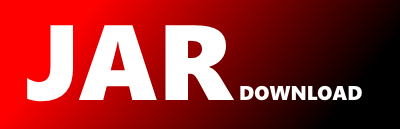
com.mastercard.merchant.checkout.model.ExpressCheckoutRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mastercard-merchant-checkout Show documentation
Show all versions of mastercard-merchant-checkout Show documentation
Masterpass Merchant Checkout SDK on MasterCard Developer Zone (https://developer.mastercard.com)
package com.mastercard.merchant.checkout.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.gson.annotations.SerializedName;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.Root;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
/**
* This class contains various methods to set express checkout request parameters required for ExpressCheckoutApi.
**/
@Root(name = "ExpressCheckoutRequest")
@XmlRootElement (name = "ExpressCheckoutRequest")
public class ExpressCheckoutRequest {
@SerializedName("checkoutId")
@Element(name = "checkoutId")
@JsonProperty(value = "checkoutId")
private String checkoutId = null;
@SerializedName("pairingId")
@Element(name = "pairingId")
@JsonProperty(value = "pairingId")
private String pairingId = null;
@SerializedName("preCheckoutTransactionId")
@Element(name = "preCheckoutTransactionId")
@JsonProperty(value = "preCheckoutTransactionId")
private String preCheckoutTransactionId = null;
@SerializedName("amount")
@Element(name = "amount")
@JsonProperty(value = "amount")
private Double amount = null;
@SerializedName("currency")
@Element(name = "currency")
@JsonProperty(value = "currency")
private String currency = null;
@SerializedName("cardId")
@Element(name = "cardId")
@JsonProperty(value = "cardId")
private String cardId = null;
@SerializedName("shippingAddressId")
@Element(name = "shippingAddressId", required = false)
@JsonProperty(value = "shippingAddressId", required = false)
private String shippingAddressId = null;
@SerializedName("digitalGoods")
@Element(name = "digitalGoods", required = false)
@JsonProperty(value = "digitalGoods", required = false)
private Boolean digitalGoods = null;
@SerializedName("pspId")
@Element(name = "pspId", required = false)
@JsonProperty(value = "pspId", required = false)
private String pspId = null;
/**
* Gets the merchant Checkout identifier.
*
* @return the merchant Checkout identifier.
**/
@XmlElement(name = "checkoutId")
public String getCheckoutId() {
return checkoutId;
}
/**
* Sets the merchant Checkout identifier.
*
* @param checkoutId the merchant Checkout identifier.
*/
public ExpressCheckoutRequest checkoutId(String checkoutId) {
this.checkoutId = checkoutId;
return this;
}
/**
* Gets the unique pairing token identifier used to fetch card and address data from a wallet that is paired with a merchant during Express Checkout.
*
* @return the unique pairing token identifier used to fetch card and address data from a wallet that is paired with a merchant during Express Checkout.
**/
@XmlElement(name = "pairingId")
public String getPairingId() {
return pairingId;
}
/**
* Sets the unique pairing token identifier used to fetch card and address data from a wallet that is paired with a merchant during Express Checkout.
*
* @param pairingId the unique pairing token identifier used to fetch card and address data from a wallet that is paired with a merchant during Express Checkout.
*/
public ExpressCheckoutRequest pairingId(String pairingId) {
this.pairingId = pairingId;
return this;
}
/**
* Gets the precheckout identifier from PreCheckoutApi response.
*
* @return the precheckout identifier from PreCheckoutApi response.
**/
@XmlElement(name = "preCheckoutTransactionId")
public String getPreCheckoutTransactionId() {
return preCheckoutTransactionId;
}
/**
* Sets the precheckout identifier from PreCheckoutApi response.
*
* @param preCheckoutTransactionId the precheckout identifier from PreCheckoutApi response.
*/
public ExpressCheckoutRequest preCheckoutTransactionId(String preCheckoutTransactionId) {
this.preCheckoutTransactionId = preCheckoutTransactionId;
return this;
}
/**
* Gets the transaction amount as a decimal (100.50 for $100.50).
*
* @return the transaction amount as a decimal (100.50 for $100.50).
**/
@XmlElement(name = "amount")
public Double getAmount() {
return amount;
}
/**
* Sets the transaction amount as a decimal (100.50 for $100.50).
*
* @param amount the transaction amount as a decimal (100.50 for $100.50).
*/
public ExpressCheckoutRequest amount(Double amount) {
this.amount = amount;
return this;
}
/**
* Gets the ISO-4217 code for currency of the transaction.
*
* @return the ISO-4217 code for currency of the transaction.
**/
@XmlElement(name = "currency")
public String getCurrency() {
return currency;
}
/**
* Sets the ISO-4217 code for currency of the transaction.
*
* @param currency the ISO-4217 code for currency of the transaction.
*/
public ExpressCheckoutRequest currency(String currency) {
this.currency = currency;
return this;
}
/**
* Gets the card identifier from PreCheckoutApi response.
*
* @return the card identifier from PreCheckoutApi response.
**/
@XmlElement(name = "cardId")
public String getCardId() {
return cardId;
}
/**
* Sets the card identifier from PreCheckoutApi response.
*
* @param cardId the card identifier from PreCheckoutApi response.
*/
public ExpressCheckoutRequest cardId(String cardId) {
this.cardId = cardId;
return this;
}
/**
* Gets the shippingAddress identifier from PreCheckoutApi response.
*
* @return the shippingAddress identifier from PreCheckoutApi response.
**/
@XmlElement(name = "shippingAddressId")
public String getShippingAddressId() {
return shippingAddressId;
}
/**
* Sets the shippingAddress identifier from PreCheckoutApi response.
*
* @param shippingAddressId the shippingAddress identifier from PreCheckoutApi response.
*/
public ExpressCheckoutRequest shippingAddressId(String shippingAddressId) {
this.shippingAddressId = shippingAddressId;
return this;
}
/**
* Gets the flag to indicate digital goods are being purchased so a shipping address is not required for the transaction.
*
* @return the flag to indicate digital goods are being purchased so a shipping address is not required for the transaction.
**/
@XmlElement(name = "digitalGoods")
public Boolean getDigitalGoods() {
return digitalGoods;
}
/**
* Sets the flag to indicate digital goods are being purchased so a shipping address is not required for the transaction.
*
* @param digitalGoods the flag to indicate digital goods are being purchased so a shipping address is not required for the transaction.
*/
public ExpressCheckoutRequest digitalGoods(Boolean digitalGoods) {
this.digitalGoods = digitalGoods;
return this;
}
/**
* Gets the pspId passed by merchant to select PSP for pushing payment data.
*
* @return the pspId passed by merchant to select PSP for pushing payment data.
**/
@XmlElement(name = "pspId")
public String getPspId() {
return pspId;
}
/**
* Sets the pspId passed by merchant to select PSP for pushing payment data.
*
* @param pspId the pspId passed by merchant to select PSP for pushing payment data.
*/
public ExpressCheckoutRequest pspId(String pspId) {
this.pspId = pspId;
return this;
}
/**
* Returns true if the arguments are equal to each other and false
* otherwise. Consequently, if both arguments are null, true is returned and
* if exactly one argument is null, false is returned. Otherwise, equality
* is determined by using the equals method of the first argument.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExpressCheckoutRequest expressCheckoutRequest = (ExpressCheckoutRequest) o;
return Objects.equals(checkoutId, expressCheckoutRequest.checkoutId) &&
Objects.equals(pairingId, expressCheckoutRequest.pairingId) &&
Objects.equals(preCheckoutTransactionId, expressCheckoutRequest.preCheckoutTransactionId) &&
Objects.equals(amount, expressCheckoutRequest.amount) &&
Objects.equals(currency, expressCheckoutRequest.currency) &&
Objects.equals(cardId, expressCheckoutRequest.cardId) &&
Objects.equals(shippingAddressId, expressCheckoutRequest.shippingAddressId) &&
Objects.equals(digitalGoods, expressCheckoutRequest.digitalGoods) &&
Objects.equals(pspId, expressCheckoutRequest.pspId);
}
/**
* Generates a hash code for a sequence of input values.
*/
@Override
public int hashCode() {
return Objects.hash(checkoutId, pairingId, preCheckoutTransactionId, amount, currency, cardId, shippingAddressId, digitalGoods, pspId);
}
/**
* Returns the result of calling toString for a non-null argument and "null" for a null argument.
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ExpressCheckoutRequest {\n");
sb.append(" checkoutId: ").append(toIndentedString(checkoutId)).append("\n");
sb.append(" pairingId: ").append(toIndentedString(pairingId)).append("\n");
sb.append(" preCheckoutTransactionId: ").append(toIndentedString(preCheckoutTransactionId)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" cardId: ").append(toIndentedString(cardId)).append("\n");
sb.append(" shippingAddressId: ").append(toIndentedString(shippingAddressId)).append("\n");
sb.append(" digitalGoods: ").append(toIndentedString(digitalGoods)).append("\n");
sb.append(" pspId: ").append(toIndentedString(pspId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy