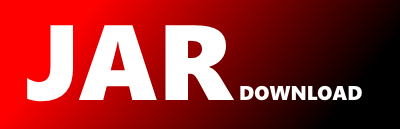
com.mastfrog.giulius.mongodb.async.MongoFutureCollection Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2018 tim.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.mastfrog.giulius.mongodb.async;
import com.google.inject.util.Providers;
import com.mastfrog.util.function.EnhCompletableFuture;
import com.mongodb.Function;
import com.mongodb.ReadConcern;
import com.mongodb.ReadPreference;
import com.mongodb.WriteConcern;
import com.mongodb.async.SingleResultCallback;
import com.mongodb.async.client.MongoCollection;
import com.mongodb.async.client.MongoIterable;
import com.mongodb.bulk.BulkWriteResult;
import com.mongodb.client.model.BulkWriteOptions;
import com.mongodb.client.model.CountOptions;
import com.mongodb.client.model.DeleteOptions;
import com.mongodb.client.model.FindOneAndUpdateOptions;
import com.mongodb.client.model.InsertManyOptions;
import com.mongodb.client.model.InsertOneOptions;
import com.mongodb.client.model.UpdateOptions;
import com.mongodb.client.model.WriteModel;
import com.mongodb.client.result.DeleteResult;
import com.mongodb.client.result.UpdateResult;
import com.mongodb.session.ClientSession;
import java.util.List;
import java.util.concurrent.CopyOnWriteArrayList;
import java.util.function.Consumer;
import javax.inject.Provider;
import org.bson.Document;
import org.bson.conversions.Bson;
import org.bson.types.ObjectId;
/**
* Wraps a MongoCollection's methods that take SingleResultCallback to instead
* return a CompletableFuture.
*
* @author Tim Boudreau
*/
public final class MongoFutureCollection {
private final Provider> coll;
MongoFutureCollection(Provider> coll) {
this.coll = coll;
}
public static MongoFutureCollection forProvider(Provider> prov) {
return new MongoFutureCollection<>(prov);
}
public static MongoFutureCollection forCollection(MongoCollection prov) {
return new MongoFutureCollection<>(Providers.of(prov));
}
public MongoCollection collection() {
return coll.get();
}
public EnhCompletableFuture> aggregate(List extends Bson> list) {
return iterFuture(collection().aggregate(list), null);
}
public EnhCompletableFuture> aggregate(ClientSession cs, List extends Bson> list) {
return iterFuture(collection().aggregate(list), null);
}
public EnhCompletableFuture> aggregate(List extends Bson> list, Class type) {
return iterFuture(collection().aggregate(list, type), null);
}
public EnhCompletableFuture> aggregate(ClientSession sess, List extends Bson> list, Class type) {
return iterFuture(collection().aggregate(list, type), null);
}
public EnhCompletableFuture bulkWrite(List extends WriteModel extends T>> list) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().bulkWrite(list, new SRC<>(result));
return result;
}
public EnhCompletableFuture bulkWrite(List extends WriteModel extends T>> list, BulkWriteOptions bwo) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().bulkWrite(list, bwo, new SRC<>(result));
return result;
}
public EnhCompletableFuture replaceOne(Bson bson, T td) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().replaceOne(bson, td, new SRC<>(result));
return result;
}
public EnhCompletableFuture replaceOne(Bson bson, T td, UpdateOptions uo) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().replaceOne(bson, td, uo, new SRC<>(result));
return result;
}
public EnhCompletableFuture> listIndexes() {
return iterFuture(collection().listIndexes(), null);
}
public EnhCompletableFuture count() {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().count(new SRC<>(result));
return result;
}
public EnhCompletableFuture count(Bson bson) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().count(bson, new SRC<>(result));
return result;
}
public EnhCompletableFuture count(Bson bson, CountOptions co) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().count(bson, co, new SRC<>(result));
return result;
}
public EnhCompletableFuture insertOne(T td) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().insertOne(td, new SRC<>(result));
return result;
}
public EnhCompletableFuture insertOne(T td, InsertOneOptions ioo) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().insertOne(td, ioo, new SRC<>(result));
return result;
}
public EnhCompletableFuture insertMany(List extends T> list) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().insertMany(list, new SRC<>(result));
return result;
}
public EnhCompletableFuture insertMany(List extends T> list, InsertManyOptions imo) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().insertMany(list, imo, new SRC<>(result));
return result;
}
public EnhCompletableFuture deleteOne(Bson bson) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().deleteOne(bson, new SRC<>(result));
return result;
}
public EnhCompletableFuture deleteOne(Bson bson, DeleteOptions d) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().deleteOne(bson, d, new SRC<>(result));
return result;
}
public EnhCompletableFuture deleteOne(ClientSession cs, Bson bson) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().deleteOne(bson, new SRC<>(result));
return result;
}
public EnhCompletableFuture deleteOne(ClientSession cs, Bson bson, DeleteOptions d) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().deleteOne(bson, d, new SRC<>(result));
return result;
}
public EnhCompletableFuture deleteMany(Bson bson) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().deleteMany(bson, new SRC<>(result));
return result;
}
public EnhCompletableFuture deleteMany(Bson bson, DeleteOptions d) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().deleteMany(bson, d, new SRC<>(result));
return result;
}
public EnhCompletableFuture deleteMany(ClientSession cs, Bson bson) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().deleteMany(bson, new SRC<>(result));
return result;
}
public EnhCompletableFuture deleteMany(ClientSession cs, Bson bson, DeleteOptions d) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().deleteMany(bson, d, new SRC<>(result));
return result;
}
public EnhCompletableFuture updateOne(Bson query, Bson update) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().updateOne(query, update, new SRC<>(result));
return result;
}
public EnhCompletableFuture updateOne(Bson query, Bson update, UpdateOptions uo) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().updateOne(query, update, uo, new SRC<>(result));
return result;
}
public EnhCompletableFuture updateMany(Bson query, Bson update) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().updateMany(query, update, new SRC<>(result));
return result;
}
public EnhCompletableFuture updateMany(Bson bson, Bson bson1, UpdateOptions uo) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().updateMany(bson, bson1, uo, new SRC<>(result));
return result;
}
static class SRC implements SingleResultCallback {
private final EnhCompletableFuture fut;
public SRC(EnhCompletableFuture fut) {
this.fut = fut;
}
@Override
public void onResult(T t, Throwable thrwbl) {
if (thrwbl != null) {
fut.completeExceptionally(thrwbl);
} else {
if (fut.isCancelled()) {
return;
}
fut.complete(t);
}
}
}
public EnhCompletableFuture> find() {
return iterFuture(collection().find(), null);
}
private > EnhCompletableFuture> iterFuture(R ft, Consumer cons) {
EnhCompletableFuture> result = new EnhCompletableFuture<>();
List l = new CopyOnWriteArrayList<>();
if (cons != null) {
cons.accept(ft);
}
ft.forEach(t -> {
if (result.isCancelled()) {
return;
}
l.add(t);
}, (v, thrown) -> {
if (thrown != null) {
result.completeExceptionally(thrown);
} else {
if (result.isCancelled()) {
return;
}
result.complete(l);
}
});
return result;
}
public EnhCompletableFuture findOneAndUpdate(Bson query, Bson update, FindOneAndUpdateOptions foauo) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().findOneAndUpdate(query, update, foauo, new SRC<>(result));
return result;
}
public EnhCompletableFuture findOneAndUpdate(Bson query, Bson update) {
return findOneAndUpdate(query, update, new FindOneAndUpdateOptions());
}
public EnhCompletableFuture findOneAndUpdate(ObjectId id, Bson update) {
return findOneAndUpdate(new Document("_id", id), update, new FindOneAndUpdateOptions());
}
public EnhCompletableFuture findOne() {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().find().first(new SRC<>(result));
return result;
}
public EnhCompletableFuture findOne(ObjectId id) {
return findOne(new Document("_id", id));
}
public EnhCompletableFuture findOne(Bson query) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().find(query).first(new SRC<>(result));
return result;
}
public EnhCompletableFuture findOne(Bson query, Bson projection) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().find(query).projection(projection).first(new SRC<>(result));
return result;
}
public EnhCompletableFuture findOne(Bson query, Class type) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().find(query, type).first(new SRC<>(result));
return result;
}
public EnhCompletableFuture findOne(Bson query, Bson projection, Class type) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().find(query, type).projection(projection).first(new SRC<>(result));
return result;
}
public EnhCompletableFuture findOne(ClientSession cs, Bson query) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().find(query).first(new SRC<>(result));
return result;
}
public EnhCompletableFuture findOne(ClientSession cs, Bson query, Bson projection) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().find(query).projection(projection).first(new SRC<>(result));
return result;
}
public EnhCompletableFuture findOne(ClientSession cs, Bson query, Class type) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().find(query, type).first(new SRC<>(result));
return result;
}
public EnhCompletableFuture findOne(ClientSession cs, Bson query, Bson projection, Class type) {
EnhCompletableFuture result = new EnhCompletableFuture<>();
collection().find(query, type).projection(projection).first(new SRC<>(result));
return result;
}
public EnhCompletableFuture> find(Class type) {
return iterFuture(collection().find(type), null);
}
public EnhCompletableFuture> find(Bson bson) {
return iterFuture(collection().find(bson), null);
}
public EnhCompletableFuture> find(Bson bson, Bson projection) {
return iterFuture(collection().find(bson).projection(projection), null);
}
public EnhCompletableFuture> find(Bson bson, Class type) {
return iterFuture(collection().find(bson, type), null);
}
public EnhCompletableFuture> find(Bson bson, Bson projection, Class type) {
return iterFuture(collection().find(bson, type).projection(projection), null);
}
public EnhCompletableFuture> find(ClientSession cs) {
return iterFuture(collection().find(), null);
}
public EnhCompletableFuture> find(ClientSession cs, Class type) {
return iterFuture(collection().find(type), null);
}
public EnhCompletableFuture> find(ClientSession cs, Bson bson) {
return iterFuture(collection().find(bson), null);
}
public EnhCompletableFuture> find(ClientSession cs, Bson bson, Bson projection) {
return iterFuture(collection().find(bson).projection(projection), null);
}
public EnhCompletableFuture> find(ClientSession cs, Bson bson, Bson projection, Class type) {
return iterFuture(collection().find(bson, type).projection(projection), null);
}
public EnhCompletableFuture> distinct(String string, Class type) {
return iterFuture(collection().distinct(string, type), null);
}
public EnhCompletableFuture> distinct(String string, Bson bson, Class type) {
return iterFuture(collection().distinct(string, bson, type), null);
}
public EnhCompletableFuture> distinct(ClientSession cs, String string, Class type) {
return iterFuture(collection().distinct(string, type), null);
}
public EnhCompletableFuture> distinct(ClientSession cs, String string, Bson bson, Class type) {
return iterFuture(collection().distinct(string, bson, type), null);
}
public MongoFutureCollection withDocumentClass(Class type) {
return new MongoFutureCollection<>(xform(cl -> cl.withDocumentClass(type)));
}
public MongoFutureCollection withReadPreference(ReadPreference rp) {
return new MongoFutureCollection<>(xform(cl -> cl.withReadPreference(rp)));
}
public MongoFutureCollection withWriteConcern(WriteConcern wc) {
return new MongoFutureCollection<>(xform(cl -> cl.withWriteConcern(wc)));
}
public MongoFutureCollection withReadConcern(ReadConcern rc) {
return new MongoFutureCollection<>(xform(cl -> cl.withReadConcern(rc)));
}
private Provider> xform(Function, MongoCollection> xform) {
return new TransformProvider<>(coll, xform);
}
private static final class TransformProvider implements Provider> {
private final Provider extends MongoCollection> orig;
private final Function, MongoCollection> xform;
public TransformProvider(Provider extends MongoCollection> orig, Function, MongoCollection> xform) {
this.orig = orig;
this.xform = xform;
}
@Override
public MongoCollection get() {
MongoCollection o = orig.get();
return xform.apply(o);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy