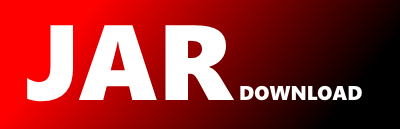
com.mastercard.merchant.checkout.model.ShippingAddress Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mastercard-merchant-checkout Show documentation
Show all versions of mastercard-merchant-checkout Show documentation
Masterpass Merchant Checkout SDK on MasterCard Developer Zone (https://developer.mastercard.com)
package com.mastercard.merchant.checkout.model;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.mastercard.merchant.checkout.model.RecipientInfo;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.google.gson.annotations.SerializedName;
import org.simpleframework.xml.Element;
import org.simpleframework.xml.ElementList;
import org.simpleframework.xml.Root;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
/**
* This class contains shipping address information.
**/
@Root(name = "ShippingAddress")
@XmlRootElement (name = "ShippingAddress")
public class ShippingAddress {
@SerializedName("recipientInfo")
@Element(name = "recipientInfo", required = false)
@JsonProperty(value = "recipientInfo", required = false)
private RecipientInfo recipientInfo = null;
@SerializedName("addressId")
@Element(name = "addressId", required = false)
@JsonProperty(value = "addressId", required = false)
private String addressId = null;
@SerializedName("default")
@Element(name = "default", required = false)
@JsonProperty(value = "default", required = false)
private Boolean _default = null;
@SerializedName("city")
@Element(name = "city", required = false)
@JsonProperty(value = "city", required = false)
private String city = null;
@SerializedName("country")
@Element(name = "country", required = false)
@JsonProperty(value = "country", required = false)
private String country = null;
@SerializedName("subdivision")
@Element(name = "subdivision", required = false)
@JsonProperty(value = "subdivision", required = false)
private String subdivision = null;
@SerializedName("line1")
@Element(name = "line1", required = false)
@JsonProperty(value = "line1", required = false)
private String line1 = null;
@SerializedName("line2")
@Element(name = "line2", required = false)
@JsonProperty(value = "line2", required = false)
private String line2 = null;
@SerializedName("line3")
@Element(name = "line3", required = false)
@JsonProperty(value = "line3", required = false)
private String line3 = null;
@SerializedName("line4")
@Element(name = "line4", required = false)
@JsonProperty(value = "line4", required = false)
private String line4 = null;
@SerializedName("line5")
@Element(name = "line5", required = false)
@JsonProperty(value = "line5", required = false)
private String line5 = null;
@SerializedName("postalCode")
@Element(name = "postalCode", required = false)
@JsonProperty(value = "postalCode", required = false)
private String postalCode = null;
/**
* Gets the recipient information.
*
* @return the recipient information.
**/
@XmlElement(name = "recipientInfo")
public RecipientInfo getRecipientInfo() {
return recipientInfo;
}
/**
* Sets the recipient information.
*
* @param recipientInfo the recipient information.
*/
public ShippingAddress recipientInfo(RecipientInfo recipientInfo) {
this.recipientInfo = recipientInfo;
return this;
}
/**
* Gets the address id.
*
* @return the address id.
**/
@XmlElement(name = "addressId")
public String getAddressId() {
return addressId;
}
/**
* Sets the address id.
*
* @param addressId the address id.
*/
public ShippingAddress addressId(String addressId) {
this.addressId = addressId;
return this;
}
/**
* Gets the default selection.
*
* @return the default selection.
**/
@XmlElement(name = "default")
public Boolean getDefault() {
return _default;
}
/**
* Sets the default selection.
*
* @param _default the default selection.
*/
public ShippingAddress _default(Boolean _default) {
this._default = _default;
return this;
}
/**
* Gets the cardholder's city.
*
* @return the cardholder's city.
**/
@XmlElement(name = "city")
public String getCity() {
return city;
}
/**
* Sets the cardholder's city.
*
* @param city the cardholder's city.
*/
public ShippingAddress city(String city) {
this.city = city;
return this;
}
/**
* Gets the cardholder's country as defined by ISO 3166-1 alpha-2 digit country codes; for example, US is the United States, AU is Australia, CA is Canada, GB is the United Kingdom, and so on.
*
* @return the cardholder's country as defined by ISO 3166-1 alpha-2 digit country codes; for example, US is the United States, AU is Australia, CA is Canada, GB is the United Kingdom, and so on.
**/
@XmlElement(name = "country")
public String getCountry() {
return country;
}
/**
* Sets the cardholder's country as defined by ISO 3166-1 alpha-2 digit country codes; for example, US is the United States, AU is Australia, CA is Canada, GB is the United Kingdom, and so on.
*
* @param country the cardholder's country as defined by ISO 3166-1 alpha-2 digit country codes; for example, US is the United States, AU is Australia, CA is Canada, GB is the United Kingdom, and so on.
*/
public ShippingAddress country(String country) {
this.country = country;
return this;
}
/**
* Gets the cardholder's country's subdivision as defined by ISO 3166-1 alpha-2 digit code; for example, US-VA is Virginia, US-OH is Ohio, and so on.
*
* @return the cardholder's country's subdivision as defined by ISO 3166-1 alpha-2 digit code; for example, US-VA is Virginia, US-OH is Ohio, and so on.
**/
@XmlElement(name = "subdivision")
public String getSubdivision() {
return subdivision;
}
/**
* Sets the cardholder's country's subdivision as defined by ISO 3166-1 alpha-2 digit code; for example, US-VA is Virginia, US-OH is Ohio, and so on.
*
* @param subdivision the cardholder's country's subdivision as defined by ISO 3166-1 alpha-2 digit code; for example, US-VA is Virginia, US-OH is Ohio, and so on.
*/
public ShippingAddress subdivision(String subdivision) {
this.subdivision = subdivision;
return this;
}
/**
* Gets the address in line 1 is used for the street number and the street name.
*
* @return the address in line 1 is used for the street number and the street name.
**/
@XmlElement(name = "line1")
public String getLine1() {
return line1;
}
/**
* Sets the address in line 1 is used for the street number and the street name.
*
* @param line1 the address in line 1 is used for the street number and the street name.
*/
public ShippingAddress line1(String line1) {
this.line1 = line1;
return this;
}
/**
* Gets the address in line 2 is used for the apartment number, suite Number, and so on.
*
* @return the address in line 2 is used for the apartment number, suite Number, and so on.
**/
@XmlElement(name = "line2")
public String getLine2() {
return line2;
}
/**
* Sets the address in line 2 is used for the apartment number, suite Number, and so on.
*
* @param line2 the address in line 2 is used for the apartment number, suite Number, and so on.
*/
public ShippingAddress line2(String line2) {
this.line2 = line2;
return this;
}
/**
* Gets the address in line 3 is used to enter the remaining address information if it does not fit in lines 1 and 2.
*
* @return the address in line 3 is used to enter the remaining address information if it does not fit in lines 1 and 2.
**/
@XmlElement(name = "line3")
public String getLine3() {
return line3;
}
/**
* Sets the address in line 3 is used to enter the remaining address information if it does not fit in lines 1 and 2.
*
* @param line3 the address in line 3 is used to enter the remaining address information if it does not fit in lines 1 and 2.
*/
public ShippingAddress line3(String line3) {
this.line3 = line3;
return this;
}
/**
* Gets the address in line 4 is used to enter the remaining address information if it does not fit in lines 1, 2 and 3.
*
* @return the address in line 4 is used to enter the remaining address information if it does not fit in lines 1, 2 and 3.
**/
@XmlElement(name = "line4")
public String getLine4() {
return line4;
}
/**
* Sets the address in line 4 is used to enter the remaining address information if it does not fit in lines 1, 2 and 3.
*
* @param line4 the address in line 4 is used to enter the remaining address information if it does not fit in lines 1, 2 and 3.
*/
public ShippingAddress line4(String line4) {
this.line4 = line4;
return this;
}
/**
* Gets the address in line 5 is used to enter the remaining address information if it does not fit in line 1,2,3 and 4.
*
* @return the address in line 5 is used to enter the remaining address information if it does not fit in line 1,2,3 and 4.
**/
@XmlElement(name = "line5")
public String getLine5() {
return line5;
}
/**
* Sets the address in line 5 is used to enter the remaining address information if it does not fit in line 1,2,3 and 4.
*
* @param line5 the address in line 5 is used to enter the remaining address information if it does not fit in line 1,2,3 and 4.
*/
public ShippingAddress line5(String line5) {
this.line5 = line5;
return this;
}
/**
* Gets the postal code or zip code appended to the mailing address for the purpose of sorting mail.
*
* @return the postal code or zip code appended to the mailing address for the purpose of sorting mail.
**/
@XmlElement(name = "postalCode")
public String getPostalCode() {
return postalCode;
}
/**
* Sets the postal code or zip code appended to the mailing address for the purpose of sorting mail.
*
* @param postalCode the postal code or zip code appended to the mailing address for the purpose of sorting mail.
*/
public ShippingAddress postalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
/**
* Returns true if the arguments are equal to each other and false
* otherwise. Consequently, if both arguments are null, true is returned and
* if exactly one argument is null, false is returned. Otherwise, equality
* is determined by using the equals method of the first argument.
*/
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ShippingAddress shippingAddress = (ShippingAddress) o;
return Objects.equals(recipientInfo, shippingAddress.recipientInfo) &&
Objects.equals(addressId, shippingAddress.addressId) &&
Objects.equals(_default, shippingAddress._default) &&
Objects.equals(city, shippingAddress.city) &&
Objects.equals(country, shippingAddress.country) &&
Objects.equals(subdivision, shippingAddress.subdivision) &&
Objects.equals(line1, shippingAddress.line1) &&
Objects.equals(line2, shippingAddress.line2) &&
Objects.equals(line3, shippingAddress.line3) &&
Objects.equals(line4, shippingAddress.line4) &&
Objects.equals(line5, shippingAddress.line5) &&
Objects.equals(postalCode, shippingAddress.postalCode);
}
/**
* Generates a hash code for a sequence of input values.
*/
@Override
public int hashCode() {
return Objects.hash(recipientInfo, addressId, _default, city, country, subdivision, line1, line2, line3, line4, line5, postalCode);
}
/**
* Returns the result of calling toString for a non-null argument and "null" for a null argument.
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ShippingAddress {\n");
sb.append(" recipientInfo: ").append(toIndentedString(recipientInfo)).append("\n");
sb.append(" addressId: ").append(toIndentedString(addressId)).append("\n");
sb.append(" _default: ").append(toIndentedString(_default)).append("\n");
sb.append(" city: ").append(toIndentedString(city)).append("\n");
sb.append(" country: ").append(toIndentedString(country)).append("\n");
sb.append(" subdivision: ").append(toIndentedString(subdivision)).append("\n");
sb.append(" line1: ").append(toIndentedString(line1)).append("\n");
sb.append(" line2: ").append(toIndentedString(line2)).append("\n");
sb.append(" line3: ").append(toIndentedString(line3)).append("\n");
sb.append(" line4: ").append(toIndentedString(line4)).append("\n");
sb.append(" line5: ").append(toIndentedString(line5)).append("\n");
sb.append(" postalCode: ").append(toIndentedString(postalCode)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy