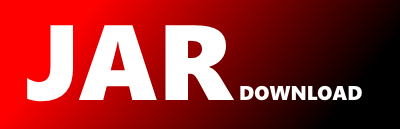
src.samples.java.ex.FII_Sample Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fb-contrib Show documentation
Show all versions of fb-contrib Show documentation
An auxiliary findbugs.sourceforge.net plugin for java bug detectors that fall outside the narrow scope of detectors to be packaged with the product itself.
package ex;
import java.math.BigDecimal;
import java.util.BitSet;
import java.util.EnumSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
public class FII_Sample {
public List getFreeBees(List baubles) {
return baubles.stream().filter(b -> b.isFree()).collect(Collectors.toList());
}
public List getNames(List baubles) {
return baubles.stream().map(b -> b.getName()).collect(Collectors.toList());
}
public void addBitSet(BitSet bs, List ints) {
ints.forEach(i -> bs.set(i));
}
public List getfpFreeBees(List baubles) {
return baubles.stream().filter(Bauble::isFree).collect(Collectors.toList());
}
public List fpGetNames(List baubles) {
return baubles.stream().map(Bauble::getName).collect(Collectors.toList());
}
public Map fpBuildMapper(List l) {
return l.stream().collect(Collectors.toMap(Object::toString, e -> e));
}
public boolean containsOnACollect(List baubles, String name) {
return baubles.stream().map(Bauble::getName).collect(Collectors.toSet()).contains(name);
}
public boolean poorMansAnyMatch(List baubles, String name) {
return baubles.stream().map(Bauble::getName).filter(n -> n.equals(name)).findFirst().isPresent();
}
public Bauble get0OnCollect(List baubles) {
return baubles.stream().collect(Collectors.toList()).get(0);
}
public List backToBackFilter(Set baubles) {
return baubles.stream().filter(b -> b.getName().equals("diamonds")).filter(b -> b.isFree()).collect(Collectors.toList());
}
public Map mapIdentity(List baubles) {
return baubles.stream().collect(Collectors.toMap(Bauble::getName, b -> b));
}
public int sizeOnACollect(List baubles, String name) {
return baubles.stream().filter(b -> b.getName().equals(name)).collect(Collectors.toSet()).size();
}
public void fpUnrelatedLambdaValue282(Map map, BaubleFactory factory) {
map.computeIfAbsent("pixie dust", _unused -> factory.getBauble());
}
public BigDecimal fpCastEliminatesMethodReference282(List baubles) {
return baubles.stream().filter(b -> b.getName().equals("special")).map(b -> (BigDecimal) b.getCost()).findFirst().get();
}
public static Stream fpIiteratorToFiniteStream283(Iterator iterator, boolean parallel) {
Iterable iterable = () -> iterator;
return StreamSupport.stream(iterable.spliterator(), parallel);
}
public void fpUseIdentity283() {
put(m -> {
m.putAll(m);
return m;
});
}
public static void foo(Consumer consumer) {
}
public static void bar342(Runnable runnable) {
foo(_unused -> runnable.run());
}
public void put(Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy