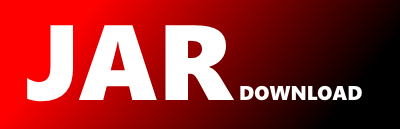
com.mastercard.merchant.checkout.model.Cryptogram Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mastercard-merchant-checkout Show documentation
Show all versions of mastercard-merchant-checkout Show documentation
Masterpass Merchant Checkout SDK on MasterCard Developer Zone (https://developer.mastercard.com)
package com.mastercard.merchant.checkout.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.gson.annotations.SerializedName;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.Root;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
/**
* This class contains the cryptogram generated by the consumer's Masterpass wallet.
**/
@Root(name = "Cryptogram")
@XmlRootElement (name = "Cryptogram")
public class Cryptogram {
@SerializedName("cryptoValue")
@Element(name = "cryptoValue", required = false)
@JsonProperty(value = "cryptoValue", required = false)
private String cryptoValue = null;
public enum CryptoTypeEnum {
@SerializedName("ICC")
ICC("ICC"),
@SerializedName("UCAF")
UCAF("UCAF"),
@SerializedName("TAVV")
TAVV("TAVV");
private String value;
CryptoTypeEnum(String value) {
this.value = value;
}
@Override
public String toString() {
return value;
}
}
@SerializedName("cryptoType")
@Element(name = "cryptoType", required = false)
@JsonProperty(value = "cryptoType", required = false)
private CryptoTypeEnum cryptoType = null;
@SerializedName("unpredictableNumber")
@Element(name = "unpredictableNumber", required = false)
@JsonProperty(value = "unpredictableNumber", required = false)
private String unpredictableNumber = null;
@SerializedName("eci")
@Element(name = "eci", required = false)
@JsonProperty(value = "eci", required = false)
private String eci = null;
/**
* Gets the cryptogram generated by the consumer's Masterpass wallet
*
* @return the cryptogram generated by the consumer's Masterpass wallet
**/
@XmlElement(name = "cryptoValue")
public String getCryptoValue() {
return cryptoValue;
}
/**
* Sets the cryptogram generated by the consumer's Masterpass wallet
*
* @param cryptoValue the cryptogram generated by the consumer's Masterpass wallet
*/
public Cryptogram cryptoValue(String cryptoValue) {
this.cryptoValue = cryptoValue;
return this;
}
/**
* Gets the type of cryptogram generated by the consumers Masterpass wallet. Masterpass passes the most secure selection (ICC) if the merchant or service provider has indicated they can accept both types (UCAF, ICC).
*
* @return the type of cryptogram generated by the consumers Masterpass wallet. Masterpass passes the most secure selection (ICC) if the merchant or service provider has indicated they can accept both types (UCAF, ICC).
**/
@XmlElement(name = "cryptoType")
public CryptoTypeEnum getCryptoType() {
return cryptoType;
}
/**
* Sets the type of cryptogram generated by the consumers Masterpass wallet. Masterpass passes the most secure selection (ICC) if the merchant or service provider has indicated they can accept both types (UCAF, ICC).
*
* @param cryptoType the type of cryptogram generated by the consumers Masterpass wallet. Masterpass passes the most secure selection (ICC) if the merchant or service provider has indicated they can accept both types (UCAF, ICC).
*/
public Cryptogram cryptoType(CryptoTypeEnum cryptoType) {
this.cryptoType = cryptoType;
return this;
}
/**
* Gets the Base64 encoded unpredictable number. EMV quality random number generated by the merchant, service provider, or, Masterpass (if null).
*
* @return the Base64 encoded unpredictable number. EMV quality random number generated by the merchant, service provider, or, Masterpass (if null).
**/
@XmlElement(name = "unpredictableNumber")
public String getUnpredictableNumber() {
return unpredictableNumber;
}
/**
* Sets the Base64 encoded unpredictable number. EMV quality random number generated by the merchant, service provider, or, Masterpass (if null).
*
* @param unpredictableNumber the Base64 encoded unpredictable number. EMV quality random number generated by the merchant, service provider, or, Masterpass (if null).
*/
public Cryptogram unpredictableNumber(String unpredictableNumber) {
this.unpredictableNumber = unpredictableNumber;
return this;
}
/**
* Gets the electronic commerce indicator (ECI) value (DE 48 SE 42 position 3). Present only when crypto type is UCAF. For Mastercard brand cards, value is: 02 Authenticated by ACS (Card Issuer Liability)
*
* @return the electronic commerce indicator (ECI) value (DE 48 SE 42 position 3). Present only when crypto type is UCAF. For Mastercard brand cards, value is: 02 Authenticated by ACS (Card Issuer Liability)
**/
@XmlElement(name = "eci")
public String getEci() {
return eci;
}
/**
* Sets the electronic commerce indicator (ECI) value (DE 48 SE 42 position 3). Present only when crypto type is UCAF. For Mastercard brand cards, value is: 02 Authenticated by ACS (Card Issuer Liability)
*
* @param eci the electronic commerce indicator (ECI) value (DE 48 SE 42 position 3). Present only when crypto type is UCAF. For Mastercard brand cards, value is: 02 Authenticated by ACS (Card Issuer Liability)
*/
public Cryptogram eci(String eci) {
this.eci = eci;
return this;
}
/**
* Returns true if the arguments are equal to each other and false
* otherwise. Consequently, if both arguments are null, true is returned and
* if exactly one argument is null, false is returned. Otherwise, equality
* is determined by using the equals method of the first argument.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Cryptogram cryptogram = (Cryptogram) o;
return Objects.equals(cryptoValue, cryptogram.cryptoValue) &&
Objects.equals(cryptoType, cryptogram.cryptoType) &&
Objects.equals(unpredictableNumber, cryptogram.unpredictableNumber) &&
Objects.equals(eci, cryptogram.eci);
}
/**
* Generates a hash code for a sequence of input values.
*/
@Override
public int hashCode() {
return Objects.hash(cryptoValue, cryptoType, unpredictableNumber, eci);
}
/**
* Returns the result of calling toString for a non-null argument and "null" for a null argument.
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Cryptogram {\n");
sb.append(" cryptoValue: ").append(toIndentedString(cryptoValue)).append("\n");
sb.append(" cryptoType: ").append(toIndentedString(cryptoType)).append("\n");
sb.append(" unpredictableNumber: ").append(toIndentedString(unpredictableNumber)).append("\n");
sb.append(" eci: ").append(toIndentedString(eci)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy