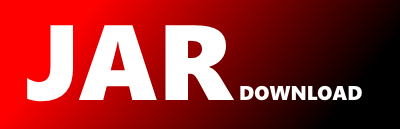
com.mastercard.merchant.checkout.model.Postback Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mastercard-merchant-checkout Show documentation
Show all versions of mastercard-merchant-checkout Show documentation
Masterpass Merchant Checkout SDK on MasterCard Developer Zone (https://developer.mastercard.com)
package com.mastercard.merchant.checkout.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.Date;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.gson.annotations.SerializedName;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.Root;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
/**
* This class contains different methods to send transaction details to Masterpass using PostbackApi.
**/
@Root(name = "Postback")
@XmlRootElement (name = "Postback")
public class Postback {
@SerializedName("transactionId")
@Element(name = "transactionId")
@JsonProperty(value = "transactionId")
private String transactionId = null;
@SerializedName("currency")
@Element(name = "currency")
@JsonProperty(value = "currency")
private String currency = null;
@SerializedName("amount")
@Element(name = "amount")
@JsonProperty(value = "amount")
private Double amount = null;
@SerializedName("paymentSuccessful")
@Element(name = "paymentSuccessful")
@JsonProperty(value = "paymentSuccessful")
private Boolean paymentSuccessful = null;
@SerializedName("paymentCode")
@Element(name = "paymentCode")
@JsonProperty(value = "paymentCode")
private String paymentCode = null;
@SerializedName("paymentDate")
@Element(name = "paymentDate")
@JsonProperty(value = "paymentDate")
private Date paymentDate = null;
@SerializedName("preCheckoutTransactionId")
@Element(name = "preCheckoutTransactionId", required = false)
@JsonProperty(value = "preCheckoutTransactionId", required = false)
private String preCheckoutTransactionId = null;
/**
* Gets the transaction identifiers. Identifies the transaction for which to return the consumer’s payment data. This is the oauth_verifier value sent by Masterpass in callback URL after the Masterpass user interface is closed.
*
* @return the transaction identifiers. Identifies the transaction for which to return the consumer’s payment data. This is the oauth_verifier value sent by Masterpass in callback URL after the Masterpass user interface is closed.
**/
@XmlElement(name = "transactionId")
public String getTransactionId() {
return transactionId;
}
/**
* Sets the transaction identifiers. Identifies the transaction for which to return the consumer’s payment data. This is the oauth_verifier value sent by Masterpass in callback URL after the Masterpass user interface is closed.
*
* @param transactionId the transaction identifiers. Identifies the transaction for which to return the consumer’s payment data. This is the oauth_verifier value sent by Masterpass in callback URL after the Masterpass user interface is closed.
*/
public Postback transactionId(String transactionId) {
this.transactionId = transactionId;
return this;
}
/**
* Gets the ISO-4217 code for currency of the transaction.
*
* @return the ISO-4217 code for currency of the transaction.
**/
@XmlElement(name = "currency")
public String getCurrency() {
return currency;
}
/**
* Sets the ISO-4217 code for currency of the transaction.
*
* @param currency the ISO-4217 code for currency of the transaction.
*/
public Postback currency(String currency) {
this.currency = currency;
return this;
}
/**
* Gets the transaction amount as a decimal (100.50 for $100.50).
*
* @return the transaction amount as a decimal (100.50 for $100.50).
**/
@XmlElement(name = "amount")
public Double getAmount() {
return amount;
}
/**
* Sets the transaction amount as a decimal (100.50 for $100.50).
*
* @param amount the transaction amount as a decimal (100.50 for $100.50).
*/
public Postback amount(Double amount) {
this.amount = amount;
return this;
}
/**
* Gets the payment status indicator. It is set to true if payment successful with payment processor else false.
*
* @return the payment status indicator. It is set to true if payment successful with payment processor else false.
**/
@XmlElement(name = "paymentSuccessful")
public Boolean getPaymentSuccessful() {
return paymentSuccessful;
}
/**
* Sets the payment status indicator. It is set to true if payment successful with payment processor else false.
*
* @param paymentSuccessful the payment status indicator. It is set to true if payment successful with payment processor else false.
*/
public Postback paymentSuccessful(Boolean paymentSuccessful) {
this.paymentSuccessful = paymentSuccessful;
return this;
}
/**
* Gets the six-digit approval code returned by payment API.
*
* @return the six-digit approval code returned by payment API.
**/
@XmlElement(name = "paymentCode")
public String getPaymentCode() {
return paymentCode;
}
/**
* Sets the six-digit approval code returned by payment API.
*
* @param paymentCode the six-digit approval code returned by payment API.
*/
public Postback paymentCode(String paymentCode) {
this.paymentCode = paymentCode;
return this;
}
/**
* Gets the date of purchase.
*
* @return the date of purchase.
**/
@XmlElement(name = "paymentDate")
public Date getPaymentDate() {
return paymentDate;
}
/**
* Sets the date of purchase.
*
* @param paymentDate the date of purchase.
*/
public Postback paymentDate(Date paymentDate) {
this.paymentDate = paymentDate;
return this;
}
/**
* Gets the preCheckoutTransactionId from the ExpressCheckout response.
*
* @return the preCheckoutTransactionId from the ExpressCheckout response.
**/
@XmlElement(name = "preCheckoutTransactionId")
public String getPreCheckoutTransactionId() {
return preCheckoutTransactionId;
}
/**
* Sets the preCheckoutTransactionId from the ExpressCheckout response.
*
* @param preCheckoutTransactionId the preCheckoutTransactionId from the ExpressCheckout response.
*/
public Postback preCheckoutTransactionId(String preCheckoutTransactionId) {
this.preCheckoutTransactionId = preCheckoutTransactionId;
return this;
}
/**
* Returns true if the arguments are equal to each other and false
* otherwise. Consequently, if both arguments are null, true is returned and
* if exactly one argument is null, false is returned. Otherwise, equality
* is determined by using the equals method of the first argument.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Postback postback = (Postback) o;
return Objects.equals(transactionId, postback.transactionId) &&
Objects.equals(currency, postback.currency) &&
Objects.equals(amount, postback.amount) &&
Objects.equals(paymentSuccessful, postback.paymentSuccessful) &&
Objects.equals(paymentCode, postback.paymentCode) &&
Objects.equals(paymentDate, postback.paymentDate) &&
Objects.equals(preCheckoutTransactionId, postback.preCheckoutTransactionId);
}
/**
* Generates a hash code for a sequence of input values.
*/
@Override
public int hashCode() {
return Objects.hash(transactionId, currency, amount, paymentSuccessful, paymentCode, paymentDate, preCheckoutTransactionId);
}
/**
* Returns the result of calling toString for a non-null argument and "null" for a null argument.
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Postback {\n");
sb.append(" transactionId: ").append(toIndentedString(transactionId)).append("\n");
sb.append(" currency: ").append(toIndentedString(currency)).append("\n");
sb.append(" amount: ").append(toIndentedString(amount)).append("\n");
sb.append(" paymentSuccessful: ").append(toIndentedString(paymentSuccessful)).append("\n");
sb.append(" paymentCode: ").append(toIndentedString(paymentCode)).append("\n");
sb.append(" paymentDate: ").append(toIndentedString(paymentDate)).append("\n");
sb.append(" preCheckoutTransactionId: ").append(toIndentedString(preCheckoutTransactionId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy