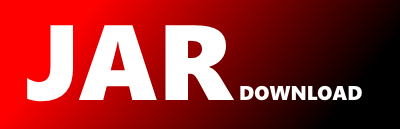
com.meliorbis.economics.model.Model Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ModelSolver Show documentation
Show all versions of ModelSolver Show documentation
A library for solving economic models, particularly
macroeconomic models with heterogeneous agents who have model-consistent
expectations
package com.meliorbis.economics.model;
import java.io.IOException;
import com.meliorbis.economics.aggregate.AggregateProblemSolver;
import com.meliorbis.economics.individual.IndividualProblemSolver;
import com.meliorbis.economics.infrastructure.simulation.SimState;
import com.meliorbis.numerics.fixedpoint.FixedPointValueDelegate;
import com.meliorbis.numerics.generic.MultiDimensionalArray;
import com.meliorbis.numerics.generic.primitives.DoubleArray;
import com.meliorbis.numerics.io.NumericsReader;
import com.meliorbis.numerics.io.NumericsWriter;
/**
* Represents models that can be solved by this toolkit and provides various methods that must be implemented in order to be able to
* do so
*
* @author Tobias Grasl
*
* @param The type used to configure this model
* @param THe type to hold calculation state for this model
*/
public interface Model>
extends ModelEvents
{
public void initialise();
/**
* @return The current configuration applied to this model
*/
public C getConfig();
/**
* Retrieves the delegate to get and update values for the fixed point strategy
*
* @return The fixed-point delegate to use for finding no-agg-risk steady
* states
*/
public FixedPointValueDelegate>> getFixedPointDelegate();
/**
* Given the current state of the calculation this method determines whether the aggregate
* solver should be called
*
* @param state_ The state of the calculation
*
* @return True if the aggregate solver is to be called, false otherwise
*/
public boolean shouldUpdateAggregates(S state_);
/**
* Called at the beginning of an iteration in the steady-state loop. Can be used for instance to
* grab some of the existing state so that it may be used for comparison at the end of the loop
*
* @param state_ The current calc state
*
* @throws ModelException If a model-specific error occurs.
*/
void beginIteration(S state_) throws ModelException;
/**
* @return An appropriate state instance for use with this model
*/
S initialState();
/**
* Calculates and returns the values of endogenous aggregate variables given the simulation state and shock values
* provided
*
* @param simState_ The current simulation state
* @param currentAggShock_ The aggregate exogenous state
* @param calcState_ The state of the model
*
* @return The current aggregate exogenous state values
*
* @throws ModelException If there are issues
*
* @param The numeric type of shocks
*/
double[] calculateAggregateStates(
SimState simState_,
MultiDimensionalArray currentAggShock_,
S calcState_) throws ModelException;
/**
* Gives implementations an opportunity to write data beyond the default when the state is saved
*
* @param state_ The calculation state being written
* @param writer_ The writer to write the state to
*
* @throws IOException If writing fails
*/
void writeAdditional(S state_, NumericsWriter writer_) throws IOException;
/**
* Gives implementations an opportunity to read data beyond the default when the state is loaded
*
* @param state_ The calculation state being read
* @param reader_ The reader to read the state from
*
* @throws IOException If reading fails
*/
void readAdditional(S state_, NumericsReader reader_) throws IOException;
/**
* Creates an array sized correctly for holding aggregate expectations. The final dimension, {@code numVars_} in size,
* is the number of expectations the array will hold.
*
* The dimensions in order are:
*
* - current exogenous state dimensions
*
- future exogenous state dimensions
*
- future normalising exogenous state dimensions
*
- current endogenous state dimensions
*
- current control dimensions
*
- A single dimension of size {@code numVars_} to hold the expected values
*
* @param numVars_ The number of variables expectations are to be held for
*
* @return The appropriately sized array
*/
public DoubleArray> createAggregateExpectationGrid(int numVars_);
/**
* Creates an array sized correctly for holding expectations of individual variables given current aggregates.
*
* The final dimension, {@code numVars_} in size, is the number of expectations the array will hold.
*
* The dimensions in order are:
*
* - current exogenous state dimensions
*
- future exogenous state dimensions
*
- future normalising exogenous state dimensions
*
- current endogenous state dimensions
*
- current control dimensions
*
- future individual endogenous state dimensions
*
- future individual exogenous state dimensions
*
- A single dimension of size {@code getConfig().getIndividualEndogenousStateCount()} to hold the expected values
*
*
* @return The appropriately sized array
*/
public DoubleArray> createIndividualExpectationGrid();
/**
* Creates an array sized correctly for holding expectations of individual variables given current aggregates.
*
* The final dimension, {@code numVars_} in size, is the number of expectations the array will hold.
*
* The dimensions in order are:
*
* - current exogenous state dimensions
*
- future exogenous state dimensions
*
- future normalising exogenous state dimensions
*
- current endogenous state dimensions
*
- current control dimensions
*
- future individual endogenous state dimensions
*
- future individual exogenous state dimensions
*
- A single dimension of size {@code numVars_} to hold the expected values
*
* @param numVars_ The number of variables expectations are to be held for
*
* @return The appropriately sized array
*/
public DoubleArray> createIndividualExpectationGrid(int numVars_);
/**
* Creates an array sized correctly for holding aggregate expectations. The final dimension, holding the number of expectations,
* is the number of aggregate endogenous states.
*
* The dimensions in order are:
*
* - current exogenous state dimensions
*
- future exogenous state dimensions
*
- future normalising exogenous state dimensions
*
- current endogenous state dimensions
*
- current control dimensions
*
- A single dimension of size {@code getConfig().getAggregateEndogenousStateCount()} to hold the expected values
*
*
* @return The appropriately sized array
*/
public DoubleArray> createAggregateExpectationGrid();
/**
* Creates an array sized correctly for holding current-period aggregate variables. The final dimension, {@code numVars_} in size,
* is the number of variables values will be held for
*
* The dimensions in order are:
*
* - current endogenous state dimensions
*
- current control dimensions
*
- current exogenous state dimensions
*
- A single dimension of size {@code numVars_} to hold values
*
*
* @param numVars_ The number of aggregate variables to create a grid for
*
* @return The appropriately sized array
*/
public DoubleArray> createAggregateVariableGrid(int numVars_);
/**
* Creates an array sized correctly for holding a single current-period aggregate variable. The final dimension, indicating the number of
* variables held, is of size one.
*
* The dimensions in order are:
*
* - current endogenous state dimensions
*
- current control dimensions
*
- current exogenous state dimensions
*
- A dimensions of size {@code 1}
*
*
* @return The appropriately sized array
*/
public DoubleArray> createAggregateVariableGrid();
/**
* Creates an array sized correctly for holding one or more current-period individual variables. The final dimension, indicating the number of
* variables held, is of size {@code numVars_}.
*
* The dimensions in order are:
*
* - individual endogenous states
*
- individual exogenous states
*
- aggregate endogenous state dimensions
*
- aggregate control dimensions
*
- aggregate exogenous state dimensions
*
- A dimensions of size {@code numVars_}
*
*
* @param numVars_ The number of individual variables to create a grid for
*
* @return The appropriately sized array
*/
public DoubleArray> createIndividualVariableGrid(int numVars_);
/**
* Creates an array sized correctly for holding a single current-period individual variable. The final dimension, indicating the number of
* variables held, is of size one.
*
* The dimensions in order are:
*
* - individual endogenous states
*
- individual exogenous states
*
- aggregate endogenous state dimensions
*
- aggregate control dimensions
*
- aggregate exogenous state dimensions
*
- A dimensions of size {@code 1}
*
*
* @return The appropriately sized array
*/
public DoubleArray> createIndividualVariableGrid();
/**
* Creates an array sized correctly for holding one or more individual transition variables. The final dimension, indicating the number of
* variables held, is of size {@code numVars_}.
*
* The dimensions in order are:
*
* - individual endogenous states
*
- individual exogenous states
*
- aggregate endogenous state dimensions
*
- aggregate control dimensions
*
- aggregate exogenous state dimensions
*
- aggregate normalising exogenous state dimensions
*
- A dimensions of size {@code numVars_}
*
*
* @param numVars_ The number of individual variables to create a grid for
*
* @return The appropriately sized array
*/
public DoubleArray> createIndividualTransitionGrid(int numVars_);
/**
* Creates an array sized correctly for holding a single individual transition variable. The final dimension, indicating the number of
* variables held, is of size one.
*
* The dimensions in order are:
*
* - individual endogenous states
*
- individual exogenous states
*
- aggregate endogenous state dimensions
*
- aggregate control dimensions
*
- aggregate exogenous state dimensions
*
- aggregate normalising exogenous state dimensions
*
- A dimensions of size {@code 1}
*
*
* @return The appropriately sized array
*/
public DoubleArray> createIndividualTransitionGrid();
/**
* Given an on-grid individual or aggregate variable, interpolates it to the
* expected future values conditional on the realisation of future aggregate
* shocks.
*
* @param individualVar_ The variable to interpolate, which should be on-grid
* @param state_ The current processing state
*
* @return The array of conditional expectations
*/
public DoubleArray> conditionalExpectation(DoubleArray> individualVar_,
final S state_);
/**
* Creates a grid that is the same size as the distribution grid used for simulation
*
* @return A correctly sized grid
*/
public DoubleArray> createSimulationGrid();
/**
* @return The solver to be used to update the aggregate forecasting function
*/
public AggregateProblemSolver getAggregateSolverInstance();
/**
* @return The solver to be used to solve the individual problem in each period
*/
public IndividualProblemSolver getIndividualSolverInstance();
/**
* Calculates the expected aggregates from the aggregate transition on the provided state class, this
* should be used whenever the aggregate transition rules have been set
*
* @param state_ The current state of the calculation
*/
public void adjustExpectedAggregates(S state_);
/**
* @return The position of the first aggregate exogenous state dimension in a individual grid
*/
int getAggStochStateStart();
/**
* @return The position of the first aggregate endogenous state dimension in a individual grid
*/
int getAggDetStateStart();;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy