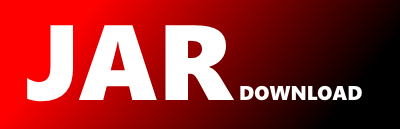
com.meliorbis.economics.model.AllAggsNewtonStrategy Maven / Gradle / Ivy
package com.meliorbis.economics.model;
/**
* Uses a multidimensional Newton method to approach the fixed point
*
* @author Tobias Grasl
*/
public class AllAggsNewtonStrategy //implements IFixedPointStrategy
{
// private static final double MAX_ERR = 1e-5;
// private static final Double GRAD_ADJUST = 1e-6;
//
// private final double _dampingFactor;
//
// private Double[] _lastImplied;
// private Double[] _lastAssumed;
// private int _currentGradIndex = -1;
//
// private RealMatrix _jacobean;
//
// private double _lastDelta;
//
// private IFixedPointValueDelegate _delegate;
// private RealMatrix _lastJump;
//
//
//
// public AllAggsNewtonStrategy()
// {
// this(1.0d);
// }
//
// public AllAggsNewtonStrategy(double dampingFactor_)
// {
// _dampingFactor = dampingFactor_;
// }
//
// private Double[] getImplied(AggregateFixedPointState state_) throws FPStrategyException
// {
// return _delegate.getOutputs(state_);
// }
//
// private Double[] getAssumed(AggregateFixedPointState state_) throws FPStrategyException
// {
// return _delegate.getInputs(state_);
// }
//
// private void addToVariable(int index_, Double delta_, AggregateFixedPointState state_) throws FPStrategyException
// {
// _delegate.adjustInput(index_, delta_, state_);
// }
//
// @Override
// public double calculateCriterionAndAdjust(AggregateFixedPointState state_) throws FPStrategyException
// {
// double criterion = 0d;
//
// Double[] implied = getImplied(state_);
//
// // Are the implied values valid?
// if(!checkValid(implied))
// {
// // No! backtrack
// failedAtPoint(state_);
//
// // Reset to initial starting state at point
// _currentGradIndex = -1;
//
// return 1d;
// }
//
// Double[] assumed = getAssumed(state_);
//
// // Compare each one to the assumed state and update the criterion to be the maximum relative difference
// for(int varIndex = 0; varIndex < assumed.length; varIndex++)
// {
// double currError = Math.abs(implied[varIndex]-assumed[varIndex]);
//
// if(currError > criterion)
// {
// criterion = currError;
// }
// }
//
//
// // Solution found!
// if(criterion < MAX_ERR)
// {
// return criterion;
// }
//
// if(_jacobean == null)
// {
// _jacobean = MatrixUtils.createRealMatrix(implied.length, implied.length);
// }
//
// // Are we in the process of calculating a grad?
// if(_currentGradIndex >= 0)
// {
// double[] column = new double[implied.length];
//
// // Yes! Construct the jacobean column - Since most of the assumed's did not changed
// // don't need to adjust for them here
// for(int i = 0; i < implied.length; i++)
// {
// column[i] = (implied[i] - _lastImplied[i])/_lastDelta;
// }
//
// // But just for the one assumed that did change... (remember it was
// // already divided by the delta above, hence the delta becomes 1!)
// column[_currentGradIndex] -= 1;
//
// // Store the column
// _jacobean.setColumn(_currentGradIndex, column);
//
// // Remove the grad delta from the variable again
// addToVariable(_currentGradIndex, -_lastDelta, state_);
//
// // Move to the next dimension
// _currentGradIndex++;
// }
//
// // Did we just complete the whole grad?
// if(_currentGradIndex == assumed.length)
// {
// // Yes! Invert the jacobean and multiply by the error
// double[] error = new double[_lastImplied.length];
//
// for(int i = 0; i < error.length; i++)
// {
// error[i] = _lastImplied[i] - _lastAssumed[i];
// }
//
// RealMatrix solved = new LUDecomposition(_jacobean).getSolver().solve(
// MatrixUtils.createColumnRealMatrix(error));
//
// _lastJump = solved.scalarMultiply(getDampingFactor());
//
//
// System.out.println("Completed Jacobean, adjusting vars");
//
// // Adjust to the new point
// for(int i = 0; i < assumed.length; i++)
// {
//
// System.out.println(String.format("x%s = %s",i,
// _lastAssumed[i]-_lastJump.getEntry(i, 0)));
//
// addToVariable(i,-_lastJump.getEntry(i, 0),state_);
// }
//
// // Reset for the next round
// _currentGradIndex = -1;
// }
// else if(_currentGradIndex == -1)
// {
// // Store this point, which is where the grad is being calculated
// _lastAssumed = assumed;
// _lastImplied = implied;
//
// System.out.println("Newton iteration complete");
//
// _currentGradIndex++;
// }
//
// // Now we are handling the next grad
// if(_currentGradIndex >= 0)
// {
// // Calculate the delta and adjust the var
// _lastDelta = /*_lastAssumed[_currentGradIndex]**/GRAD_ADJUST;
//
//
// System.out.println(String.format("Setting x%s=%s for derivative calc ",_currentGradIndex,
// _lastAssumed[_currentGradIndex]+_lastDelta));
//
// addToVariable(_currentGradIndex, _lastDelta, state_);
// }
//
// // Return the criterion
// return criterion;
// }
//
// /**
// * @return
// */
// protected double getDampingFactor()
// {
// if(_dampingFactor > 0)
// {
// return _dampingFactor;
// }
// else
// {
// return Math.random()/2d+0.5;
// }
// }
//
// /**
// * Checks that the implied values are finite numbers
// * @param implied_
// * @return
// */
// private boolean checkValid(Double[] implied_)
// {
// for(int i = 0; i < implied_.length; i++)
// {
// Double d = implied_[i];
//
// if(Double.isInfinite(d)||Double.isNaN(d))
// {
// System.out.println(String.format("Invalid Aggregate at index %s: %s",i,d));
// return false;
// }
//
// }
// return true;
// }
//
// public void setDelegate(IFixedPointValueDelegate delegate)
// {
// _delegate = delegate;
// }
//
// public void failedAtPoint(AggregateFixedPointState state_) throws FPStrategyException
// {
// System.out.println("Backtracking due to failure");
//
// // Failed at the last point - backtrack halfway!
// for(int i = 0; i < _lastImplied.length; i++)
// {
// double half = _lastJump.getEntry(i, 0)/2d;
//
// addToVariable(i,half,state_);
//
// // Store the delta as halfway, so the next time it
// // would only go a quarter back etc.
// _lastJump.setEntry(i,0,half);
// }
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy