessageSignerException, NotAllowedException, ObjectNotFoundException, SystemException {
return new MediaMeasurements(BaseObject.createObject(auth, new MediaMeasurements(map)));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy