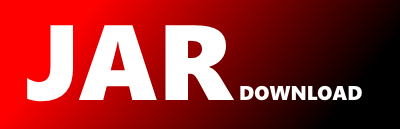
com.meliorbis.economics.infrastructure.simulation.Simulator Maven / Gradle / Ivy
package com.meliorbis.economics.infrastructure.simulation;
import java.io.File;
import com.meliorbis.economics.model.Model;
import com.meliorbis.economics.model.ModelConfig;
import com.meliorbis.economics.model.ModelException;
import com.meliorbis.economics.model.ModelWithControls;
import com.meliorbis.economics.model.State;
import com.meliorbis.economics.model.StateWithControls;
import com.meliorbis.numerics.generic.MultiDimensionalArray;
public interface Simulator
{
/**
* Creates a random sequence of aggregate shocks for simulating a model
* economy, consistent with the shock specification of model_.
*
* @param initialShockStates_
* Initial exogenous state of the economy
* @param periods_
* The number of periods for which to create a shock sequence
* @param model_
* The model for which to create a shock sequence
* @return An array of length periods_, and where each period contains a
* shock value for each aggregate exogenous shock of the model
*/
public MultiDimensionalArray createShockSequence(MultiDimensionalArray initialShockStates_, int periods_, Model, ?> model_);
/**
* Creates a random sequence of aggregate shocks for simulating a model
* economy, consistent with the shock specification of model_. Providing the
* same seed will return the same sequence of shocks, for repeatability.
*
* @param initialShockStates_
* Initial exogenous state of the economy
* @param periods_
* The number of periods for which to create a shock sequence
* @param model_
* The model for which to create a shock sequence
* @param seed_
* The seed for the random number generator
* @return An array of length periods_, and where each period contains a
* shock value for each aggregate exogenous shock of the model
*/
public MultiDimensionalArray createShockSequence(MultiDimensionalArray initialShockStates_, int periods_, Model, ?> model_, T seed_);
/**
* Simulate a provided sequence of shocks using the aggregate transition
* rules
*
* @param shocks_
* The sequence of shocks to simulate
* @param initialState_
* The initial aggregate states
* @param model_
* The model
* @param state_
* The model's state
* @param outDir_
* The directory to which to write simulation results
* @param resultsPath_
* The path within the output directory at which sim results
* should be placed
* @return A SimResults object containing the path of shocks, aggregate
* variables and controls
*
* @throws ModelException If there is a model-specific failure
* @throws SimulatorException If there is a generic Simulator failure
*
* @param The type used to hold state during the calculation
*/
public > SimulationResults simAggregate(
MultiDimensionalArray shocks_,
PeriodAggregateState initialState_,
Model, S> model_,
S state_,
File outDir_,
String resultsPath_) throws ModelException, SimulatorException;
/**
* Simulate a provided sequence of shocks using the aggregate transition
* rules
*
* @param shocks_
* The sequence of shocks to simulate
* @param initialState_
* The initial aggregate states
* @param model_
* The model
* @param state_
* The model's state
* @param outDir_
* The directory to which to write simulation results
* @param resultsPath_
* The path within the output directory at which sim results
* should be placed
* @return A SimResults object containing the path of shocks, aggregate
* variables and controls
*
* @throws ModelException If there is a model-specific failure
* @throws SimulatorException If there is a generic Simulator failure
*
* @param The type used to configure the model
* @param The type used to hold state during the calculation
* @param The type of the model being solved
*/
public , MC extends ModelWithControls> SimulationResults simAggregatesForecastingControls(
MultiDimensionalArray shocks_, PeriodAggregateState initialState_, MC model_, SC state_, File outDir_,
String resultsPath_) throws ModelException, SimulatorException;
public , M extends Model, S>> SimulationResults simulate(int periods_, int burnIn_,
D initialState_, MultiDimensionalArray initialShockStates_, M model_, S calcState_, SimulationObserver observer_, File outputDir_,
String resultsPath_) throws SimulatorException, ModelException;
public , M extends Model, S>> SimulationResults simulate(int periods_, int burnIn_,
D initialState_, MultiDimensionalArray initialShockStates_, M model_, S calcState_, SimulationObserver observer_)
throws SimulatorException, ModelException;
public , M extends Model, S>> SimulationResults simulateShocks(D simState_,
MultiDimensionalArray shocks_, M model_, S calcState_, SimulationObserver observer_, File outputDir_,
String resultsPath_) throws SimulatorException, ModelException;
public abstract , M extends Model, S>> SimulationResults simulateShocks(D simState_,
MultiDimensionalArray shocks_, M model_, S calcState_, SimulationObserver observer_) throws ModelException;
public abstract , M extends Model, S>> TransitionRecord simulateTransition(
D distribution_, M model_, S calcState_, MultiDimensionalArray priorAggShockIndices_, MultiDimensionalArray futureShocks_)
throws ModelException;
public abstract D createState();
public abstract , M extends Model, S>> D findErgodicDist(M model_, S state_, D simState_)
throws ModelException, SimulatorException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy