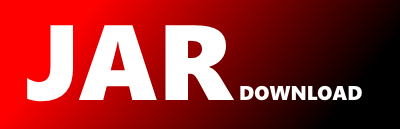
com.dianping.cat.alarm.AlertDao Maven / Gradle / Ivy
The newest version!
package com.dianping.cat.alarm;
import java.util.List;
import org.unidal.dal.jdbc.DalException;
import org.unidal.dal.jdbc.AbstractDao;
import org.unidal.dal.jdbc.Readset;
import org.unidal.dal.jdbc.Updateset;
public class AlertDao extends AbstractDao {
public Alert createLocal() {
Alert proto = new Alert();
return proto;
}
public int deleteByPK(Alert proto) throws DalException {
return getQueryEngine().deleteSingle(
AlertEntity.DELETE_BY_PK,
proto);
}
public List queryAlertsByTimeDomain(java.util.Date startTime, java.util.Date endTime, String domain, Readset readset) throws DalException {
Alert proto = new Alert();
proto.setStartTime(startTime);
proto.setEndTime(endTime);
proto.setDomain(domain);
List result = getQueryEngine().queryMultiple(
AlertEntity.QUERY_ALERTS_BY_TIME_DOMAIN,
proto,
readset);
return result;
}
public List queryAlertsByTimeDomainCategories(java.util.Date startTime, java.util.Date endTime, String domain, String[] categories, Readset readset) throws DalException {
Alert proto = new Alert();
proto.setStartTime(startTime);
proto.setEndTime(endTime);
proto.setDomain(domain);
proto.setCategories(categories);
List result = getQueryEngine().queryMultiple(
AlertEntity.QUERY_ALERTS_BY_TIME_DOMAIN_CATEGORIES,
proto,
readset);
return result;
}
public List queryAlertsByTimeCategoryDomain(java.util.Date startTime, java.util.Date endTime, String category, String domain, Readset readset) throws DalException {
Alert proto = new Alert();
proto.setStartTime(startTime);
proto.setEndTime(endTime);
proto.setCategory(category);
proto.setDomain(domain);
List result = getQueryEngine().queryMultiple(
AlertEntity.QUERY_ALERTS_BY_TIME_CATEGORY_DOMAIN,
proto,
readset);
return result;
}
public List queryAlertsByTimeCategory(java.util.Date startTime, java.util.Date endTime, String category, Readset readset) throws DalException {
Alert proto = new Alert();
proto.setStartTime(startTime);
proto.setEndTime(endTime);
proto.setCategory(category);
List result = getQueryEngine().queryMultiple(
AlertEntity.QUERY_ALERTS_BY_TIME_CATEGORY,
proto,
readset);
return result;
}
public Alert findByPK(int keyId, Readset readset) throws DalException {
Alert proto = new Alert();
proto.setKeyId(keyId);
Alert result = getQueryEngine().querySingle(
AlertEntity.FIND_BY_PK,
proto,
readset);
return result;
}
@Override
protected Class>[] getEntityClasses() {
return new Class>[] { AlertEntity.class };
}
public int insert(Alert proto) throws DalException {
return getQueryEngine().insertSingle(
AlertEntity.INSERT,
proto);
}
public int updateByPK(Alert proto, Updateset updateset) throws DalException {
return getQueryEngine().updateSingle(
AlertEntity.UPDATE_BY_PK,
proto,
updateset);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy