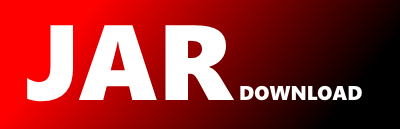
com.meliorbis.economics.infrastructure.simulation.SimulationResults Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelSolver Show documentation
Show all versions of ModelSolver Show documentation
A library for solving economic models, particularly
macroeconomic models with heterogeneous agents who have model-consistent
expectations
package com.meliorbis.economics.infrastructure.simulation;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import com.meliorbis.numerics.generic.MultiDimensionalArray;
import com.meliorbis.numerics.generic.primitives.DoubleArray;
import com.meliorbis.numerics.io.NumericsWriter;
/**
* Holds the aggegate results from a simulation run
*
* @param The Simulation-state type
* @param The numeric type of shocks
*/
public final class SimulationResults
{
private final List> _periods;
private S _finalState;
private DoubleArray> _states;
private DoubleArray> _controls;
public SimulationResults()
{
_periods = new ArrayList<>();
}
public SimulationResults(MultiDimensionalArray shocks_, DoubleArray> states_,
DoubleArray> controls_)
{
this();
for (int i = 0; i < shocks_.size()[0]; i++)
{
addPeriod(shocks_.at(i), states_.at(i),controls_ != null ? controls_.at(i) : null);
}
}
public void addPeriod(MultiDimensionalArray shocks_,
DoubleArray> states_,
DoubleArray> controls_)
{
_periods.add(new PeriodAggregateState(shocks_,states_,controls_));
}
public MultiDimensionalArray getShocks()
{
// Can't create an array here (don't have the factory) so just return null
if(_periods.size() == 0)
{
return null;
}
final MultiDimensionalArray initialShocks = _periods.get(0).getShocks();
if(_periods.size() == 1)
{
// Only one array, so that's all we need to return
return initialShocks.copy();
}
// Need to create an array for all the later stack elements
@SuppressWarnings("unchecked")
MultiDimensionalArray laterShocks[] = new MultiDimensionalArray[_periods.size()-1];
// Copy all the period shocks into the array
for(int i = 1; i < _periods.size();i++)
{
laterShocks[i-1] = _periods.get(i).getShocks();
}
// Return the stacked array of all the shocks
return initialShocks.stack(laterShocks).transpose(0,1).copy();
}
public DoubleArray> getStates()
{
// Can't create an array here (don't have the factory) so just return null
if(_periods.size() == 0)
{
return null;
}
final DoubleArray> intialStates = _periods.get(0).getStates();
if(_states == null) {
// Need to create an array for all the later stack elements
DoubleArray> laterStates[] = new DoubleArray[_periods.size()-1];
// Copy all the period shocks into the array
for(int i = 1; i < _periods.size();i++)
{
laterStates[i-1] = _periods.get(i).getStates();
}
// Return the stacked array of all the shocks
_states = intialStates.stack(laterStates).transpose(0, 1).copy();
}
return _states;
}
public DoubleArray> getControls()
{
// Can't create an array here (don't have the factory) so just return null
if(_periods.size() == 0)
{
return null;
}
final DoubleArray> initialControls = _periods.get(0).getControls();
// The simulated model may not have controls
if(initialControls == null || initialControls.numberOfElements() == 0)
{
return null;
}
if(_controls == null)
{
// Need to create an array for all the later stack elements
DoubleArray> laterControls[] = new DoubleArray[_periods.size()-1];
// Copy all the period shocks into the array
for(int i = 1; i < _periods.size();i++)
{
laterControls[i-1] = _periods.get(i).getControls();
}
// Return the stacked array of all the shocks
_controls = initialControls.stack(laterControls).transpose(0, 1).copy();
}
return _controls;
}
public S getFinalState()
{
return _finalState;
}
public void setFinalState(S finalState_)
{
_finalState = finalState_;
}
public PeriodAggregateState getPeriod(int period_)
{
return _periods.get(period_);
}
public void write(NumericsWriter writer_) throws IOException
{
writer_.writeArray("shocks", (MultiDimensionalArray extends Number, ?>) getShocks());
writer_.writeArray("states", getStates());
if (getControls() != null)
{
writer_.writeArray("controls", getControls());
}
final S finalState = getFinalState();
if (finalState != null)
{
finalState.write(writer_, "finalDistribution");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy