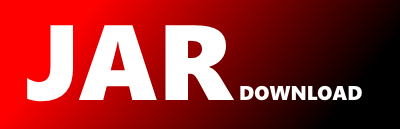
com.meliorbis.economics.aggregate.derivagg.DefaultDASolver Maven / Gradle / Ivy
package com.meliorbis.economics.aggregate.derivagg;
import com.meliorbis.economics.infrastructure.simulation.DiscretisedDistributionSimulator;
import com.meliorbis.economics.model.Model;
import com.meliorbis.economics.model.ModelConfig;
import com.meliorbis.numerics.generic.primitives.DoubleArray;
public class DefaultDASolver, M extends Model>
extends DerivativeAggregationSolver
{
protected DefaultDASolver(M model_, C config_, DiscretisedDistributionSimulator simulator_)
{
super(model_, config_, simulator_);
}
private DoubleArray>[][] _aggByIndDerivatives = null;
private DoubleArray>[][] _aggByAggDerivatives = null;
private DoubleArray>[][] _transByIndDerivatives = null;
private void initAggByIndDerivs()
{
if(_aggByIndDerivatives != null) {
return;
}
_aggByIndDerivatives = new DoubleArray>[_config.getAggregateEndogenousStateCount()][_config.getIndividualEndogenousStateCount()];
}
private void initAggByAggDerivs()
{
if(_aggByAggDerivatives != null) {
return;
}
// Overkill
_aggByAggDerivatives = new DoubleArray>[_config.getAggregateEndogenousStateCount()][_config.getAggregateEndogenousStateCount()];
}
private void initTransByIndDerivs()
{
if(_transByIndDerivatives != null) {
return;
}
// Overkill
_transByIndDerivatives = new DoubleArray>[_config.getAggregateEndogenousStateCount()][_config.getIndividualEndogenousStateCount()];
}
protected void setAggregationByIndividualStateDerivative(int aggregationIndex_, int indIndex_, DoubleArray> values_)
{
initAggByIndDerivs();
_aggByIndDerivatives[aggregationIndex_][indIndex_] = values_;
}
protected void setAggregationByAggregateDerivative(int aggregationIndex_, int otherAggregateIndex_, DoubleArray> values_)
{
initAggByAggDerivs();
_aggByIndDerivatives[aggregationIndex_][otherAggregateIndex_] = values_;
}
protected void setTransformationByIndStateDerivative(int aggIndex_, int indIndex_, DoubleArray> values_)
{
initTransByIndDerivs();
_transByIndDerivatives[aggIndex_][indIndex_] = values_;
}
@Override
protected DoubleArray> deriveAggregationByIndividualState(int aggIndex_, int indIndex_, int[] aggExoIndex_, double[] currentAggregates_,
boolean current_)
{
if(_aggByIndDerivatives == null) return null;
return _aggByIndDerivatives[aggIndex_][indIndex_];
}
@Override
protected DoubleArray> deriveAggregationByAggregateState(int aggretationIndex_, int stateIndex_, int[] aggExoIndex_, double[] currentAggregates_)
{
if(_aggByAggDerivatives == null) return null;
return _aggByAggDerivatives[aggretationIndex_][stateIndex_];
}
@Override
protected DoubleArray> deriveIndividualTransformationByTheta(int transformationIndex_, int individualIndex_, int[] aggExoIndex_,
double[] currentAggregates_)
{
if(_transByIndDerivatives == null) return null;
return _transByIndDerivatives[transformationIndex_][individualIndex_];
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy