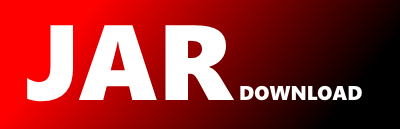
com.mastercard.merchant.checkout.model.PreCheckoutData Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mastercard-merchant-checkout Show documentation
Show all versions of mastercard-merchant-checkout Show documentation
Masterpass Merchant Checkout SDK on MasterCard Developer Zone (https://developer.mastercard.com)
package com.mastercard.merchant.checkout.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.mastercard.merchant.checkout.model.ContactInfo;
import com.mastercard.merchant.checkout.model.PreCheckoutCard;
import com.mastercard.merchant.checkout.model.ShippingAddress;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.*;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.gson.annotations.SerializedName;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.Root;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
/**
* This class contains different methods for different types of precheckout data like card, contact and shipping address details.
**/
@Root(name = "PreCheckoutData")
@XmlRootElement (name = "PreCheckoutData")
public class PreCheckoutData {
@SerializedName("cards")
@ElementList(name = "cards" , inline = true, entry = "cards", required = false)
@JsonProperty(value = "cards" , required = false)
private List cards = new ArrayList();
@SerializedName("shippingAddresses")
@ElementList(name = "shippingAddresses" , inline = true, entry = "shippingAddresses", required = false)
@JsonProperty(value = "shippingAddresses" , required = false)
private List shippingAddresses = new ArrayList();
@SerializedName("contactInfo")
@Element(name = "contactInfo", required = false)
@JsonProperty(value = "contactInfo", required = false)
private ContactInfo contactInfo = null;
@SerializedName("preCheckoutTransactionId")
@Element(name = "preCheckoutTransactionId", required = false)
@JsonProperty(value = "preCheckoutTransactionId", required = false)
private String preCheckoutTransactionId = null;
@SerializedName("consumerWalletId")
@Element(name = "consumerWalletId", required = false)
@JsonProperty(value = "consumerWalletId", required = false)
private String consumerWalletId = null;
@SerializedName("walletName")
@Element(name = "walletName", required = false)
@JsonProperty(value = "walletName", required = false)
private String walletName = null;
@SerializedName("pairingId")
@Element(name = "pairingId", required = false)
@JsonProperty(value = "pairingId", required = false)
private String pairingId = null;
/**
* Gets the cards in the paired wallet.
*
* @return the cards in the paired wallet.
**/
@XmlElement(name = "cards")
public List getCards() {
return cards;
}
/**
* Sets the cards in the paired wallet.
*
* @param cards the cards in the paired wallet.
*/
public PreCheckoutData cards(List cards) {
this.cards = cards;
return this;
}
/**
* Sets the cards in the paired wallet.
*
* @param cards the cards in the paired wallet. Adds cards elements in a list.
* This is a optional method to add elements into the list.
*/
public PreCheckoutData cards(PreCheckoutCard cards) {
this.cards.add(cards);
return this;
}
/**
* Gets the shipping addresses details.
*
* @return the shipping addresses details.
**/
@XmlElement(name = "shippingAddresses")
public List getShippingAddresses() {
return shippingAddresses;
}
/**
* Sets the shipping addresses details.
*
* @param shippingAddresses the shipping addresses details.
*/
public PreCheckoutData shippingAddresses(List shippingAddresses) {
this.shippingAddresses = shippingAddresses;
return this;
}
/**
* Sets the shipping addresses details.
*
* @param shippingAddresses the shipping addresses details. Adds shippingAddresses elements in a list.
* This is a optional method to add elements into the list.
*/
public PreCheckoutData shippingAddresses(ShippingAddress shippingAddresses) {
this.shippingAddresses.add(shippingAddresses);
return this;
}
/**
* Gets the contact info details.
*
* @return the contact info details.
**/
@XmlElement(name = "contactInfo")
public ContactInfo getContactInfo() {
return contactInfo;
}
/**
* Sets the contact info details.
*
* @param contactInfo the contact info details.
*/
public PreCheckoutData contactInfo(ContactInfo contactInfo) {
this.contactInfo = contactInfo;
return this;
}
/**
* Gets the preCheckout transaction id.
*
* @return the preCheckout transaction id.
**/
@XmlElement(name = "preCheckoutTransactionId")
public String getPreCheckoutTransactionId() {
return preCheckoutTransactionId;
}
/**
* Sets the preCheckout transaction id.
*
* @param preCheckoutTransactionId the preCheckout transaction id.
*/
public PreCheckoutData preCheckoutTransactionId(String preCheckoutTransactionId) {
this.preCheckoutTransactionId = preCheckoutTransactionId;
return this;
}
/**
* Gets the consumer's wallet id.
*
* @return the consumer's wallet id.
**/
@XmlElement(name = "consumerWalletId")
public String getConsumerWalletId() {
return consumerWalletId;
}
/**
* Sets the consumer's wallet id.
*
* @param consumerWalletId the consumer's wallet id.
*/
public PreCheckoutData consumerWalletId(String consumerWalletId) {
this.consumerWalletId = consumerWalletId;
return this;
}
/**
* Gets the wallet name.
*
* @return the wallet name.
**/
@XmlElement(name = "walletName")
public String getWalletName() {
return walletName;
}
/**
* Sets the wallet name.
*
* @param walletName the wallet name.
*/
public PreCheckoutData walletName(String walletName) {
this.walletName = walletName;
return this;
}
/**
* Gets the new pairing token id.
*
* @return the new pairing token id.
**/
@XmlElement(name = "pairingId")
public String getPairingId() {
return pairingId;
}
/**
* Sets the new pairing token id.
*
* @param pairingId the new pairing token id.
*/
public PreCheckoutData pairingId(String pairingId) {
this.pairingId = pairingId;
return this;
}
/**
* Returns true if the arguments are equal to each other and false
* otherwise. Consequently, if both arguments are null, true is returned and
* if exactly one argument is null, false is returned. Otherwise, equality
* is determined by using the equals method of the first argument.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
PreCheckoutData preCheckoutData = (PreCheckoutData) o;
return Objects.equals(cards, preCheckoutData.cards) &&
Objects.equals(shippingAddresses, preCheckoutData.shippingAddresses) &&
Objects.equals(contactInfo, preCheckoutData.contactInfo) &&
Objects.equals(preCheckoutTransactionId, preCheckoutData.preCheckoutTransactionId) &&
Objects.equals(consumerWalletId, preCheckoutData.consumerWalletId) &&
Objects.equals(walletName, preCheckoutData.walletName) &&
Objects.equals(pairingId, preCheckoutData.pairingId);
}
/**
* Generates a hash code for a sequence of input values.
*/
@Override
public int hashCode() {
return Objects.hash(cards, shippingAddresses, contactInfo, preCheckoutTransactionId, consumerWalletId, walletName, pairingId);
}
/**
* Returns the result of calling toString for a non-null argument and "null" for a null argument.
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class PreCheckoutData {\n");
sb.append(" cards: ").append(toIndentedString(cards)).append("\n");
sb.append(" shippingAddresses: ").append(toIndentedString(shippingAddresses)).append("\n");
sb.append(" contactInfo: ").append(toIndentedString(contactInfo)).append("\n");
sb.append(" preCheckoutTransactionId: ").append(toIndentedString(preCheckoutTransactionId)).append("\n");
sb.append(" consumerWalletId: ").append(toIndentedString(consumerWalletId)).append("\n");
sb.append(" walletName: ").append(toIndentedString(walletName)).append("\n");
sb.append(" pairingId: ").append(toIndentedString(pairingId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy