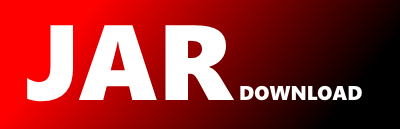
com.maxiaofa.captcha.spring.boot.service.impl.CaptchaServiceImpl Maven / Gradle / Ivy
package com.maxiaofa.captcha.spring.boot.service.impl;
import com.maxiaofa.captcha.Producer;
import com.maxiaofa.captcha.spring.boot.framework.cache.ICacheService;
import com.maxiaofa.captcha.spring.boot.framework.constants.Constants;
import com.maxiaofa.captcha.spring.boot.framework.domain.Captcha;
import com.maxiaofa.captcha.spring.boot.framework.exception.chapcha.CaptchaErrorException;
import com.maxiaofa.captcha.spring.boot.framework.exception.chapcha.CaptchaExpireException;
import com.maxiaofa.captcha.spring.boot.framework.exception.io.CaptchaIoErrorException;
import com.maxiaofa.captcha.spring.boot.framework.utils.RsaUtils;
import com.maxiaofa.captcha.spring.boot.properties.RotateCaptchaProperties;
import com.maxiaofa.captcha.spring.boot.service.ICaptchaService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.util.FastByteArrayOutputStream;
import javax.annotation.Resource;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.IOException;
import java.util.Base64;
import java.util.UUID;
import java.util.concurrent.TimeUnit;
/**
* @author MaXiaoFa
*/
@Service
public class CaptchaServiceImpl implements ICaptchaService {
@Autowired
private ICacheService cacheService;
@Resource(name = "rotateCaptchaProducer")
private Producer rotateCaptchaConfig;
@Autowired
private RotateCaptchaProperties rotateCaptchaProperties;
@Autowired
private RotateCaptchaProperties.Rsa rsa;
/**
* 生成验证码
* @return {@link Captcha}验证码
* */
@Override
public Captcha createCaptcha() throws CaptchaIoErrorException {
String uuid = UUID.randomUUID().toString();
String verifyKey = Constants.CAPTCHA_KEY + uuid;
int angle = rotateCaptchaConfig.createAngle();
FastByteArrayOutputStream os = null;
try {
BufferedImage image = rotateCaptchaConfig.createImage(angle);
cacheService.setCache(verifyKey, String.valueOf(angle),rotateCaptchaProperties.getExpireTime(), TimeUnit.MINUTES);
os = new FastByteArrayOutputStream();
ImageIO.write(image, Constants.IMAGES_SUFFIX, os);
}catch (IOException e){
throw new CaptchaIoErrorException();
}
Captcha captcha = new Captcha();
captcha.setUuid(uuid);
captcha.setImg(Constants.BASE64_HEAD + Base64.getEncoder().encodeToString(os.toByteArray()));
return captcha;
}
/**
* 验证验证码 调用后会删除这个UUID验证码需重新生成
* @param uuid 生成验证码返回的UUID
* @param code 前台传递的验证码
* @return 验证码验证通过生成的key
* */
@Override
public String verifyCaptcha(String uuid,String code) throws CaptchaExpireException,CaptchaErrorException {
String verifyUuid = Constants.CAPTCHA_KEY + uuid;
String verifyValue = cacheService.getCache(verifyUuid);
delCaptcha(verifyUuid);
if(verifyValue==null){
throw new CaptchaExpireException();
}
String angleDecrypt = rsa.isEnable() ? RsaUtils.decrypt(code) : code;
int angle = Integer.parseInt(verifyValue), inputAngle = Integer.parseInt(angleDecrypt);
if(rotateCaptchaConfig.verify(angle,Math.abs(inputAngle-360))){
String key = UUID.randomUUID().toString();
cacheService.setCache(verifyUuid, key,rotateCaptchaProperties.getExpireTime(), TimeUnit.MINUTES);
return key;
}else{
throw new CaptchaErrorException();
}
}
/**
* 验证验证码key 调用后会删除这个UUID验证码需重新生成
* @param uuid 生成验证码返回的UUID
* @param key 验证验证码通过返回的key
* @return 是否验证通过
* */
@Override
public boolean verifyCaptchaKey(String uuid, String key) throws CaptchaExpireException {
String verifyUuid = Constants.CAPTCHA_KEY + uuid;
String verifyKey = cacheService.getCache(verifyUuid);
delCaptcha(verifyUuid);
if(verifyKey==null){
throw new CaptchaExpireException();
}
return verifyKey.equals(key);
}
/**
* 删除验证码
* @param uuid 生成验证码返回的UUID
* */
@Override
public void delCaptcha(String uuid) {
cacheService.delCache(Constants.CAPTCHA_KEY + uuid);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy