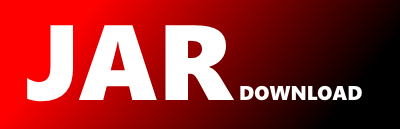
com.mediamiser.test.LogTester Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avogadro Show documentation
Show all versions of avogadro Show documentation
A set of utilities to aid in difficult unit-testing.
// Copyright (c) 2013-2014 MediaMiser Ltd. All rights reserved.
package com.mediamiser.test;
import static org.mockito.Mockito.mock;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* A utility for testing that logging events have occurred and when combined
* with well placed trace logging allows testing control flow.
*
*
*
* When used, a class is instrumented so that actual logging statements will not
* be submitted to the logger, but will instead be intercepted to test their
* contents. This allows us to test conditions that would log errors, but not
* log those errors, meaning that if a test does actually log at the error level
* to an actual logger then an error that attention should be paid to has
* occurred.
*
*
* Not thread safe (so at least restrict your test running to run each set of
* tests on a single object in one thread). Assumes that each class that is
* instrumented has an slf4j logger, e.g.:
*
*
*
* public class MyClass {
* private static final Logger LOG = LoggerFactory.getLogger(MyClass.class);
*
* public static final MESSAGE = "Oops";
*
* public void causeAnError() {
* LOG.error(MESSAGE);
* }
* }
*
*
* This can then be instrumented and the logging statement captured, hidden, but
* verified that it was sent, using:
*
*
*
* public class MyClassTest {
*
* @Test
* public void synopsis() throws Exception {
*
* // Must prepare before using. Only needs to be done once.
* LogTester.prepare(MyClass.class);
*
* // Perform some action that will output to the logger
* final MyClass myClass = new MyClass();
* myClass.causeAnError();
*
* // Verify that the logger was actually called
* verify(LogTester.getMock()).error(MyClass.MESSAGE);
*
* // LogTester will work until close is called.
* LogTester.close();
* }
* }
*
*
* @author Samer Al-Buhaisi
* @author Chris Fournier
*/
public final class LogTester {
public static final String LOGGER_FIELD_NAME = "LOG";
private static Class> klass;
private static Logger mock;
/**
* Obtains the mock that was created from a previous
* {@link LogTester#prepare(Class)} or
* {@link LogTester#prepare(Class, String)} call.
*
* @return A mocked {@link org.slf4j.Logger} instance.
*/
public static Logger getMock() {
return mock;
}
/**
* Replaces with a class' {@link org.slf4j.Logger} with a mock to allow for
* logging statements to be verified and to capture and hide log messages in
* certain situations during testing.
*
* @param klass
* Class with a static {@link org.slf4j.Logger} instance to
* replace with a mock.
* @param loggerFieldName
* Name of the field that stores the static
* {@link org.slf4j.Logger} instance in {@code klass}.
* @throws Exception
* If the static field cannot be manipulated.
*/
public static void prepare(final Class> klass, final String loggerFieldName) throws Exception {
mock = mock(Logger.class);
LogTester.klass = klass;
Manipulator.set(klass, loggerFieldName, mock);
}
/**
* Identical to {@link LogTester#prepare(Class, String)} but assumes that
* the {@code loggerFieldName} is {@link LogTester#LOGGER_FIELD_NAME}.
*
* @see LogTester#prepare(Class, String)
*/
public static void prepare(final Class> klass) throws Exception {
prepare(klass, LOGGER_FIELD_NAME);
}
/**
* Restores a class' {@link org.slf4j.Logger} that was mocked by a previous
* {@link LogTester#prepare(Class)} or
* {@link LogTester#prepare(Class, String)} call.
*/
public static void close() throws Exception {
Manipulator.set(klass, LOGGER_FIELD_NAME, LoggerFactory.getLogger(klass));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy