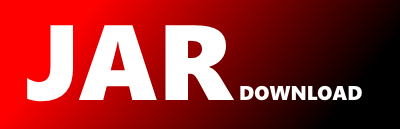
com.mastercard.test.flow.assrt.junit4.Flocessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of assert-junit4 Show documentation
Show all versions of assert-junit4 Show documentation
JUnit4 comparison components
The newest version!
package com.mastercard.test.flow.assrt.junit4;
import java.util.Collection;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import org.junit.Assert;
import org.junit.AssumptionViolatedException;
import org.junit.Rule;
import com.mastercard.test.flow.Flow;
import com.mastercard.test.flow.Model;
import com.mastercard.test.flow.assrt.AbstractFlocessor;
/**
* Integrates {@link Flow} processing into junit 4. This should be used to
* produce the parameters for a parameterised
* test, e.g.:
*
*
* @RunWith(Parameterized.class)
* public class MyTest {
*
* private static final Flocessor flows = new Flocessor( "My test name", MY_SYSTEM_MODEL )
* .system( State.LESS, MY_ACTORS_UNDER_TEST )
* .behaviour( asrt -> {
* // test behaviour
* } );
*
* @Parameters(name = "{0}")
* public static Collection<Object[]> flows() {
* return flows.parameters();
* }
*
* @Parameter(0)
* public String name;
*
* @Parameter(1)
* public Flow flow;
*
* @Rule
* public FlowRule flowRule = flows.rule( () -> flow );
*
* @Test
* public void test() {
* flows.process( flow );
* }
* }
*
*/
public class Flocessor extends AbstractFlocessor {
/**
* @param title A meaningful name for the test
* @param model The system model to exercise
*/
public Flocessor( String title, Model model ) {
super( title, model );
}
/**
* Produces test parameters
*
* @return Test parameters
*/
public Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy