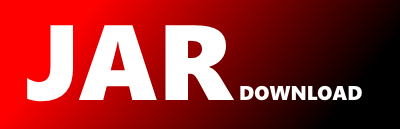
com.mastercard.masterpass.merchant.model.Contact Maven / Gradle / Ivy
package com.mastercard.masterpass.merchant.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.mastercard.masterpass.merchant.model.DateOfBirth;
import com.mastercard.masterpass.merchant.model.ExtensionPoint;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.google.gson.annotations.SerializedName;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.Root;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
/**
* This class contains methods to get consumer contact information.
**/
@Root(name = "Contact")
@XmlRootElement (name = "Contact")
public class Contact {
@SerializedName("FirstName")
@Element(name = "FirstName", required = false)
private String firstName = null;
@SerializedName("MiddleName")
@Element(name = "MiddleName", required = false)
private String middleName = null;
@SerializedName("LastName")
@Element(name = "LastName", required = false)
private String lastName = null;
public enum GenderEnum {
@SerializedName("M")
M("M"),
@SerializedName("F")
F("F");
private String value;
GenderEnum(String value) {
this.value = value;
}
@Override
public String toString() {
return value;
}
}
@SerializedName("Gender")
@Element(name = "Gender", required = false)
private GenderEnum gender = null;
@SerializedName("DateOfBirth")
@Element(name = "DateOfBirth", required = false)
private DateOfBirth dateOfBirth = null;
@SerializedName("NationalID")
@Element(name = "NationalID", required = false)
private String nationalID = null;
@SerializedName("Country")
@Element(name = "Country", required = false)
private String country = null;
@SerializedName("EmailAddress")
@Element(name = "EmailAddress", required = false)
private String emailAddress = null;
@SerializedName("PhoneNumber")
@Element(name = "PhoneNumber", required = false)
private String phoneNumber = null;
@SerializedName("ExtensionPoint")
@Element(name = "ExtensionPoint", required = false)
private ExtensionPoint extensionPoint = null;
/**
* Gets the contact first name.
*
* @return the contact first name.
**/
@XmlElement(name = "FirstName")
public String getFirstName() {
return firstName;
}
/**
* Sets the contact first name.
*
* @param firstName the contact first name.
*/
public Contact firstName(String firstName) {
this.firstName = firstName;
return this;
}
/**
* Gets the contact middle name or initial.
*
* @return the contact middle name or initial.
**/
@XmlElement(name = "MiddleName")
public String getMiddleName() {
return middleName;
}
/**
* Sets the contact middle name or initial.
*
* @param middleName the contact middle name or initial.
*/
public Contact middleName(String middleName) {
this.middleName = middleName;
return this;
}
/**
* Gets the contact surname.
*
* @return the contact surname.
**/
@XmlElement(name = "LastName")
public String getLastName() {
return lastName;
}
/**
* Sets the contact surname.
*
* @param lastName the contact surname.
*/
public Contact lastName(String lastName) {
this.lastName = lastName;
return this;
}
/**
* Gets the contact Gender (M or F). NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
*
* @return the contact Gender (M or F). NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
**/
@XmlElement(name = "Gender")
public GenderEnum getGender() {
return gender;
}
/**
* Sets the contact Gender (M or F). NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
*
* @param gender the contact Gender (M or F). NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
*/
public Contact gender(GenderEnum gender) {
this.gender = gender;
return this;
}
/**
* Gets the contact DOB - YYYY/MM/DD. NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
*
* @return the contact DOB - YYYY/MM/DD. NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
**/
@XmlElement(name = "DateOfBirth")
public DateOfBirth getDateOfBirth() {
return dateOfBirth;
}
/**
* Sets the contact DOB - YYYY/MM/DD. NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
*
* @param dateOfBirth the contact DOB - YYYY/MM/DD. NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
*/
public Contact dateOfBirth(DateOfBirth dateOfBirth) {
this.dateOfBirth = dateOfBirth;
return this;
}
/**
* Gets the contact National Identification. NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
*
* @return the contact National Identification. NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
**/
@XmlElement(name = "NationalID")
public String getNationalID() {
return nationalID;
}
/**
* Sets the contact National Identification. NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
*
* @param nationalID the contact National Identification. NOTE: This field may only be requested from a MasterPass wallet if it is required by law in a region. Merchants and service providers seeking to use this field must work with the local MasterPass representative to get the necessary clearances before requesting these data elements.
*/
public Contact nationalID(String nationalID) {
this.nationalID = nationalID;
return this;
}
/**
* Gets the contact country of residence.
*
* @return the contact country of residence.
**/
@XmlElement(name = "Country")
public String getCountry() {
return country;
}
/**
* Sets the contact country of residence.
*
* @param country the contact country of residence.
*/
public Contact country(String country) {
this.country = country;
return this;
}
/**
* Gets the contact email aAddress.
*
* @return the contact email aAddress.
**/
@XmlElement(name = "EmailAddress")
public String getEmailAddress() {
return emailAddress;
}
/**
* Sets the contact email aAddress.
*
* @param emailAddress the contact email aAddress.
*/
public Contact emailAddress(String emailAddress) {
this.emailAddress = emailAddress;
return this;
}
/**
* Gets the contact phone.
*
* @return the contact phone.
**/
@XmlElement(name = "PhoneNumber")
public String getPhoneNumber() {
return phoneNumber;
}
/**
* Sets the contact phone.
*
* @param phoneNumber the contact phone.
*/
public Contact phoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
return this;
}
/**
* Gets the ExtensionPoint for future enhancement.
*
* @return the ExtensionPoint for future enhancement.
**/
@XmlElement(name = "ExtensionPoint")
public ExtensionPoint getExtensionPoint() {
return extensionPoint;
}
/**
* Sets the ExtensionPoint for future enhancement.
*
* @param extensionPoint the ExtensionPoint for future enhancement.
*/
public Contact extensionPoint(ExtensionPoint extensionPoint) {
this.extensionPoint = extensionPoint;
return this;
}
/**
* Returns true if the arguments are equal to each other and false
* otherwise. Consequently, if both arguments are null, true is returned and
* if exactly one argument is null, false is returned. Otherwise, equality
* is determined by using the equals method of the first argument.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Contact contact = (Contact) o;
return Objects.equals(firstName, contact.firstName) &&
Objects.equals(middleName, contact.middleName) &&
Objects.equals(lastName, contact.lastName) &&
Objects.equals(gender, contact.gender) &&
Objects.equals(dateOfBirth, contact.dateOfBirth) &&
Objects.equals(nationalID, contact.nationalID) &&
Objects.equals(country, contact.country) &&
Objects.equals(emailAddress, contact.emailAddress) &&
Objects.equals(phoneNumber, contact.phoneNumber) &&
Objects.equals(extensionPoint, contact.extensionPoint);
}
/**
* Generates a hash code for a sequence of input values.
*/
@Override
public int hashCode() {
return Objects.hash(firstName, middleName, lastName, gender, dateOfBirth, nationalID, country, emailAddress, phoneNumber, extensionPoint);
}
/**
* Returns the result of calling toString for a non-null argument and "null" for a null argument.
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Contact {\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" middleName: ").append(toIndentedString(middleName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" gender: ").append(toIndentedString(gender)).append("\n");
sb.append(" dateOfBirth: ").append(toIndentedString(dateOfBirth)).append("\n");
sb.append(" nationalID: ").append(toIndentedString(nationalID)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append(" emailAddress: ").append(toIndentedString(emailAddress)).append("\n");
sb.append(" phoneNumber: ").append(toIndentedString(phoneNumber)).append("\n");
sb.append(" extensionPoint: ").append(toIndentedString(extensionPoint)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy