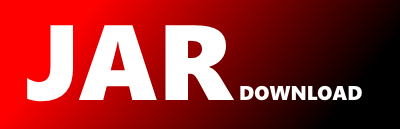
com.meliorbis.economics.infrastructure.Base Maven / Gradle / Ivy
Show all versions of ModelSolver Show documentation
/**
*
*/
package com.meliorbis.economics.infrastructure;
import java.io.File;
import java.io.IOException;
import java.util.logging.Logger;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import com.meliorbis.numerics.Numerics;
import com.meliorbis.numerics.NumericsException;
import com.meliorbis.numerics.function.primitives.DoubleGridFunctionFactory;
import com.meliorbis.numerics.generic.primitives.DoubleArray;
import com.meliorbis.numerics.io.NOOPWriterFactory;
import com.meliorbis.numerics.io.NumericsReader;
import com.meliorbis.numerics.io.NumericsWriter;
import com.meliorbis.numerics.io.NumericsWriterFactory;
import com.meliorbis.numerics.io.csv.CSVReader;
import com.meliorbis.numerics.io.matlab.MatlabReader;
/**
* A base class that combines commonly requires parts of infrastructure classes
*
* @author Tobias Grasl
*/
public class Base
{
private static final Logger LOG = Logger.getLogger(Base.class.getName());
private NumericsWriterFactory _writerFactory = new NOOPWriterFactory();
final protected DoubleGridFunctionFactory _functionFactory;
public Base()
{
_functionFactory = new DoubleGridFunctionFactory();
}
public Base(NumericsWriterFactory writerFactory_)
{
this();
_writerFactory = writerFactory_;
}
protected NumericsWriter getNumericsWriter(File solutionDir_)
{
return getWriterFactory().create(solutionDir_);
}
protected Numerics> getNumerics()
{
return Numerics.instance();
}
/**
* Gets a reader to read data from the provided path.
*
* If the path is a file, is is assumed to be a matlab file, and a corresponding reader is passed.
*
* If the path is a directory, the method looks for a 'state.mat' file. If that exists, as matlab reader is returned
* on that file. Otherwise, a CSV reader on the directory is returned.
*
* @param solutionDir_ Path to a directory or matlab file that must be non-null and exist.
*
* @return A numerics reader as detailed above. Never null.
*/
public NumericsReader getNumericsReader(File solutionDir_)
{
assert solutionDir_ != null : "Null file passed";
assert solutionDir_.exists() : "Non-existent file: "+solutionDir_.getAbsolutePath();
if(solutionDir_.isDirectory())
{
final File matFile = new File(solutionDir_, "state.mat");
// Is there a state.mat file in there?
if(matFile.exists())
{
// Yes
return new MatlabReader(matFile);
}
// No - assume it is a CSV reader
return new CSVReader(solutionDir_);
}
// By elimination, it is an existing file; only available option is mat, check that it has the right extension
if(!solutionDir_.getName().endsWith(".mat"))
{
LOG.warning("Reading state from a file, assumed to be MATLAB, but it does not end in .mat");
}
return new MatlabReader(solutionDir_);
}
public NumericsWriterFactory getWriterFactory()
{
return _writerFactory;
}
public void setWriterFactory(NumericsWriterFactory writerFactory_)
{
_writerFactory = writerFactory_;
}
/**
* Writes an array to a file with the name 'name_<ext>' where ext is
* the value returned by {@code getDefaultExtension} of the configured writer factory.
*
* If {@code name_} already contains an extension it will be stripped. To be precise, anything
* after the last {@code .} is stripped.
*
* @param array_ The array to be written
* @param name_ The name to be given to the file and the array in the file (if applicable).
*/
protected void debugWriteArray(DoubleArray> array_, String name_)
{
try
{
final NumericsWriterFactory writerFactory = getWriterFactory();
Pattern pattern = Pattern.compile(String.format("(.*)[.](?:[^.]*)$"));
final Matcher matcher = pattern.matcher(name_);
// If it matches the extension pattern, return only the bit before the extension
if(matcher.matches())
{
name_ = matcher.group(1);
}
NumericsWriter writer =
writerFactory.create(String.format("%s%s", name_, writerFactory.defaultExtension()));
writer.writeArray(name_, array_);
writer.close();
} catch (IOException e)
{
throw new NumericsException(
String.format("Unable to write array named '%s'",name_),e);
}
}
}