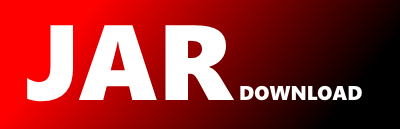
com.meliorbis.economics.infrastructure.simulation.RepresentativeAgentSimState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelSolver Show documentation
Show all versions of ModelSolver Show documentation
A library for solving economic models, particularly
macroeconomic models with heterogeneous agents who have model-consistent
expectations
/**
*
*/
package com.meliorbis.economics.infrastructure.simulation;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.IOException;
import com.meliorbis.numerics.generic.primitives.DoubleArray;
import com.meliorbis.numerics.io.NumericsReader;
import com.meliorbis.numerics.io.NumericsWriter;
import com.meliorbis.numerics.io.csv.CSVReader;
import com.meliorbis.numerics.io.matlab.MatlabReader;
/**
* Simulation State for Representative Agent Models
*
* @author Tobias Grasl
*/
public class RepresentativeAgentSimState implements SimState
{
private static final String DEFAULT_ARRAY_NAME = "states";
private DoubleArray> _states;
/**
* Constructs an empty sim state
*/
public RepresentativeAgentSimState()
{
}
/**
* Constructs the sim state for the current state variables
*
* @param states_ The current state variables
*/
public RepresentativeAgentSimState(DoubleArray> states_)
{
_states = states_;
}
/**
* @return The state variable values
*/
public DoubleArray> getStates()
{
return _states;
}
/**
* Returns the first state variable
*/
@Override
public double mean(DoubleArray> levels_)
{
return _states.get(0);
}
/**
* Returns 0, since the distribution is a single point
*/
@Override
public double variance(DoubleArray> levels_)
{
return 0d;
}
/**
* Returns 0, since the distribution is a single point
*/
@Override
public double variance(DoubleArray> levels_, double mean_)
{
return 0;
}
@Override
public void write(NumericsWriter writer_, String name_) throws IOException
{
writer_.writeArray(name_, _states);
}
@Override
public void write(NumericsWriter writer_) throws IOException
{
write(writer_, DEFAULT_ARRAY_NAME);
}
@Override
public void read(NumericsReader reader_, String name_) throws IOException
{
_states = (DoubleArray>) reader_.getArray(name_);
}
@Override
public void read(File file_) throws IOException
{
if(!file_.exists())
{
throw new FileNotFoundException(file_.getPath());
}
NumericsReader reader;
// If it isn't a directory
if(!file_.isDirectory())
{
reader = new MatlabReader(file_);
}
else
{
reader = new CSVReader(file_);
}
read(reader, DEFAULT_ARRAY_NAME);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy