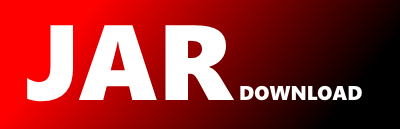
learn.week3.LineSegment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of algorithm Show documentation
Show all versions of algorithm Show documentation
Simple implementation of basic algorithm.
The newest version!
package com.mazhangjing.algorithm.learn.week3;
public class LineSegment {
final Point p; // one endpoint of this line segment
final Point q; // the other endpoint of this line segment
/**
* Initializes a new line segment.
*
* @param p one endpoint
* @param q the other endpoint
* @throws NullPointerException if either p or q
* is null
*/
public LineSegment(Point p, Point q) {
if (p == null || q == null) {
throw new NullPointerException("argument is null");
}
this.p = p;
this.q = q;
}
/**
* Draws this line segment to standard draw.
*/
public void draw() {
p.drawTo(q);
}
/**
* Returns a string representation of this line segment
* This method is provide for debugging;
* your program should not rely on the format of the string representation.
*
* @return a string representation of this line segment
*/
public String toString() {
return p + " -> " + q;
}
/**
* Throws an exception if called. The hashCode() method is not supported because
* hashing has not yet been introduced in this course. Moreover, hashing does not
* typically lead to good *worst-case* performance guarantees, as required on this
* assignment.
*
* @throws UnsupportedOperationException if called
*/
public int hashCode() {
throw new UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy