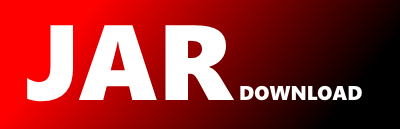
com.mattunderscore.http.headers.EncodingParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of structured-http-headers Show documentation
Show all versions of structured-http-headers Show documentation
Allows HTTP headers to be represented in a structured way. The parsers for each header field are represented as separate classes implementing the 'com.mattunderscore.http.headers.HeaderParser' interface. The values of each header field are represented as separate classes implementing the 'com.mattunderscore.http.headers.HeaderFieldElement' interface. Where a header field has a list of values each of these values is represented as a separate object of the same class. When these values are qualified the class implements the 'com.mattunderscore.http.headers.Qualified' interface.
/* Copyright © 2013 Matthew Champion */
/* */
package com.mattunderscore.http.headers;
import java.util.ArrayList;
import java.util.Collection;
/**
* Parse an accept encoding field header string to a structured representation.
*
* A special note is required for the identity encoding. The identity encoding is always acceptable
* unless it explicitly or by wild card has a qualifier of 0. Where the identity is acceptable it
* shall have the quality value a tenth less than the smallest other quality value. The quality
* value is not part of the specification.
*
* @author Matt Champion
* @since 0.1.2
*/
public class EncodingParser implements HeaderParser
{
public EncodingParser()
{
}
@Override
public boolean isCorrectHeader(String header)
{
return "Accept-Encoding".equalsIgnoreCase(header);
}
@Override
public Collection parseAll(String header) throws UnParsableHeaderException
{
Collection qEcodings = new ArrayList();
String[] types = header.split(",");
boolean addIdentity = true;
double smallestQualifier = 10.0;
for (int i = 0; i < types.length; i++)
{
QEncoding encoding = parse(types[i].trim());
if (encoding != null)
{
if (encoding.sameEncoding(new QEncoding("identity", 1.0)))
{
addIdentity = false;
}
else if (encoding.isAnyEncoding())
{
addIdentity = false;
}
else if (addIdentity && encoding.getQualifier() < smallestQualifier)
{
smallestQualifier = encoding.getQualifier();
}
qEcodings.add(encoding);
}
}
if (addIdentity)
{
qEcodings.add(new QEncoding("identity", smallestQualifier / 10.0));
}
return qEcodings;
}
@Override
public QEncoding parse(String header) throws UnParsableHeaderException
{
if (header == null)
{
throw new UnParsableHeaderException("Null Pointer.");
}
else if ("".equals(header.trim()))
{
return null;
}
else
{
String[] headers = header.split(";q=");
if (headers.length == 2)
{
double qualifier = Double.parseDouble(headers[1].trim());
if (qualifier < 0.0 || qualifier > 1.0)
{
throw new UnParsableHeaderException("Invalid qualifier range.");
}
else
{
return new QEncoding(headers[0].trim(), qualifier);
}
}
else
{
return new QEncoding(headers[0].trim(), 1.0);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy