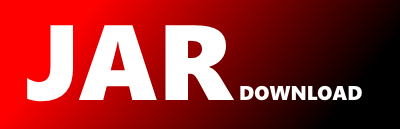
com.meliorbis.economics.infrastructure.simulation.DiscretisedDistribution Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelSolver Show documentation
Show all versions of ModelSolver Show documentation
A library for solving economic models, particularly
macroeconomic models with heterogeneous agents who have model-consistent
expectations
package com.meliorbis.economics.infrastructure.simulation;
import static com.meliorbis.numerics.DoubleArrayFactories.createArrayOfSize;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import com.meliorbis.numerics.generic.MultiDimensionalArray;
import com.meliorbis.numerics.generic.primitives.DoubleArray;
import com.meliorbis.numerics.io.NumericsReader;
import com.meliorbis.numerics.io.NumericsWriter;
import com.meliorbis.numerics.io.csv.CSVReader;
import com.meliorbis.numerics.io.matlab.MatlabReader;
/**
* Presents the state of a simulation in a particular period in the form
* of a discretised distribution.
*/
public final class DiscretisedDistribution implements SimState
{
public static final String DENSITY_VAR = "density";
public static final String OVERFLOW_AVGS_VAR = "oa";
public static final String OVERFLOW_PROPS_VAR = "op";
public DoubleArray> _density;
public DoubleArray> _overflowProportions;
public DoubleArray> _overflowAverages;
public Map> _custom = new HashMap>();
/**
* Default constructor does nothing
*/
public DiscretisedDistribution()
{
}
public DiscretisedDistribution(File inDir_) throws IOException
{
this();
read(inDir_);
}
public DiscretisedDistribution(DiscretisedDistribution other_)
{
_density = createArrayOfSize(other_._density.size());
_overflowProportions = createArrayOfSize(other_._overflowProportions.size());
_overflowAverages = createArrayOfSize(other_._overflowAverages.size());
}
/**
* Returns a new, empty state of the same size as the present one
*
* @return The newly created, empty SimState
*/
public DiscretisedDistribution createSameSized()
{
return new DiscretisedDistribution(this);
}
/* (non-Javadoc)
* @see com.meliorbis.economics.infrastructure.simulation.ISimState#clone()
*/
@Override
public DiscretisedDistribution clone()
{
DiscretisedDistribution clone = new DiscretisedDistribution();
clone._density = _density.copy();
clone._overflowAverages = _overflowAverages.copy();
clone._overflowProportions = _overflowProportions.copy();
return clone;
}
/* (non-Javadoc)
* @see com.meliorbis.economics.infrastructure.simulation.ISimState#mean(com.meliorbis.numerics.generic.primitives.IDoubleArray)
*/
@Override
public double mean(DoubleArray> levels_)
{
return _density.mean(levels_, 0) + overflowMeanContribution();
}
private double overflowMeanContribution()
{
return _overflowAverages.matrixMultiply(_overflowProportions.transpose(0, 1)).get(0);
}
/* (non-Javadoc)
* @see com.meliorbis.economics.infrastructure.simulation.ISimState#variance(com.meliorbis.numerics.generic.primitives.IDoubleArray)
*/
@Override
public double variance(DoubleArray> levels_)
{
return variance(levels_, Double.NaN);
}
/* (non-Javadoc)
* @see com.meliorbis.economics.infrastructure.simulation.ISimState#variance(com.meliorbis.numerics.generic.primitives.IDoubleArray, double)
*/
@Override
public double variance(DoubleArray> levels_, double mean_)
{
// Calculate the mean first if need be
if (Double.isNaN(mean_))
{
mean_ = _density.mean(levels_, 0);
mean_ += overflowMeanContribution();
}
return // UNCENTERED!
_density.secondMoment(levels_, 0) +
// Add the overflow sum-of-sqares
_overflowAverages.multiply(_overflowAverages).
matrixMultiply(_overflowProportions.transpose(0, 1)).get(0)
// Adjust the square of the mean part
- Math.pow(mean_, 2);
}
/* (non-Javadoc)
* @see com.meliorbis.economics.infrastructure.simulation.ISimState#write(com.meliorbis.numerics.io.INumericsWriter, java.lang.String)
*/
@Override
public void write(NumericsWriter writer_, String name_) throws IOException
{
final Map> arrays =
new HashMap>();
arrays.put(DENSITY_VAR,_density);
arrays.put(OVERFLOW_AVGS_VAR,_overflowAverages);
arrays.put(OVERFLOW_PROPS_VAR,_overflowProportions);
if(name_ != null)
{
writer_.writeStructure(name_, arrays);
}
else
{
writer_.writeArrays(arrays);
}
}
/* (non-Javadoc)
* @see com.meliorbis.economics.infrastructure.simulation.ISimState#write(com.meliorbis.numerics.io.INumericsWriter)
*/
@Override
public void write(NumericsWriter writer_) throws IOException
{
write(writer_, null);
}
/* (non-Javadoc)
* @see com.meliorbis.economics.infrastructure.simulation.ISimState#read(com.meliorbis.numerics.io.INumericsReader, java.lang.String)
*/
@Override
public void read(NumericsReader reader_, String name_) throws IOException
{
final Map> arrays =
new HashMap>();
arrays.put("density",_density);
arrays.put("oa",_overflowAverages);
arrays.put("op",_overflowProportions);
if(name_ != null && !name_.isEmpty())
{
final Map> struct = reader_.getStruct(name_);
_density = (DoubleArray>) struct.get(DENSITY_VAR);
_overflowAverages = (DoubleArray>) struct.get(OVERFLOW_AVGS_VAR);
_overflowProportions = (DoubleArray>) struct.get(OVERFLOW_PROPS_VAR);
}
else
{
_density = (DoubleArray>) reader_.getArray(DENSITY_VAR);
_overflowAverages = (DoubleArray>) reader_.getArray(OVERFLOW_AVGS_VAR);
_overflowProportions = (DoubleArray>) reader_.getArray(OVERFLOW_PROPS_VAR);
}
}
/* (non-Javadoc)
* @see com.meliorbis.economics.infrastructure.simulation.ISimState#read(java.io.File)
*/
@Override
public void read(File file_) throws IOException
{
if(!file_.exists())
{
throw new FileNotFoundException(file_.getPath());
}
NumericsReader reader;
// If it isn't a directory
if(!file_.isDirectory())
{
reader = new MatlabReader(file_);
}
else
{
reader = new CSVReader(file_);
}
read(reader, null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy