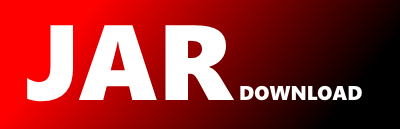
com.meliorbis.economics.model.ModelAndConfigValueDelegate Maven / Gradle / Ivy
/**
*
*/
package com.meliorbis.economics.model;
import java.util.Arrays;
import org.apache.commons.lang.ArrayUtils;
import com.meliorbis.economics.infrastructure.Base;
import com.meliorbis.economics.infrastructure.simulation.DiscretisedDistribution;
import com.meliorbis.numerics.IntArrayFactories;
import com.meliorbis.numerics.fixedpoint.FixedPointValueDelegate;
import com.meliorbis.numerics.generic.IntegerArray;
import com.meliorbis.numerics.generic.primitives.DoubleArray;
import com.meliorbis.utils.Utils;
/**
* Gets the input values from the config, and allows them to be adjusted. The
* output values come from the model, taking the state into account. Both inputs
* and outputs comprise an array of aggregate endogenous states followed by
* aggregate controls.
*
* @author Tobias Grasl
*
* @param The config type
* @param The state type
* @param The model type
*/
public class ModelAndConfigValueDelegate, M extends Model> extends Base implements FixedPointValueDelegate>
{
private final C _config;
public ModelAndConfigValueDelegate(C config_)
{
_config = config_;
}
public C getConfig()
{
return _config;
}
@Override
public void setInputs(double[] values_)
{
for (int i = 0; i < values_.length; i++)
{
setInput(i, values_[i]);
}
}
@SuppressWarnings({ "rawtypes", "unchecked" })
@Override
public double[] getOutputs(AggregateFixedPointState state_)
{
DiscretisedDistribution distribution = state_.getSimState();
S calcState = state_.getCalcState();
final M model = state_.getModel();
try
{
// Construct a zero-shock array of appropriate length
IntegerArray> zeroShocks = IntArrayFactories.createIntArrayOfSize(model.getConfig().getAggregateExogenousStateCount());
// Calculate the aggregates implied by the provided distribution
double[] impliedAggStates = model.calculateAggregateStates(distribution, zeroShocks,calcState);
// Get the transition function
DoubleArray> fullTrans = calcState.getIndividualPolicyForSimulation();
// Need to 'hide' the aggregate dimensions, will should all be of size 1
int[] selectionArray = Utils.repeatArray(0, fullTrans.numberOfDimensions());
Arrays.fill(selectionArray, 0,model.getConfig().getIndividualEndogenousStateCount()+model.getConfig().getIndividualExogenousStateCount(),-1);
int nAggControls = model.getConfig().getAggregateControlCount();
Arrays.fill(selectionArray, model.getConfig().getIndividualEndogenousStateCount()+model.getConfig().getIndividualExogenousStateCount()+model.getConfig().getAggregateEndogenousStateCount(),
model.getConfig().getIndividualEndogenousStateCount()+model.getConfig().getIndividualExogenousStateCount()+model.getConfig().getAggregateEndogenousStateCount()+ nAggControls,-1);
if( nAggControls > 0 )
{
// Determine the implied controls
return ArrayUtils.addAll(impliedAggStates, ((ModelWithControls)model).calculateAggregateControls(distribution, fullTrans.at(selectionArray), impliedAggStates, zeroShocks,(StateWithControls>) calcState));
}
else
{
return impliedAggStates;
}
} catch (ModelException e)
{
throw new RuntimeException("Unable to determine implied values", e);
}
}
@Override
public double[] getInitialInputs()
{
// Just get the configured values for aggregate states and controls, and return
final int nStates = _config.getAggregateEndogenousStates().size();
double[] initial = new double[nStates + _config.getAggregateControls().size()];
for(int i = 0; i < initial.length; i++)
{
if(i < nStates)
{
initial[i] = _config.getAggregateEndogenousStates().get(i).get(0);
}
else
{
initial[i] = _config.getAggregateControls().get(i - nStates).get(0);
}
}
return initial;
}
private void setInput(int index_, double newValue_)
{
DoubleArray> varToAdjust;
int stateCount = _config.getAggregateEndogenousStates().size();
if(index_ >= stateCount)
{
varToAdjust = _config.getAggregateControls().get(index_ - stateCount);
}
else
{
varToAdjust = _config.getAggregateEndogenousStates().get(index_);
}
varToAdjust.set(newValue_, 0);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy