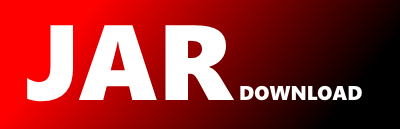
com.mastercard.merchant.checkout.model.Card Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mastercard-merchant-checkout Show documentation
Show all versions of mastercard-merchant-checkout Show documentation
Masterpass Merchant Checkout SDK on MasterCard Developer Zone (https://developer.mastercard.com)
package com.mastercard.merchant.checkout.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.mastercard.merchant.checkout.model.Address;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.gson.annotations.SerializedName;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.Root;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
/**
* This class contains card details for available cards.
**/
@Root(name = "Card")
@XmlRootElement (name = "Card")
public class Card {
@SerializedName("brandId")
@Element(name = "brandId", required = false)
@JsonProperty(value = "brandId", required = false)
private String brandId = null;
@SerializedName("brandName")
@Element(name = "brandName", required = false)
@JsonProperty(value = "brandName", required = false)
private String brandName = null;
@SerializedName("accountNumber")
@Element(name = "accountNumber", required = false)
@JsonProperty(value = "accountNumber", required = false)
private String accountNumber = null;
@SerializedName("cardHolderName")
@Element(name = "cardHolderName", required = false)
@JsonProperty(value = "cardHolderName", required = false)
private String cardHolderName = null;
@SerializedName("expiryMonth")
@Element(name = "expiryMonth", required = false)
@JsonProperty(value = "expiryMonth", required = false)
private Integer expiryMonth = null;
@SerializedName("expiryYear")
@Element(name = "expiryYear", required = false)
@JsonProperty(value = "expiryYear", required = false)
private Integer expiryYear = null;
@SerializedName("billingAddress")
@Element(name = "billingAddress", required = false)
@JsonProperty(value = "billingAddress", required = false)
private Address billingAddress = null;
@SerializedName("lastFour")
@Element(name = "lastFour", required = false)
@JsonProperty(value = "lastFour", required = false)
private String lastFour = null;
@SerializedName("cardType")
@Element(name = "cardType", required = false)
@JsonProperty(value = "cardType", required = false)
private String cardType = null;
/**
* Gets the card brand id referenced by Masterpass (master, amex, diners, discover, jcb, maestro, visa).
*
* @return the card brand id referenced by Masterpass (master, amex, diners, discover, jcb, maestro, visa).
**/
@XmlElement(name = "brandId")
public String getBrandId() {
return brandId;
}
/**
* Sets the card brand id referenced by Masterpass (master, amex, diners, discover, jcb, maestro, visa).
*
* @param brandId the card brand id referenced by Masterpass (master, amex, diners, discover, jcb, maestro, visa).
*/
public Card brandId(String brandId) {
this.brandId = brandId;
return this;
}
/**
* Gets the card brand full name (Mastercard, American Express, Diners Club, Discover, JCB, Maestro, Visa).
*
* @return the card brand full name (Mastercard, American Express, Diners Club, Discover, JCB, Maestro, Visa).
**/
@XmlElement(name = "brandName")
public String getBrandName() {
return brandName;
}
/**
* Sets the card brand full name (Mastercard, American Express, Diners Club, Discover, JCB, Maestro, Visa).
*
* @param brandName the card brand full name (Mastercard, American Express, Diners Club, Discover, JCB, Maestro, Visa).
*/
public Card brandName(String brandName) {
this.brandName = brandName;
return this;
}
/**
* Gets the card number, also known as Primary Account Number (PAN).
*
* @return the card number, also known as Primary Account Number (PAN).
**/
@XmlElement(name = "accountNumber")
public String getAccountNumber() {
return accountNumber;
}
/**
* Sets the card number, also known as Primary Account Number (PAN).
*
* @param accountNumber the card number, also known as Primary Account Number (PAN).
*/
public Card accountNumber(String accountNumber) {
this.accountNumber = accountNumber;
return this;
}
/**
* Gets the cardholder's name.
*
* @return the cardholder's name.
**/
@XmlElement(name = "cardHolderName")
public String getCardHolderName() {
return cardHolderName;
}
/**
* Sets the cardholder's name.
*
* @param cardHolderName the cardholder's name.
*/
public Card cardHolderName(String cardHolderName) {
this.cardHolderName = cardHolderName;
return this;
}
/**
* Gets the PAN expiration month, returned as a one or two digit integer. No leading zero is required for single digit months e.g. April is 4.
*
* @return the PAN expiration month, returned as a one or two digit integer. No leading zero is required for single digit months e.g. April is 4.
**/
@XmlElement(name = "expiryMonth")
public Integer getExpiryMonth() {
return expiryMonth;
}
/**
* Sets the PAN expiration month, returned as a one or two digit integer. No leading zero is required for single digit months e.g. April is 4.
*
* @param expiryMonth the PAN expiration month, returned as a one or two digit integer. No leading zero is required for single digit months e.g. April is 4.
*/
public Card expiryMonth(Integer expiryMonth) {
this.expiryMonth = expiryMonth;
return this;
}
/**
* Gets the PAN expiration year, returned as a four digit integer.
*
* @return the PAN expiration year, returned as a four digit integer.
**/
@XmlElement(name = "expiryYear")
public Integer getExpiryYear() {
return expiryYear;
}
/**
* Sets the PAN expiration year, returned as a four digit integer.
*
* @param expiryYear the PAN expiration year, returned as a four digit integer.
*/
public Card expiryYear(Integer expiryYear) {
this.expiryYear = expiryYear;
return this;
}
/**
* Gets the billing address associated with the card.
*
* @return the billing address associated with the card.
**/
@XmlElement(name = "billingAddress")
public Address getBillingAddress() {
return billingAddress;
}
/**
* Sets the billing address associated with the card.
*
* @param billingAddress the billing address associated with the card.
*/
public Card billingAddress(Address billingAddress) {
this.billingAddress = billingAddress;
return this;
}
/**
* Gets the last four digits of the PAN.
*
* @return the last four digits of the PAN.
**/
@XmlElement(name = "lastFour")
public String getLastFour() {
return lastFour;
}
/**
* Sets the last four digits of the PAN.
*
* @param lastFour the last four digits of the PAN.
*/
public Card lastFour(String lastFour) {
this.lastFour = lastFour;
return this;
}
/**
* Gets the card type (CREDIT/DEBIT) used in checkout. Available only for combo card transactions.
*
* @return the card type (CREDIT/DEBIT) used in checkout. Available only for combo card transactions.
**/
@XmlElement(name = "cardType")
public String getCardType() {
return cardType;
}
/**
* Sets the card type (CREDIT/DEBIT) used in checkout. Available only for combo card transactions.
*
* @param cardType the card type (CREDIT/DEBIT) used in checkout. Available only for combo card transactions.
*/
public Card cardType(String cardType) {
this.cardType = cardType;
return this;
}
/**
* Returns true if the arguments are equal to each other and false
* otherwise. Consequently, if both arguments are null, true is returned and
* if exactly one argument is null, false is returned. Otherwise, equality
* is determined by using the equals method of the first argument.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Card card = (Card) o;
return Objects.equals(brandId, card.brandId) &&
Objects.equals(brandName, card.brandName) &&
Objects.equals(accountNumber, card.accountNumber) &&
Objects.equals(cardHolderName, card.cardHolderName) &&
Objects.equals(expiryMonth, card.expiryMonth) &&
Objects.equals(expiryYear, card.expiryYear) &&
Objects.equals(billingAddress, card.billingAddress) &&
Objects.equals(lastFour, card.lastFour) &&
Objects.equals(cardType, card.cardType);
}
/**
* Generates a hash code for a sequence of input values.
*/
@Override
public int hashCode() {
return Objects.hash(brandId, brandName, accountNumber, cardHolderName, expiryMonth, expiryYear, billingAddress, lastFour, cardType);
}
/**
* Returns the result of calling toString for a non-null argument and "null" for a null argument.
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Card {\n");
sb.append(" brandId: ").append(toIndentedString(brandId)).append("\n");
sb.append(" brandName: ").append(toIndentedString(brandName)).append("\n");
sb.append(" accountNumber: ").append(toIndentedString(accountNumber)).append("\n");
sb.append(" cardHolderName: ").append(toIndentedString(cardHolderName)).append("\n");
sb.append(" expiryMonth: ").append(toIndentedString(expiryMonth)).append("\n");
sb.append(" expiryYear: ").append(toIndentedString(expiryYear)).append("\n");
sb.append(" billingAddress: ").append(toIndentedString(billingAddress)).append("\n");
sb.append(" lastFour: ").append(toIndentedString(lastFour)).append("\n");
sb.append(" cardType: ").append(toIndentedString(cardType)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy