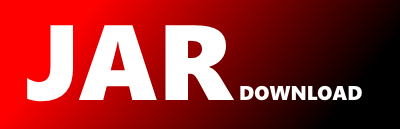
com.mastercard.masterpass.merchant.model.CheckoutExtension Maven / Gradle / Ivy
package com.mastercard.masterpass.merchant.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.mastercard.masterpass.merchant.model.CheckoutDSRP;
import com.mastercard.masterpass.merchant.model.ExtensionPoint;
import com.mastercard.masterpass.merchant.model.InstallmentOption;
import com.mastercard.masterpass.merchant.model.PrivateDatas;
import com.mastercard.masterpass.merchant.model.RiskDecisioning;
import com.mastercard.masterpass.merchant.model.Tokenization;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.google.gson.annotations.SerializedName;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.Root;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
/**
* This class contains methods require to set checkout details during DSRP.
**/
@Root(name = "CheckoutExtension")
@XmlRootElement (name = "CheckoutExtension")
public class CheckoutExtension {
@SerializedName("CardVerificationStatus")
@Element(name = "CardVerificationStatus", required = false)
private String cardVerificationStatus = null;
@SerializedName("LoginAuthenticationMethod")
@Element(name = "LoginAuthenticationMethod", required = false)
private String loginAuthenticationMethod = null;
@SerializedName("RiskDecisioning")
@Element(name = "RiskDecisioning", required = false)
private RiskDecisioning riskDecisioning = null;
@SerializedName("DSRP")
@Element(name = "DSRP", required = false)
private CheckoutDSRP DSRP = null;
@SerializedName("PrivateDatas")
@Element(name = "PrivateDatas", required = false)
private PrivateDatas privateDatas = null;
@SerializedName("InstallmentOption")
@Element(name = "InstallmentOption", required = false)
private InstallmentOption installmentOption = null;
@SerializedName("Tokenization")
@Element(name = "Tokenization", required = false)
private Tokenization tokenization = null;
@SerializedName("PaymentAccountReference")
@Element(name = "PaymentAccountReference", required = false)
private String paymentAccountReference = null;
@SerializedName("ExtensionPoint")
@Element(name = "ExtensionPoint", required = false)
private ExtensionPoint extensionPoint = null;
/**
* Gets the card verification status.
*
* @return the card verification status.
**/
@XmlElement(name = "CardVerificationStatus")
public String getCardVerificationStatus() {
return cardVerificationStatus;
}
/**
* Sets the card verification status.
*
* @param cardVerificationStatus the card verification status.
*/
public CheckoutExtension cardVerificationStatus(String cardVerificationStatus) {
this.cardVerificationStatus = cardVerificationStatus;
return this;
}
/**
* Gets the login authentication method.
*
* @return the login authentication method.
**/
@XmlElement(name = "LoginAuthenticationMethod")
public String getLoginAuthenticationMethod() {
return loginAuthenticationMethod;
}
/**
* Sets the login authentication method.
*
* @param loginAuthenticationMethod the login authentication method.
*/
public CheckoutExtension loginAuthenticationMethod(String loginAuthenticationMethod) {
this.loginAuthenticationMethod = loginAuthenticationMethod;
return this;
}
/**
* Gets the risk decisioning data.
*
* @return the risk decisioning data.
**/
@XmlElement(name = "RiskDecisioning")
public RiskDecisioning getRiskDecisioning() {
return riskDecisioning;
}
/**
* Sets the risk decisioning data.
*
* @param riskDecisioning the risk decisioning data.
*/
public CheckoutExtension riskDecisioning(RiskDecisioning riskDecisioning) {
this.riskDecisioning = riskDecisioning;
return this;
}
/**
* Gets the DSRP data.
*
* @return the DSRP data.
**/
@XmlElement(name = "DSRP")
public CheckoutDSRP getDSRP() {
return DSRP;
}
/**
* Sets the DSRP data.
*
* @param DSRP the DSRP data.
*/
public CheckoutExtension DSRP(CheckoutDSRP DSRP) {
this.DSRP = DSRP;
return this;
}
/**
* Gets the private data details.
*
* @return the private data details.
**/
@XmlElement(name = "PrivateDatas")
public PrivateDatas getPrivateDatas() {
return privateDatas;
}
/**
* Sets the private data details.
*
* @param privateDatas the private data details.
*/
public CheckoutExtension privateDatas(PrivateDatas privateDatas) {
this.privateDatas = privateDatas;
return this;
}
/**
* Gets the installment options details.
*
* @return the installment options details.
**/
@XmlElement(name = "InstallmentOption")
public InstallmentOption getInstallmentOption() {
return installmentOption;
}
/**
* Sets the installment options details.
*
* @param installmentOption the installment options details.
*/
public CheckoutExtension installmentOption(InstallmentOption installmentOption) {
this.installmentOption = installmentOption;
return this;
}
/**
* Gets the token details.
*
* @return the token details.
**/
@XmlElement(name = "Tokenization")
public Tokenization getTokenization() {
return tokenization;
}
/**
* Sets the token details.
*
* @param tokenization the token details.
*/
public CheckoutExtension tokenization(Tokenization tokenization) {
this.tokenization = tokenization;
return this;
}
/**
* Gets the payment account reference.
*
* @return the payment account reference.
**/
@XmlElement(name = "PaymentAccountReference")
public String getPaymentAccountReference() {
return paymentAccountReference;
}
/**
* Sets the payment account reference.
*
* @param paymentAccountReference the payment account reference.
*/
public CheckoutExtension paymentAccountReference(String paymentAccountReference) {
this.paymentAccountReference = paymentAccountReference;
return this;
}
/**
* Gets the ExtensionPoint for future enhancement.
*
* @return the ExtensionPoint for future enhancement.
**/
@XmlElement(name = "ExtensionPoint")
public ExtensionPoint getExtensionPoint() {
return extensionPoint;
}
/**
* Sets the ExtensionPoint for future enhancement.
*
* @param extensionPoint the ExtensionPoint for future enhancement.
*/
public CheckoutExtension extensionPoint(ExtensionPoint extensionPoint) {
this.extensionPoint = extensionPoint;
return this;
}
/**
* Returns true if the arguments are equal to each other and false
* otherwise. Consequently, if both arguments are null, true is returned and
* if exactly one argument is null, false is returned. Otherwise, equality
* is determined by using the equals method of the first argument.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CheckoutExtension checkoutExtension = (CheckoutExtension) o;
return Objects.equals(cardVerificationStatus, checkoutExtension.cardVerificationStatus) &&
Objects.equals(loginAuthenticationMethod, checkoutExtension.loginAuthenticationMethod) &&
Objects.equals(riskDecisioning, checkoutExtension.riskDecisioning) &&
Objects.equals(DSRP, checkoutExtension.DSRP) &&
Objects.equals(privateDatas, checkoutExtension.privateDatas) &&
Objects.equals(installmentOption, checkoutExtension.installmentOption) &&
Objects.equals(tokenization, checkoutExtension.tokenization) &&
Objects.equals(paymentAccountReference, checkoutExtension.paymentAccountReference) &&
Objects.equals(extensionPoint, checkoutExtension.extensionPoint);
}
/**
* Generates a hash code for a sequence of input values.
*/
@Override
public int hashCode() {
return Objects.hash(cardVerificationStatus, loginAuthenticationMethod, riskDecisioning, DSRP, privateDatas, installmentOption, tokenization, paymentAccountReference, extensionPoint);
}
/**
* Returns the result of calling toString for a non-null argument and "null" for a null argument.
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CheckoutExtension {\n");
sb.append(" cardVerificationStatus: ").append(toIndentedString(cardVerificationStatus)).append("\n");
sb.append(" loginAuthenticationMethod: ").append(toIndentedString(loginAuthenticationMethod)).append("\n");
sb.append(" riskDecisioning: ").append(toIndentedString(riskDecisioning)).append("\n");
sb.append(" DSRP: ").append(toIndentedString(DSRP)).append("\n");
sb.append(" privateDatas: ").append(toIndentedString(privateDatas)).append("\n");
sb.append(" installmentOption: ").append(toIndentedString(installmentOption)).append("\n");
sb.append(" tokenization: ").append(toIndentedString(tokenization)).append("\n");
sb.append(" paymentAccountReference: ").append(toIndentedString(paymentAccountReference)).append("\n");
sb.append(" extensionPoint: ").append(toIndentedString(extensionPoint)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy