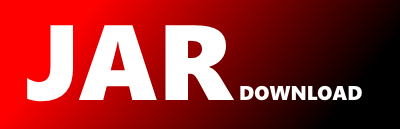
com.mastercard.masterpass.merchant.model.MerchantTransaction Maven / Gradle / Ivy
package com.mastercard.masterpass.merchant.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.mastercard.masterpass.merchant.model.ExtensionPoint;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.google.gson.annotations.SerializedName;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.Root;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
/**
* This class contains different methods for to send and retrieve transaction details.
**/
@Root(name = "MerchantTransaction")
@XmlRootElement (name = "MerchantTransaction")
public class MerchantTransaction {
@SerializedName("TransactionId")
@Element(name = "TransactionId")
private String transactionId = null;
@SerializedName("ConsumerKey")
@Element(name = "ConsumerKey", required = false)
private String consumerKey = null;
@SerializedName("Currency")
@Element(name = "Currency")
private String currency = null;
@SerializedName("OrderAmount")
@Element(name = "OrderAmount")
private Long orderAmount = null;
@SerializedName("PurchaseDate")
@Element(name = "PurchaseDate")
private String purchaseDate = null;
public enum TransactionStatusEnum {
@SerializedName("Success")
Success("Success"),
@SerializedName("Failure")
Failure("Failure");
private String value;
TransactionStatusEnum(String value) {
this.value = value;
}
@Override
public String toString() {
return value;
}
}
@SerializedName("TransactionStatus")
@Element(name = "TransactionStatus")
private TransactionStatusEnum transactionStatus = null;
@SerializedName("ApprovalCode")
@Element(name = "ApprovalCode")
private String approvalCode = null;
@SerializedName("PreCheckoutTransactionId")
@Element(name = "PreCheckoutTransactionId", required = false)
private String preCheckoutTransactionId = null;
@SerializedName("ExpressCheckoutIndicator")
@Element(name = "ExpressCheckoutIndicator", required = false)
private Boolean expressCheckoutIndicator = null;
@SerializedName("ExtensionPoint")
@Element(name = "ExtensionPoint", required = false)
private ExtensionPoint extensionPoint = null;
/**
* Gets the transaction Id.
*
* @return the transaction Id.
**/
@XmlElement(name = "TransactionId")
public String getTransactionId() {
return transactionId;
}
/**
* Sets the transaction Id.
*
* @param transactionId the transaction Id.
*/
public MerchantTransaction transactionId(String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* Gets the consumer key from checkout project.
*
* @return the consumer key from checkout project.
**/
@XmlElement(name = "ConsumerKey")
public String getConsumerKey() {
return consumerKey;
}
/**
* Sets the consumer key from checkout project.
*
* @param consumerKey the consumer key from checkout project.
*/
public MerchantTransaction consumerKey(String consumerKey) {
this.consumerKey = consumerKey;
return this;
}
/**
* Gets the currency for the transaction, for example, USD.
*
* @return the currency for the transaction, for example, USD.
**/
@XmlElement(name = "Currency")
public String getCurrency() {
return currency;
}
/**
* Sets the currency for the transaction, for example, USD.
*
* @param currency the currency for the transaction, for example, USD.
*/
public MerchantTransaction currency(String currency) {
this.currency = currency;
return this;
}
/**
* Gets the transaction order amount, for example, 1500 (for USD 15 transaction amount)
*
* @return the transaction order amount, for example, 1500 (for USD 15 transaction amount)
**/
@XmlElement(name = "OrderAmount")
public Long getOrderAmount() {
return orderAmount;
}
/**
* Sets the transaction order amount, for example, 1500 (for USD 15 transaction amount)
*
* @param orderAmount the transaction order amount, for example, 1500 (for USD 15 transaction amount)
*/
public MerchantTransaction orderAmount(Long orderAmount) {
this.orderAmount = orderAmount;
return this;
}
/**
* Gets the date of purchase.
*
* @return the date of purchase.
**/
@XmlElement(name = "PurchaseDate")
public String getPurchaseDate() {
return purchaseDate;
}
/**
* Sets the date of purchase.
*
* @param purchaseDate the date of purchase.
*/
public MerchantTransaction purchaseDate(String purchaseDate) {
this.purchaseDate = purchaseDate;
return this;
}
/**
* Gets the status of transaction. Valid values are, Success: For approved transaction and Failure: For declined transaction.
*
* @return the status of transaction. Valid values are, Success: For approved transaction and Failure: For declined transaction.
**/
@XmlElement(name = "TransactionStatus")
public TransactionStatusEnum getTransactionStatus() {
return transactionStatus;
}
/**
* Sets the status of transaction. Valid values are, Success: For approved transaction and Failure: For declined transaction.
*
* @param transactionStatus the status of transaction. Valid values are, Success: For approved transaction and Failure: For declined transaction.
*/
public MerchantTransaction transactionStatus(TransactionStatusEnum transactionStatus) {
this.transactionStatus = transactionStatus;
return this;
}
/**
* Gets the six-digit approval code returned by payment API.
*
* @return the six-digit approval code returned by payment API.
**/
@XmlElement(name = "ApprovalCode")
public String getApprovalCode() {
return approvalCode;
}
/**
* Sets the six-digit approval code returned by payment API.
*
* @param approvalCode the six-digit approval code returned by payment API.
*/
public MerchantTransaction approvalCode(String approvalCode) {
this.approvalCode = approvalCode;
return this;
}
/**
* Gets the precheckout transaction id element of the PrecheckoutData.(This is not required for Standard Checkout)
*
* @return the precheckout transaction id element of the PrecheckoutData.(This is not required for Standard Checkout)
**/
@XmlElement(name = "PreCheckoutTransactionId")
public String getPreCheckoutTransactionId() {
return preCheckoutTransactionId;
}
/**
* Sets the precheckout transaction id element of the PrecheckoutData.(This is not required for Standard Checkout)
*
* @param preCheckoutTransactionId the precheckout transaction id element of the PrecheckoutData.(This is not required for Standard Checkout)
*/
public MerchantTransaction preCheckoutTransactionId(String preCheckoutTransactionId) {
this.preCheckoutTransactionId = preCheckoutTransactionId;
return this;
}
/**
* Gets the express checkout indicator. Set to true for express checkout
*
* @return the express checkout indicator. Set to true for express checkout
**/
@XmlElement(name = "ExpressCheckoutIndicator")
public Boolean getExpressCheckoutIndicator() {
return expressCheckoutIndicator;
}
/**
* Sets the express checkout indicator. Set to true for express checkout
*
* @param expressCheckoutIndicator the express checkout indicator. Set to true for express checkout
*/
public MerchantTransaction expressCheckoutIndicator(Boolean expressCheckoutIndicator) {
this.expressCheckoutIndicator = expressCheckoutIndicator;
return this;
}
/**
* Gets the ExtensionPoint for future enhancement.
*
* @return the ExtensionPoint for future enhancement.
**/
@XmlElement(name = "ExtensionPoint")
public ExtensionPoint getExtensionPoint() {
return extensionPoint;
}
/**
* Sets the ExtensionPoint for future enhancement.
*
* @param extensionPoint the ExtensionPoint for future enhancement.
*/
public MerchantTransaction extensionPoint(ExtensionPoint extensionPoint) {
this.extensionPoint = extensionPoint;
return this;
}
/**
* Returns true if the arguments are equal to each other and false
* otherwise. Consequently, if both arguments are null, true is returned and
* if exactly one argument is null, false is returned. Otherwise, equality
* is determined by using the equals method of the first argument.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MerchantTransaction merchantTransaction = (MerchantTransaction) o;
return Objects.equals(transactionId, merchantTransaction.transactionId) &&
Objects.equals(consumerKey, merchantTransaction.consumerKey) &&
Objects.equals(currency, merchantTransaction.currency) &&
Objects.equals(orderAmount, merchantTransaction.orderAmount) &&
Objects.equals(purchaseDate, merchantTransaction.purchaseDate) &&
Objects.equals(transactionStatus, merchantTransaction.transactionStatus) &&
Objects.equals(approvalCode, merchantTransaction.approvalCode) &&
Objects.equals(preCheckoutTransactionId, merchantTransaction.preCheckoutTransactionId) &&
Objects.equals(expressCheckoutIndicator, merchantTransaction.expressCheckoutIndicator) &&
Objects.equals(extensionPoint, merchantTransaction.extensionPoint);
}
/**
* Generates a hash code for a sequence of input values.
*/
@Override
public int hashCode() {
return Objects.hash(transactionId, consumerKey, currency, orderAmount, purchaseDate, transactionStatus, approvalCode, preCheckoutTransactionId, expressCheckoutIndicator, extensionPoint);
}
/**
* Returns the result of calling toString for a non-null argument and "null" for a null argument.
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MerchantTransaction {\n");
sb.append(" transactionId: ").append(toIndentedString(transactionId)).append("\n");
sb.append(" consumerKey: ").append(toIndentedString(consumerKey)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" orderAmount: ").append(toIndentedString(orderAmount)).append("\n");
sb.append(" purchaseDate: ").append(toIndentedString(purchaseDate)).append("\n");
sb.append(" transactionStatus: ").append(toIndentedString(transactionStatus)).append("\n");
sb.append(" approvalCode: ").append(toIndentedString(approvalCode)).append("\n");
sb.append(" preCheckoutTransactionId: ").append(toIndentedString(preCheckoutTransactionId)).append("\n");
sb.append(" expressCheckoutIndicator: ").append(toIndentedString(expressCheckoutIndicator)).append("\n");
sb.append(" extensionPoint: ").append(toIndentedString(extensionPoint)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy