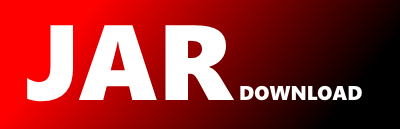
com.meliorbis.economics.individual.egm.EGMIndividualProblemSolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelSolver Show documentation
Show all versions of ModelSolver Show documentation
A library for solving economic models, particularly
macroeconomic models with heterogeneous agents who have model-consistent
expectations
/**
*
*/
package com.meliorbis.economics.individual.egm;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.apache.commons.lang.ArrayUtils;
import com.meliorbis.economics.model.Model;
import com.meliorbis.economics.model.ModelConfig;
import com.meliorbis.economics.model.State;
import com.meliorbis.numerics.generic.primitives.DoubleArray;
import com.meliorbis.numerics.generic.primitives.impl.Interpolation.Params;
import com.meliorbis.utils.Utils;
/**
* Base class for individual problem solvers that use the endogenous gridpoints
* method of Caroll (2006, Economics Letters).
*
* @author Tobias Grasl
*
* @param The Config type
* @param The State type
* @param The Model type
*/
public abstract class EGMIndividualProblemSolver, M extends Model> extends
EGMSolverBase
{
/**
* Constructs a solver for the given model
*
* @param model_ The model being solved
* @param config_ The configuration of the model
*/
public EGMIndividualProblemSolver(M model_, C config_)
{
super(model_, config_);
_interpParams = new Params().constrained(_config.isConstrained());
final DoubleArray> transitionProbabilities = _config.getExogenousStateTransition();
/* Determine the size of the conditional expectations array in advance
*/
List fccDimensions = new ArrayList();
/* IMPORTANT: Assumes the prior ind exo state is not relevant, i.e both markov property
* and that all the endo states are the same
*/
fccDimensions.addAll(Arrays.asList(ArrayUtils.toObject(
ArrayUtils.subarray(transitionProbabilities.size(), _config.getIndividualExogenousStateCount(),
transitionProbabilities.numberOfDimensions()))));
_firstAggStateDim = fccDimensions.size();
Utils.addLengthsToList(fccDimensions, _config.getAggregateEndogenousStates());
_firstIndividualStateDim = fccDimensions.size();
Utils.addLengthsToList(fccDimensions, _config.getIndividualEndogenousStates());
fccDimensions.add(getNumberOfIndividualExpectations());
_futureConditionalContribsSize = Utils.toIntArray(fccDimensions);
final int nIndShocks = _config.getIndividualExogenousStateCount();
final int nIndStates = _config.getIndividualEndogenousStateCount();
final int nAggShocks = _config.getAggregateExogenousStateCount();
final int nAggStates = _config.getAggregateEndogenousStateCount();
final int nAggControls = _config.getAggregateControlCount();
_arrangeIndexs = Utils.combine(
// First the individual endo states, which are at the end of the fcc
// array. NOTE: Just using efc as a convenience since we are extracting
// from
// the (equal length) subindex
Utils.sequence( nAggShocks + nAggStates, nAggShocks + nAggStates + nIndStates),
// Then the aggregate states and controls, which are in the
// subindex where the future shocks are in the full index
Utils.sequence(nAggShocks, nAggShocks+nAggStates),
// Then the agg exo states
Utils.sequence(0, nAggShocks),
// Leave the one at the end which differentiates the variables,
new int[] {nAggShocks+nIndStates+nAggStates,
});
// If there are aggregate controls...
if( nAggControls > 0 )
{
//...need to fill across the control dimensions which are present in the output but not the input
// Ind shocks are missing due to fillAt, and the controls are the dimensions we need to expand to
_acrossDims = Utils.combine(Utils.sequence(0, nIndStates + nAggStates),
Utils.sequence(nIndStates + nAggStates + nAggControls,_futureConditionalContribsSize.length-nIndShocks));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy